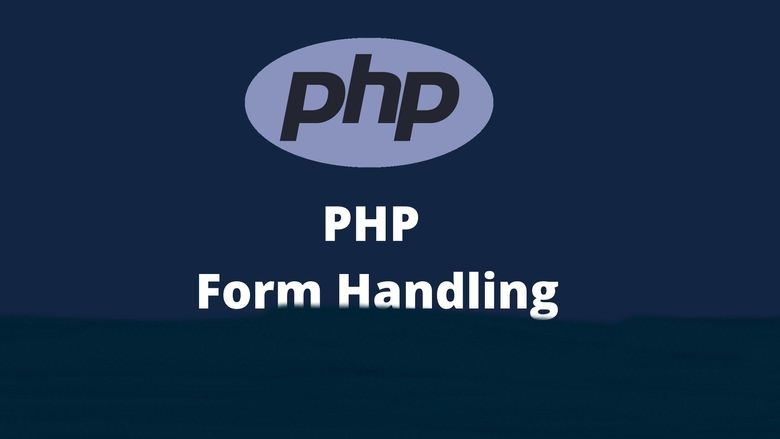
PHP Form Handling is an essential aspect of web development that allows you to collect and process data submitted through web forms. Whether you are building a simple contact form or a complex e-commerce checkout, understanding PHP Form Handling is crucial. It involves creating HTML forms, submitting data to a PHP script, validating and sanitizing user input, and processing it as per the requirements. This comprehensive guide covers all the essential aspects of PHP Form Handling, including best practices and tips for secure and optimized coding.
Introduction to PHP Form Handling
What is PHP Form Handling?
PHP form handling refers to the process of collecting data submitted by web users via HTML forms and processing it in a PHP script. The PHP script can then take specific actions with the user-submitted data, such as storing it in a database or sending it in an email.
Importance of PHP Form Handling
Forms are fundamental elements of web applications that allow users to submit data to a server for processing. PHP form handling is crucial because it provides a secure and efficient way to collect user data and validate it. Without proper form handling, an application’s security and functionality can be compromised.
Understanding HTML Forms and PHP POST Method
Creating HTML Forms
HTML forms can collect various types of data such as text, numbers, and files. To create an HTML form, you need to use thetag and provide the action attribute that specifies the target PHP script where the form data will be submitted.
HTTP POST Method and Its Advantages
The HTTP POST method is the most used method for submitting form data because it sends the data in the HTTP request body, protecting it from being visible in the URL. The POST method also allows for a larger amount of data to be sent compared to the GET method.
How PHP Handles Form Data
PHP handles form data through superglobal arrays such as $_POST and $_GET, which contain the user-submitted data from the HTML form. You can access this data using PHP functions and process it accordingly.
Validating Form Data with PHP
Why Form Data Validation is Important?
Form data validation is crucial because it ensures that the data submitted by the user is in the correct format and meets specific requirements. This prevents errors and improves the user experience.
PHP Functions for Form Validation
PHP provides various built-in functions for data validation, such as filter_var(), preg_match(), and ctype_digit(). These functions allow you to validate and sanitize user data in different ways.
Custom Validation Rules
In addition to built-in validation functions, you can also create custom validation functions tailored to your application’s specific needs. Custom validation rules can ensure that data meets specific standards unique to your application.
Displaying Error Messages and Feedback to Users
Displaying Error Messages
When form data fails validation, it’s essential to provide clear and concise error messages to help the user understand what went wrong. To display these messages, you can use PHP’s error handling functions and display them on the form page.
Highlighting Error Fields
Another best practice in form handling is to highlight the fields that contain errors visually. This helps users quickly identify which fields need correcting and improves the user experience.
Providing Feedback to Users
After users submit their data, it’s essential to provide feedback to let them know their submission has been successful. This can be achieved by displaying a thank you message or redirecting them to a confirmation page. Proper feedback improves user satisfaction and trust in your application.
Sanitizing Data with PHP Functions
What is Data Sanitization?
Data sanitization is the process of removing or replacing any potentially harmful or invalid characters from user input to ensure data security and integrity. It helps prevent various types of attacks such as SQL injection and cross-site scripting (XSS).
PHP Functions for Data Sanitization
PHP offers a variety of functions for data sanitization, including strip_tags(), htmlspecialchars(), and addslashes(). Strip_tags() removes HTML and PHP tags from user input, while htmlspecialchars() converts special characters to their HTML entity equivalents. addslashes() adds a backslash before special characters to prevent SQL injection.
Best Practices for Data Sanitization
The best way to prevent attacks is by using a combination of data validation and sanitization. Always sanitize user input before processing it and use filters to validate the data type. Avoid using blacklists and instead allow only specific valid characters. It’s also good practice to sanitize user input before storing it in a database.
Sending Emails from PHP Form
Setting Up Email Configuration
To send emails from a PHP form, you need to configure the email settings in PHP. You can use the mail() function to send emails, but it requires SMTP authentication. Set the SMTP server, user name, and password in the PHP script to enable email sending.
PHP Functions for Sending Emails
PHP provides built-in functions for sending emails, such as mail() and PHPMailer. Mail() is a basic function that sends emails using SMTP authentication. PHPMailer is a third-party library that provides more advanced email options, such as attachments, HTML emails, and SMTP authentication.
Handling Email Attachments
To add attachments to an email, use the PHPMailer library or the built-in PHP function called mime_content_type(). Use the move_uploaded_file() function to move uploaded files to the server and refer to the file’s path for attaching it to an email.
Handling File Uploads with PHP
HTML Form for File Uploads
To enable file uploads, set the enctype attribute of the form tag to “multipart/form-data”. Use the input tag with type “file” to allow the user to select a file for upload.
PHP Functions for File Uploads
PHP provides several built-in functions for handling file uploads, such as is_uploaded_file() and move_uploaded_file(). is_uploaded_file() returns true if the specified file is uploaded via HTTP POST, while move_uploaded_file() moves the uploaded file to a new location on the server.
Validating and Sanitizing Uploaded Files
Never trust user input for file validation. Always validate the file type and size on the server-side. Use the finfo_file() function or the mime_content_type() function to validate the file type. Sanitize the file name by removing unwanted characters and spaces.
Best Practices and Tips for PHP Form Handling
Secure Coding Practices
Filter and sanitize all user input, use encryption for sensitive data, and validate input to prevent any malicious code injection. Use prepared statements to prevent SQL injection.
Performance Optimization Techniques
Optimize the code by reducing the number of database queries. Use caching techniques to speed up page loading. Use gzip compression to reduce the size of files transferred from the server to the client.
Common Pitfalls and How to Avoid Them
Avoid using deprecated PHP functions and libraries. Keep the code up to date with the latest security patches and updates. Never store sensitive data in session variables or cookies. Use HTTPS to ensure a secure connection between the server and the client.In conclusion, PHP Form Handling is an indispensable part of web development, allowing you to create dynamic and interactive web applications. By following the best practices and techniques discussed in this guide, you can ensure that your PHP forms are secure, reliable, and efficient. Whether you are a seasoned PHP developer or just starting, this guide provides you with all the essential knowledge and skills to handle form data effectively. So, go ahead and implement these strategies in your next project, and take your PHP Form Handling skills to the next level.
FAQs
What is the difference between GET and POST methods in PHP Form Handling?
The GET method is used to retrieve data from the server, while the POST method is used to submit data to the server. In PHP Form Handling, the POST method is preferred for submitting form data as it is more secure and can handle larger data sets.
Why is data validation important in PHP Form Handling?
Data validation is crucial in PHP Form Handling to ensure that the data submitted through a form is accurate, complete, and conforms to the expected format. Proper validation prevents the submission of invalid data that can cause errors or vulnerabilities in the application.
Can PHP Form Handling be used for file uploads?
Yes, PHP Form Handling can be used for file uploads by creating an HTML form with a file input field and configuring the PHP script to handle file uploads. The uploaded files can then be validated, sanitized, and processed as required.
What are the best practices for PHP Form Handling?
Some of the best practices for PHP Form Handling include using secure coding practices, validating user input, sanitizing data, handling errors and feedback, optimizing performance, and avoiding common pitfalls such as SQL injection and cross-site scripting (XSS) attacks.