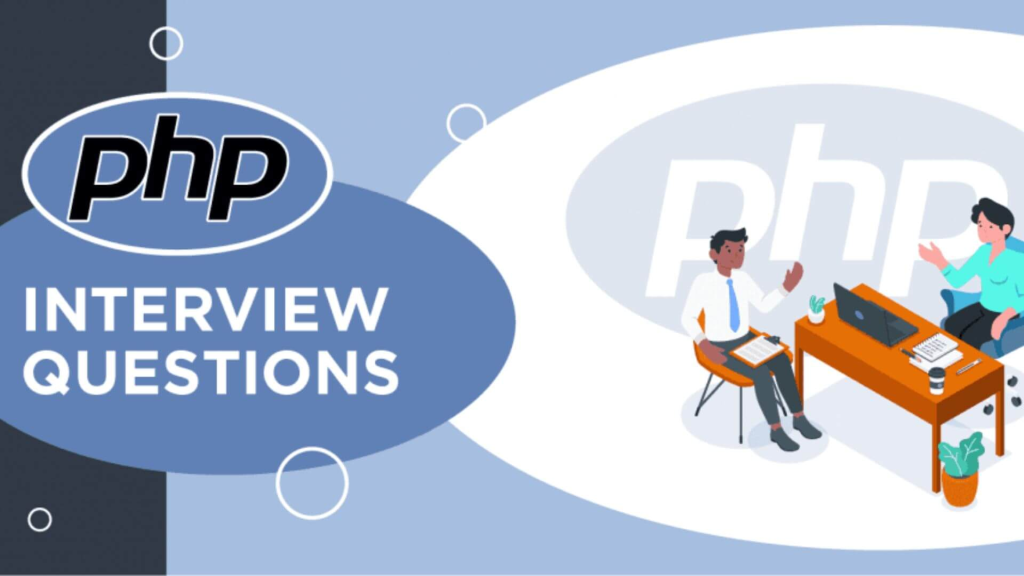
1. List some of the features of PHP8.
Features of PHP8:
- Just-In-Time (JIT) compilation for improved performance.
- Union Types to enable specifying multiple data types for function arguments, return types, and variables.
- Named Arguments to allow passing function arguments in any order by specifying the parameter names.
- Match Expressions for a more concise and powerful alternative to switch statements.
- Attributes for adding metadata to classes, functions, and other code elements.
- Constructor Property Promotion to simplify the process of declaring and initializing class properties.
2. What is “echo” in PHP?
echo is a language construct in PHP that outputs one or more strings. It does not require parentheses or semicolons.
3. What is “print” in PHP?
print is another language construct in PHP that outputs a string. Unlike echo, it requires parentheses and returns 1, so it can be used in expressions.
4. What is the difference between “echo” and “print” in PHP?
The main difference is that print returns 1, so it can be used in expressions, while echo doesn’t return anything. Also, print requires parentheses, whereas echo does not.
5. How a variable is declared in PHP?
A variable in PHP is declared using the $ symbol followed by the variable name. For example:
$name = "maruti";
6. What is the difference between $message and $$message?
$message refers to a variable called message, while $$message refers to a variable whose name is stored in the variable $message.
7. What are the ways to define a constant in PHP?
Constants can be defined using the define() function or the const keyword. For example:
define("CONSTANT", "Hello world!");
or
const CONSTANT = "Hello world!";
8. What are magic constants in PHP?
Magic constants are predefined constants in PHP that change based on their usage. Some examples include:
- _LINE_: Returns the current line number.
- _FILE_: Returns the full path and filename of the file.
- _DIR_: Returns the directory of the file.
- _FUNCTION_: Returns the function name.
- _CLASS_: Returns the class name.
- _METHOD_: Returns the class method name.
9. How many data types are there in PHP?
PHP has eight primitive data types:
- four scalar types:- boolean, integer, float (floating-point number), string
- two compound types:- array, object
- two special types:- resource, NULL
10. How to do single and multi line comment in PHP?
Single and multi-line comment in PHP:
- Single-line comment: // is used for single-line comments.
- Multi-line comment: /* */ encloses multi-line comments. For example:
// Single-line comment
/*
Multi-line
comment
*/
11. What are the different loops in PHP?
In PHP, there are several types of loops including:
- for loop: A for loop repeats until a specified condition evaluates to FALSE.
Example:
// Print numbers from 1 to 5 using a for loop
for ($i = 1; $i <= 5; $i++) {
echo $i . " ";
}
// Output: 1 2 3 4 5
2. while loop: A while loop executes a block of code as long as the test expression is TRUE.
Example:
// Print numbers from 1 to 5 using a while loop
$num = 1;
while ($num <= 5) {
echo $num . " ";
$num++;
}
// Output: 1 2 3 4 5
3. do while loop: A do while loop is similar to a while loop except the condition is tested after the statement(s) have been executed.
Example:
// Print numbers from 1 to 5 using a do-while loop
$num = 1;
do {
echo $num . " ";
$num++;
} while ($num <= 5);
// Output: 1 2 3 4 5
4. foreach loop: A foreach loop iterates over arrays, objects and strings.
Example:
// Iterate through an array using foreach loop
$colors = array("Red", "Green", "Blue", "Yellow");
foreach ($colors as $color) {
echo $color . " ";
}
// Output: Red Green Blue Yellow
12. What is the use of count() function in PHP?
The count() function in PHP is used to count the number of elements in an array or the number of properties in an object.
13. What is the use of header() function in PHP?
The header() function in PHP is used to send raw HTTP headers to the browser. It is commonly used for tasks like redirecting the user to a different page, setting cookies, or specifying the content type of the response.
14. What does isset() function?
The isset() function in PHP is used to determine whether a variable is set and not null. It returns true if the variable exists and has a value other than null, and false otherwise.
15. Explain PHP parameterized functions.
PHP parameterized functions, also known as user-defined functions, allow you to define your own functions and pass values (parameters) to them. These values can be used within the function to perform specific tasks. Parameterized functions enhance code reusability and modularity.
16. Explain PHP variable length argument function
PHP variable-length argument functions, also known as variadic functions, allow you to pass a varying number of arguments to a function. The number of arguments can be dynamic, and you can process them within the function using the func_get_args() or … operator.
17. What is the array in PHP?
An array in PHP is a special variable that can hold multiple values under a single name. These values can be accessed by referring to an index number or name 1. An array can store different types of data, such as integers, strings, and even other arrays. Each value in an array is identified by a unique key or index.
18. How many types of array are there in PHP?
There are three types of arrays in PHP: indexed arrays, associative arrays, and multidimensional arrays.
- Indexed arrays: These arrays use numerical indices to store values. The first element has an index of 0, the second has an index of 1, and so on.
- Associative arrays: These arrays use named keys to store values. Each key is associated with a specific value, allowing easy retrieval of values based on their keys.
- Multidimensional arrays: These arrays can store arrays within arrays, forming a multidimensional structure. They allow the organization of data in rows and columns, similar to a table.
19. Explain some of the PHP array functions?
Here some Array Functions is:
- array(): Creates an array.
- count(): Counts the number of elements in an array.
- sort(): Sorts an array in ascending order.
- rsort(): Sorts an array in descending order.
- array_push(): Adds one or more elements to the end of an array.
- array_pop(): Removes the last element from an array.
- array_key_exists(): Checks if a specified key exists in an array.
- array_search(): Searches for a specific value in an array and returns its key.
20. What is the difference between indexed and associative array?
Indexed Arrays:
Indexed arrays, also known as numeric arrays, are arrays that use numeric indices to access and store values. In these arrays, each element is assigned a unique index starting from 0 and incrementing by 1 for each subsequent element. The indices determine the order of the elements in the array, and they are used to directly access the values stored in the array.
For example, consider the following indexed array in PHP:
$fruits = array("Apple", "Banana", "Orange");
In this array, “Apple” is stored at index 0, “Banana” is stored at index 1, and “Orange” is stored at index 2.
Associative Arrays:
Associative arrays, also known as key-value pairs or dictionaries, are arrays where each element is associated with a unique key instead of a numeric index. In these arrays, the keys can be of any data type (e.g., strings, integers) and are used to access and store values in the array.
For example, consider the following associative array in PHP:
$person = array(
"name" => "John Doe",
"age" => 30,
"city" => "New York"
);
In this array, “name”, “age”, and “city” are the keys associated with their respective values. The keys allow you to access the specific values in the array.
The main difference between indexed and associative arrays is the way elements are accessed and stored. In indexed arrays, elements are accessed using their numeric indices, while in associative arrays, elements are accessed using their associated keys.