PHP Iterative Statements, also known as loops, are used to execute a block of code repeatedly as long as a specified condition is true. PHP supports four types of iterative statements:
- while Loop
- do-while Loop
- for Loop
- foreach loop
while Loop:
The while loop is used when you want to execute a block of code as long as a specified condition is true. The show is given follow chat
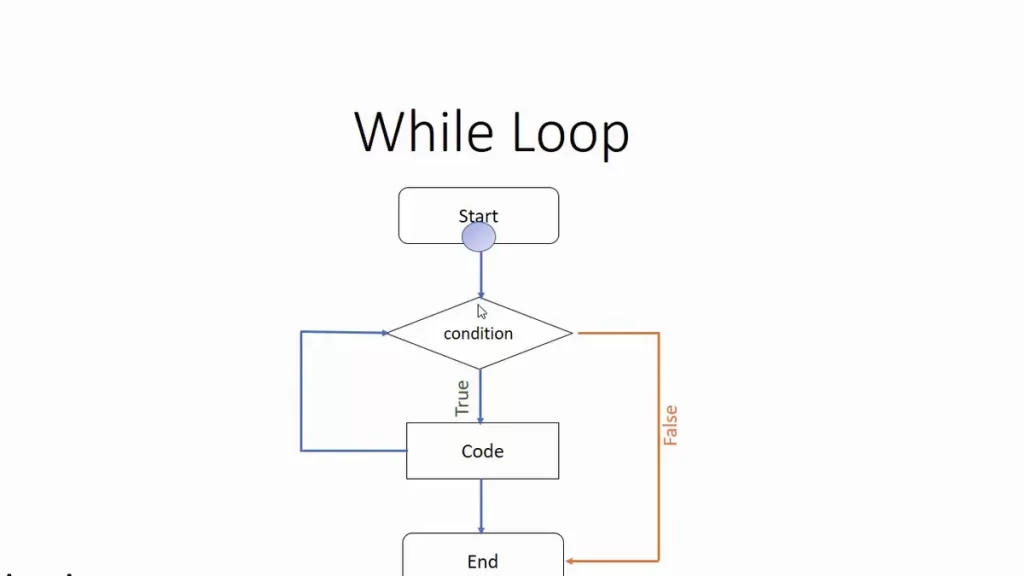
Syntax:
while (condition)
{
// code to be executed
}
Example:
<?php
$x = 1;
while($x <= 10) {
echo "The number is: $x <br>";
$x++;
}
?>
do-while Loop:
The do-while loop is similar to the while loop, but the block of code is executed at least once, regardless of whether the condition is true or false.
Syntax:
do {
// code to be executed
} while (condition);
Example:
<?php
$x =10;
do {
echo "The number is: $x <br>";
$x++;
} while ($x <= 9);
?>
for Loop:
The for loop is used when you know exactly how many times you want to execute a block of code. It has a counter variable that is incremented or decremented with each iteration.
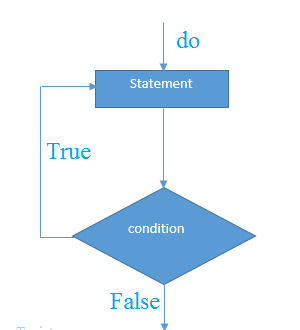
Syntax:
for (initialization; condition; increment/decrement)
{
// code to be executed
}
Example:
<?php
for ($x = 0; $x <= 10; $x++) {
echo "The number is: $x <br>";
}
?>
For-each loop:
The foreach loop is used to loop through arrays and objects. It iterates over each element in the array or each property in the object.
Syntax:
foreach ($array as $value)
{
// code to be executed
}
Example:
<?php
echo "Welcome to the world of foreach loops <br>";
$arr = array("Bananas", "Apples", "Harpic", "Bread", "Butter");
foreach ($arr as $value) {
echo "$value <br>";
}
?>