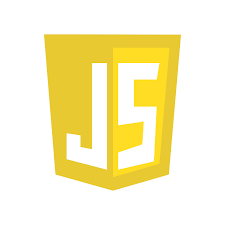
JavaScript is a programming language commonly used in web development to add interactive features and dynamic content to websites. It was first introduced in 1995 and has since become one of the most widely used programming languages on the web. In this article, we will explore the various use cases, features, and workflows of JavaScript.
Top Use Cases of JavaScript
JavaScript is commonly used for client-side scripting, which means it is executed on the user’s web browser rather than on the web server. Some of the top use cases of JavaScript include:
- Form validation
- Creating interactive UI elements such as dropdown menus and sliders
- Animating web pages
- Loading new content without refreshing the page (also known as AJAX)
- Creating browser-based games
- Creating chatbots
- Implementing real-time updates on a page
- Implementing user authentication and authorization
- Creating interactive maps and charts
- Building web-based calculators
Features of JavaScript
JavaScript is a dynamic language that supports a variety of features, including:
- Object-oriented programming
- Functional programming
- Prototypal inheritance
- Asynchronous programming using callbacks, promises, and async/await
- Manipulating the DOM (Document Object Model)
- Event-driven programming
- Regular expressions
Workflow of JavaScript
The workflow of JavaScript typically involves the following steps:
- Writing the code in a text editor or an integrated development environment (IDE)
- Saving the code with a .js file extension
- Linking the .js file to an HTML document using a script tag
- Running the code in a web browser
How JavaScript Works & Architecture
JavaScript has a single-threaded event loop architecture, which means that it can only execute one task at a time. However, it can delegate tasks to other threads using Web Workers. JavaScript is also a loosely typed language, which means that variables can be assigned different data types at runtime.
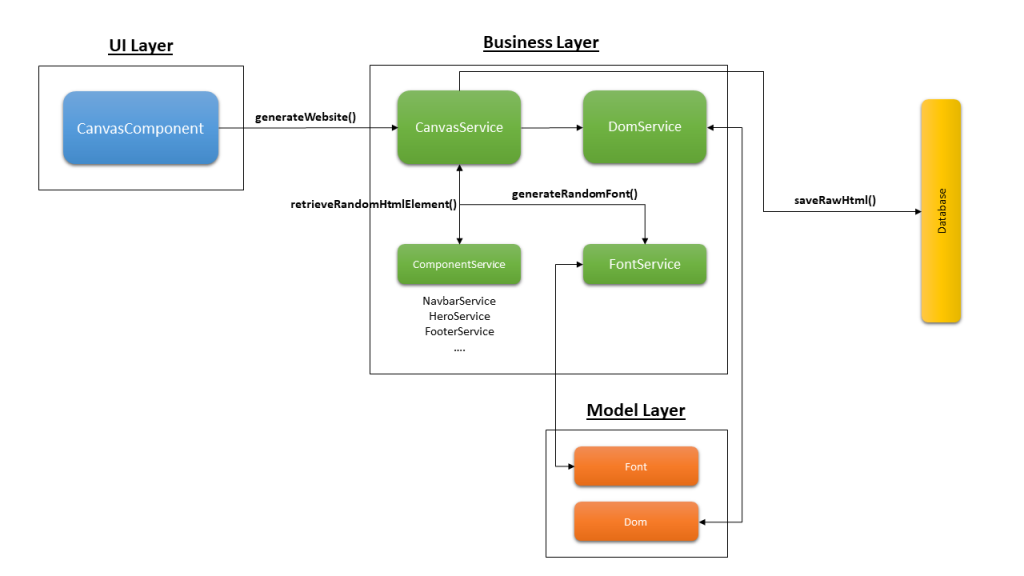
How to Install and Configure JavaScript
JavaScript is a client-side programming language, which means that it is executed on the user’s web browser rather than on the web server. Therefore, there is no need to install or configure JavaScript on a web server. However, developers can install and configure various JavaScript frameworks and libraries for their development environment.
Fundamental Tutorials of JavaScript: Getting started Step by Step
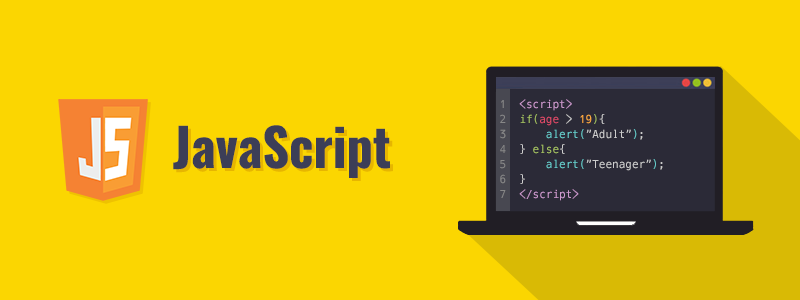
What is JavaScript?
JavaScript is a programming language used to create interactive websites. It is the most commonly used language for front-end web development. With JavaScript, you can add animations, create forms, and much more.
JavaScript is a versatile language that can be used for both front-end and back-end development. It can be run on any browser, making it a popular choice for web development.
Setting Up Your Environment
Before we start coding, we need to set up our environment. To write JavaScript code, we need a text editor and a web browser.
- Choose a text editor: There are many text editors available, such as Notepad++, Visual Studio Code, Sublime Text, and Atom. Choose the one that suits your needs.
- Open a web browser: You can use any web browser, such as Google Chrome, Mozilla Firefox, Safari, or Microsoft Edge.
Writing Your First Program
Now that we have our environment set up, let’s write our first program. The “Hello, World!” program is a simple program that prints the text “Hello, World!” on the screen.
- Open your text editor and create a new file.
- Type the following code:
console.log("Hello, World!");
- Save the file with the name “hello_world.js”.
- Open your web browser and create a new HTML file.
- Type the following code:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<script src="hello_world.js"></script>
</body>
</html>
- Save the file with the name “index.html”.
- Open the “index.html” file in your web browser. You should see the text “Hello, World!” printed on the screen.
Congratulations! You have written your first JavaScript program.
Understanding the Code
Let’s take a closer look at the code we just wrote.
console.log("Hello, World!");
This line of code uses the console.log()
method to print the text “Hello, World!” on the console. The console is a tool used for debugging and testing JavaScript code.
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<script src="hello_world.js"></script>
</body>
</html>
This HTML code creates a basic HTML document and includes the JavaScript file we just created using the script
tag. The src
attribute is used to specify the location of the JavaScript file.
More Examples
Now that you have written your first JavaScript program, it’s time to practice some more examples. Here are a few examples to get you started:
Example 1: Print the Sum of Two Numbers
let num1 = 10;
let num2 = 20;
let sum = num1 + num2;
console.log(sum);
This code declares two variables num1
and num2
and initializes them with the values 10 and 20, respectively. It then calculates the sum of the two numbers and stores it in a variable called sum
. Finally, it prints the value of sum
to the console.
Example 2: Create a Function to Calculate the Area of a Circle
function calculateArea(radius) {
let area = Math.PI * radius * radius;
return area;
}
let circleRadius = 5;
let circleArea = calculateArea(circleRadius);
console.log(circleArea);
This code defines a function called calculateArea
that takes a parameter radius
. It calculates the area of a circle using the formula Math.PI * radius * radius
and returns the result. It then declares a variable circleRadius
and initializes it with the value 5. It calls the calculateArea
function with circleRadius
as an argument and stores the result in a variable called circleArea
. Finally, it prints the value of circleArea
to the console.
Example 3: Create an Object to Represent a Person
let person = {
firstName: "John",
lastName: "Doe",
age: 35,
occupation: "Web Developer"
};
console.log(person.firstName + " " + person.lastName);
console.log(person.age);
console.log(person.occupation);
This code creates an object person
that represents a person. It has four properties: firstName
, lastName
, age
, and occupation
. It then prints the person’s full name, age, and occupation to the console.
Example 4: Reverse a String
function reverseString(str) {
let reversedStr = "";
for (let i = str.length - 1; i >= 0; i--) {
reversedStr += str[i];
}
return reversedStr;
}
let str = "hello world";
let reversedStr = reverseString(str);
console.log(reversedStr);
This code defines a function called reverseString
that takes a parameter str
. It initializes a variable called reversedStr
to an empty string. It then loops through the characters of the string in reverse order and appends each character to reversedStr
. Finally, it returns the reversed string. It then declares a variable str
and initializes it with the value “hello world”. It calls the reverseString
function with str
as an argument and stores the result in a variable called reversedStr
. Finally, it prints the value of reversedStr
to the console.
Conclusion
In this article, we have learned the basics of JavaScript and how to write your first program – the “Hello, World!” program. We have also looked at how to set up your environment and run the program in a web browser. Additionally, we have provided some examples for you to practice and improve your JavaScript skills. With practice and dedication, you can become a skilled JavaScript developer. Happy coding!
[…] What is JavaScript and How JavaScript Works & Architecture […]