What is JavaScript Objects ?
JavaScript object is an real world entity having some state and behavior (properties and method) is known as JavaScript. For example: car, pen, bike, chair, glass, keyboard, monitor etc.
JavaScript is an object-based language. Everything is an object in JavaScript. It is template based not class based. Here, we don’t create class to get the object. But, we direct create objects.
Creating Objects in JavaScript:
In JavaScript, There are 3 ways to create objects.
- By object literal
- By creating instance of Object directly (using new keyword)
- By using an object constructor (using new keyword)
- Creating object using object literal:
Syntax:
object={property1:value1,property2:value2.....propertyN:valueN}
The given syntax, property and value is separated by : (colon).
Example:
<html>
<body>
<script>
emp={id:101,name:"Abhishek Kumar",salary:50000}
document.write(emp.id+" "+emp.name+" "+emp.salary);
</script>
</body>
</html>
Output:

2) By creating instance of Object:
Syntax:
var objectname=new Object();
Example:
<html>
<body>
<script>
var emp=new Object();
emp.id=101;
emp.name="Rahul Kumar";
emp.salary=30000;
document.write(emp.id+" "+emp.name+" "+emp.salary);
</script>
</body>
</html>
Output:
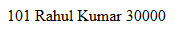
3) By using an Object constructor:
Object constructor need to create function with arguments. Each argument value can be assigned in the current object by using this keyword. The this keyword refers to the current object.
Example:
<html>
<body>
<script>
function emp(id,name,salary)
{
this.id=id;
this.name=name;
this.salary=salary;
}
e=new emp(1001,"Rohit Kumar",50000);
document.write(e.id+" "+e.name+" "+e.salary);
</script>
</body>
</html>
Output:

JavaScript Array:
In JavaScript, An array is a collection of data that are stored in a variable. The values in an array can be of any type, including numbers, strings, objects, and even other arrays. Arrays are useful for storing and manipulating sets of data, such as a list of names or a series of numbers.
To create an array in JavaScript, you can use square brackets []
and separate the values with commas( , ).
There are 3 ways to construct array in JavaScript
- By array literal
- By creating instance of Array directly (using new keyword)
- By using an Array constructor (using new keyword)
1) JavaScript array literal
Syntax:
var arrayname=[value1,value2.....valueN];
Example:
<html>
<body>
<script>
var emp=["Ram","Shyam","Mohan"];
for (i=0;i<emp.length;i++){
document.write(emp[i] + "<br/>");
}
</script>
</body>
</html>
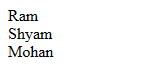
2) JavaScript Array directly (new keyword)
Syntax:
var arrayname=new Array();
Here, new keyword is used to create instance of array.
Example:
<html>
<body>
<script>
var i;
var emp = new Array();
emp[0] = "Rohit";
emp[1] = "Mohit";
emp[2] = "Urfi";
for (i=0;i<emp.length;i++)
{
document.write(emp[i] + "<br>");
}
</script>
</body>
</html>
Output:
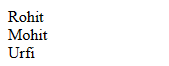
3) JavaScript array constructor (new keyword):
The example of creating object by array constructor is given below-
Example:
<html>
<body>
<script>
var emp=new Array("Rahul","Rohit","Smith");
for (i=0;i<emp.length;i++)
{
document.write(emp[i] + "<br>");
}
</script>
</body>
</html>
Output:
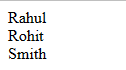
JavaScript Array Methods:
Arrays have many built-in methods for manipulating and iterating over the elements.
Some of the most commonly used array methods are:
- length – This method returns the number of elements in an array. For example, the following code will return 3:
var myArray = [1, "Hello", [2, 3]];
console.log(myArray.length);
- push – This method is used to add an element to the end of an array. For example, the following code will add the element “World” to the end of the array:
var myArray = [1, "Hello", [2, 3]];
myArray.push("World");
console.log(myArray); // [1, "Hello", [2, 3], "World"]
- pop – This method is used to remove the last element of an array. For example, the following code will remove the last element (“World”) from the array:
var myArray = [1, "Hello", [2, 3], "World"];
myArray.pop();
console.log(myArray); // [1, "Hello", [2, 3]]
- shift – This method is used to remove the first element of an array. For example, the following code will remove the first element (1) from the array:
var myArray = [1, "Hello", [2, 3]];
myArray.shift();
console.log(myArray); // ["Hello", [2, 3]]
- unshift – This method is used to add an element to the beginning of an array. For example, the following code will add the element 0 to the beginning of the array:
var myArray = [1, "Hello", [2, 3]];
myArray.unshift(0);
console.log(myArray); // [0, 1, "Hello", [2, 3]]
- slice – This method is used to extract a portion of an array. For example, the following code will extract the elements from index 1 to 2 (exclusive):
var myArray = [1, "Hello", [2, 3]];
console.log(myArray.slice(1, 2)); // ["Hello"]
- splice – This method is used to add or remove elements from an array. For example, the following code will remove the element at index 1 and add the elements “Hello World” and [4, 5] at index 1:
var myArray = [1, "Hello", [2, 3]];
myArray.splice(1, 1, "Hello World", [4, 5]);
console.
JavaScript String:
It is one of the most important concept of JavaScript, A string is a sequence of characters enclosed within single quotes or double quotes. For example, the following are valid strings in JavaScript:
let strName = 'Hello, world!';
let strName = "Hello, world!";
There are 2 ways to create string in JavaScript
- By string literal
- By string object (using new keyword)
- By string literal:
String literal is created using single quotes or double quotes.
Syntax:
let strName = 'Hello, world!';
let strName = "Hello, world!";
Example:
<!DOCTYPE html>
<html>
<body>
<script>
var str='This is the example of string literal';
var str1="This is the example of string literal";
document.write(str + "<br>");
document.write(str1);
</script>
</body>
</html>
Output:
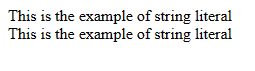
2. By string object (using new keyword):
new keyword is used to create instance of string.
Syntax:
var strName=new String("string literal");
Example:
<!DOCTYPE html>
<html>
<body>
<script>
var strName=new String("hello javascript string");
document.write(strName);
</script>
</body>
</html>
Output:
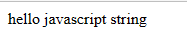
String Methods:
String have many in-built methods for manipulating the elements.
Some of the most commonly used string methods are:
- length() – This method returns the number of characters in a string. For example, the following code will return 11:
var str = "Hello World";
console.log(str.length);
- charAt(Index) – This method returns the character at the given index. For example, the following code will return c:
<!DOCTYPE html>
<html>
<body>
<script>
var str="Welcome";
document.write(str.charAt(3));
</script>
</body>
</html>
- concat(str) – This method concatenates or joins two strings. For example, the following code will return – Welcome To Cotocus
<!DOCTYPE html>
<html>
<body>
<script>
var s1="Welcome ";
var s2="To Cotocus";
var s3=s1.concat(s2);
document.write(s3); // Welcome To Cotocus
</script>
</body>
</html>
- indexOf (index) – This method is used to find the index of a specific character or substring in a string. For example, the following code will return 8:
<!DOCTYPE html>
<html>
<body>
<script>
var s1="Welcome to aiuniverse.xyz";
var n=s1.indexOf("to");
document.write(n);
</script>
</body>
</html>
- split() Method:
It splits a string into substring array, then returns that newly created array.
<!DOCTYPE html>
<html>
<body>
<script>
var str="Welcome to aiuniverse.xyz";
document.write(str.split(" ")); //splits the given string.
</script>
</body>
</html>
- trim() Method: This method removes leading and trailing whitespaces from the string.
<!DOCTYPE html>
<html>
<body>
<script>
var s1=" Maruti Cotocus ";
var s2=s1.trim();
document.write(s2); // remove before space and after space
</script>
</body>
</html>
- toUpperCase() Method: These methods are used to convert a string to uppercase letters.
<!DOCTYPE html>
<html>
<body>
<script>
var s1="JavaScript toUpperCase Example by Maruti";
var s2=s1.toUpperCase();
document.write(s2);
</script>
</body>
</html>
Output:

- toLowerCase() Method: These methods are used to convert a string to lowercase letters.
<!DOCTYPE html>
<html>
<body>
<script>
var s1="JavaScript toUpperCase Example by Maruti";
var s2=s1.toLowerCase();
document.write(s2);
</script>
</body>
</html>

- slice(beginIndex, endIndex) Method– This method is used to extract a portion of a string.
<!DOCTYPE html>
<html>
<body>
<script>
var s1="MarutiKumar";
var s2=s1.slice(3,6);
document.write(s2); //uti
</script>
</body>
</html>
- replace() – This method is used to replace a specific character or substring in a string. For example, the following code will return “Hello Universe”:
<!DOCTYPE html>
<html>
<body>
<script>
var str="Welcome Cotocus";
document.writeln(str.replace("Cotocus","Maruti"));
</script>
</body>
</html>
Output:
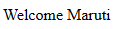
Date Object:
In JavaScript, Date object has a in-built object that can be used to work with dates and times.
You can use 4 variant of Date constructor to create date object.
- Date()
- Date(milliseconds)
- Date(dateString)
- Date(year, month, day, hours, minutes, seconds, milliseconds)
- Date(): Date object is used to prints current date and time both.
Example:
<html>
<body>
Current Date and Time: <span id="txt"></span>
<script>
var today=new Date();
document.getElementById('txt').innerHTML=today;
</script>
</body>
</html>
Another Example: Print date/month/year.
<html>
<body>
<script>
var date=new Date();
var day=date.getDate();
var month=date.getMonth()+1;
var year=date.getFullYear();
document.write("<br>Date is: "+day+"/"+month+"/"+year);
</script>
</script>
</body>
</html>
Current Time Example:
<html>
<body>
Current Time: <span id="txt"></span>
<script>
var today=new Date();
var h=today.getHours();
var m=today.getMinutes();
var s=today.getSeconds();
document.getElementById('txt').innerHTML=h+":"+m+":"+s;
</script>
</body>
</html>
JavaScript Math:
In JavaScript, Math is a built-in object in JavaScript that provides mathematical functions and constants for performing mathematical operations such as trigonometry, logarithms, etc. It provides a set of methods and properties that allow developers to perform complex mathematical calculations easily and efficiently.
most commonly used functions in the Math
object is-
- Math.random()
Math.random() method returns the random number between 0 (inclusive) and 1 (exclusive). This function can be used to generate random numbers for various purposes such as games, simulations, and other applications.
For Example:
<!DOCTYPE html>
<html>
<body>
Random Number is: <span id="p2"></span>
<script>
document.getElementById('p2').innerHTML=Math.random();
</script>
</body>
</html>
Output:

2. Math.pow(m,n)
Math.pow(m,n) method returns the m to the power of n that is mn.
For Example:
<!DOCTYPE html>
<html>
<body>
5 to the power of 3 is: <span id="p5"></span>
<script>
document.getElementById('p5').innerHTML=Math.pow(5,3);
</script>
</body>
</html>

3. Math.sqrt(n)
This method is used to returns the square root of the given number.
Example:
<!DOCTYPE html>
<html>
<body>
Square Root of 625 is: <span id="p1"></span>
<script>
document.getElementById('p1').innerHTML=Math.sqrt(625);
</script>
</body>
</html>
Output:

4. Math.floor(n)
This method is used to round a number down to the nearest integer. For example 9 for 9.7, 5 for 5.9 etc.
<!DOCTYPE html>
<html>
<body>
Floor of 4.6 is: <span id="p4"></span>
<script>
document.getElementById('p4').innerHTML=Math.floor(4.6);
</script>
</body>
</html>
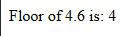
5. Math.ceil(n)
This method is used to rounds a number up to the nearest integer. For example 4 for 3.7, 6 for 5.9 etc.
<!DOCTYPE html>
<html>
<body>
Ceil of 7.6 is: <span id="p5"></span>
<script>
document.getElementById('p5').innerHTML=Math.ceil(7.6);
</script>
</body>
</html>
Output:
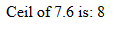
6. Math.abs(n)
This method returns the absolute value for the given number. For example 5 for -5.6 & 6 for -6.6 etc.
<!DOCTYPE html>
<html>
<body>
Absolute value of -4 is: <span id="p8"></span>
<script>
document.getElementById('p8').innerHTML=Math.abs(-4);
</script>
</body>
</html>
Example:

Number Object:
In JavaScript, a number object is a built-in object that provides a number of properties and methods for working with numeric values. The Number
object can be used to convert a value to a number, perform mathematical operations, and format numbers for display.
Most commonly used methods of the Number
object is–
- Number(): This method is used to convert a value to a number, This function can be used to convert strings, Booleans, and other types of values to numbers.
Example:
console.log(Number("3.14"));
// Output: 3.14
console.log(Number(true));
// Output: 1
- toFixed(): This method to format a number with a specific number of decimal places:
Example:
const numObj = new Number(10.12345);
console.log(numObj.toFixed(2)); // Output: "10.12"
- valueOf(): convert a number object to a primitive numeric value using the
valueOf()
method:
Example:
const numObj = new Number(10);
const num = numObj.valueOf();
console.log(num); // Output: 10
Boolean:
Boolean object is used to represents value in two states: true or false. The Boolean
object can be used to create Boolean values and perform logical operations, such as and, or, and not.
A Boolean value can be created by using the Boolean()
function or by assigning a value of true or false to a variable.
var isTrue = Boolean(1);
console.log(isTrue); // Output: true
var isFalse = Boolean(0);
console.log(isFalse); // Output: false