JavaScript Comment:
In JavaScript, comments are used to add information about the code, that are ignored by the browser or interpreter.
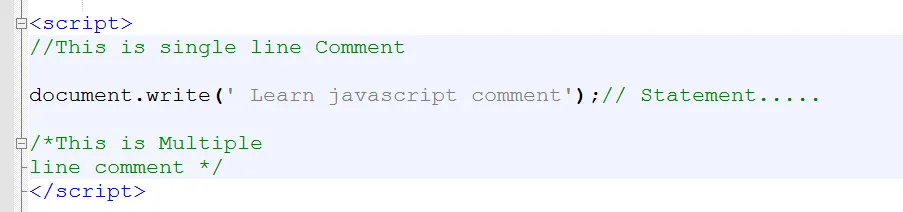
Advantage of JavaScript comments:
The main advantage of comments are-
- Easy to understand the code: It can be used to elaborate the code so that end user can easily understand the code.
- To avoid the unnecessary code: It can also be used to avoid the code being executed. Sometimes, we add the code to perform some action. But after sometime, there may be need to disable the code. In such case, it is better to use comments.
Types of JavaScript Comments
There are two types of comments in JavaScript.
- Single-line comments: They begin with two forward slashes (//) and continue until the end of the line.
Example1:
<html>
<body>
<script>
// It is single line comment
document.write("hello aiuniverse.xyz");
</script>
</body>
</html>
Output:
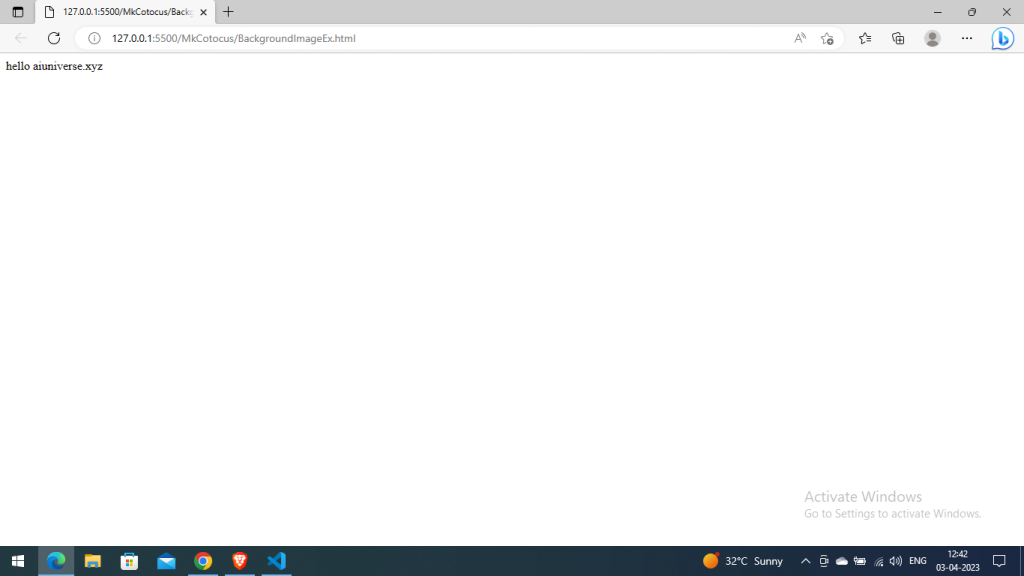
Example2:
<html>
<body>
<script>
var a=10;
var b=20;
var c=a+b;//It adds values of a and b variable
document.write(c);//prints sum of 10 and 20
</script>
</body>
</html>
Output:
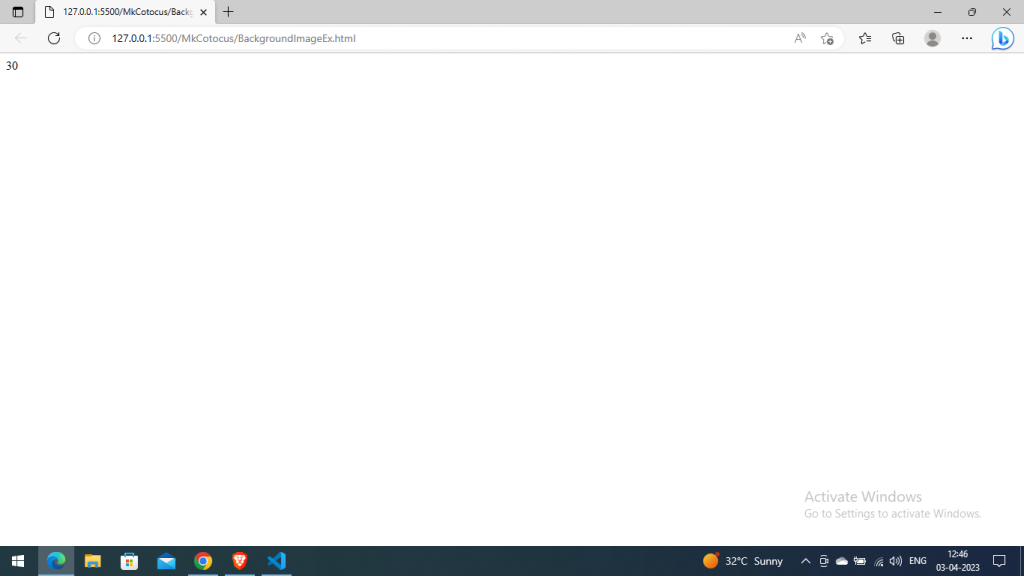
2. Multi-line comments: They begin with /* and end with */. Anything between these characters will be ignored by the browser or interpreter.
<html>
<body>
<script>
/* It is multi line comment.
It will not be displayed */
document.write("example of javascript multiline comment");
</script>
</body>
</html>
Output:
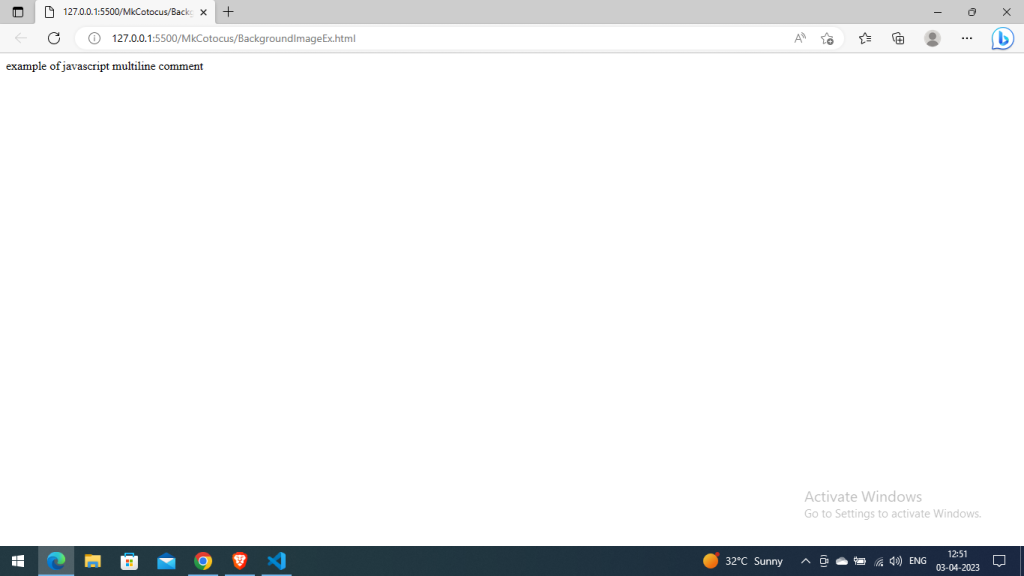
What is Variables ?
Variable is an essential part of any programming language, It is used to stored data. They allow you to store, retrieve, and manipulate data in your programs. There are two types of variables in JavaScript : Local variable , Global variable.
Some rules for declaring a JavaScript variable (also known as identifiers).
- Name must start with a letter (a to z or A to Z), underscore( _ ), or dollar( $ ) sign.
- After first letter we can use any digits (0 to 9), for example value1.
- JavaScript variables are case sensitive, for example x and X are different variables.
Variables are declared using the var
, let
, or const
keyword followed by the variable name.
For example:
// Declaring a variable using var
var message = "Hello World!";
// Declaring a variable using let
let age = 20;
// Declaring a variable using const
const PI = 3.14;
Where, “var” keyword followed by the name of the variable and “let” and “const” keywords to declare variables.
Once a variable is declared, its value can be assigned or updated by using the =
operator:
// Assigning a value to a variable
var x;
x = 20;
// Updating the value of a variable
var y = 6;
y = y + 1;
Variables can hold various types of values, including numbers, strings, Boolean values, arrays, objects, and more.
// Example of variables holding different types of values
var num = 12;
var greeting = "Hello";
var isTrue = true;
var myArray = [1, 2, 3];
var person = {name: "Rohit", age: 25};
JavaScript Local Variable:
A JavaScript local variable is declared inside block (function). It is accessible within the function or block only. For example:
<script>
function abc(){
var x=20; //local variable
}
</script>
Or
<script>
If(10<15){
var y=30; //JavaScript local variable
}
</script>
JavaScript global variable:
A variable i.e. declared outside the function or declared with window object is known as global variable. A JavaScript global variable is accessible from any function. For example:
<html>
<body>
<script>
var data=500; //gloabal variable
function a(){
document.writeln(data);
}
function b(){
document.writeln(data);
}
a();//calling JavaScript function
b();
</script>
</body>
</html>
Output:
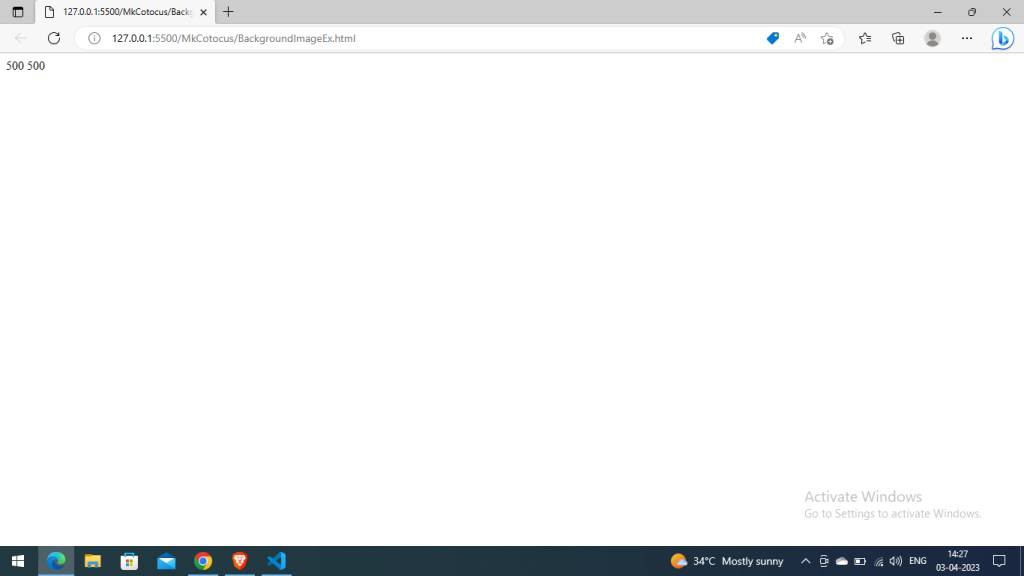
Data Types
JavaScript provides different data types to hold different types of values. There are two types of data types in JavaScript.
- Primitive data type
- Non-primitive (reference) data type
Primitive data type:
There are five types of primitive data types in JavaScript.
- Number: This data type is used to represent numeric values, including integers and floating-point numbers. (e.g. 10, 3.14)
- String: This data type is used to represent text values, enclosed in single or double quotes. (e.g. “hello”, ‘world’)
- Boolean: This data type is used to represent true or false values. (e.g. true, false)
- Undefined: This data type is used to represent a variable that has been declared but not assigned a value.
- Null: This data type has only one value, null, which represents the absence of any object value.
Non-Primitive data type:
The non-primitive data types are as follows:
- Object: This data type is used to represent complex data structures, including arrays, functions, and objects. (e.g. { name: “John”, age: 30 })
- Array: (e.g. [1, 2, 3])
JavaScript Operators and Expressions
What is Operators ?
Operators in JavaScript are symbols that perform specific operations on one or more operands (values or variables). For example, the addition operator (+) adds two operands together and the assignment operator (=) assigns a value to a variable.
There are different types of operators in JavaScript, including:
- Arithmetic Operators
- Comparison (Relational) Operators
- Bitwise Operators
- Logical Operators
- Assignment Operators
- Conditional (ternary) operator
- Arithmetic Operators: Used to perform arithmetic operations on numbers. Examples: + (addition), – (subtraction), * (multiplication), / (division), % (modulus), ++ (increment), and — (decrement).
- Comparison Operators: It is used to compare two values or variables. Examples: == (equal to), != (not equal to), === (strictly equal to), !== (strictly not equal to), > (greater than), < (less than), >= (greater than or equal to), and <= (less than or equal to).
- Bitwise Operators: Used to perform bitwise operations on numbers. Examples: & (bitwise and), | (bitwise or), ^ (bitwise exclusive or), ~ (bitwise not), << (left shift), and >> (right shift).
- Logical Operators: It is used to combine two or more conditions. Examples: && (logical and), || (logical or), and ! (logical not).
- Assignment Operators: It is used to assign values to variables. Examples: = (assignment), += (add and assign), -= (subtract and assign), *= (multiply and assign), /= (divide and assign), and %= (modulus and assign).
- Conditional (Ternary) Operator: It is used to assign a value to a variable based on a condition. Example: variable = (condition) ? value1 : value2;
What is Expressions ?
Expressions are combinations of values, variables, and operators that produce a result. For example:
2 + 2 // produces the value 4
"Hello, " + "world!" // produces the value "Hello, world!"
true && false // produces the value false
Math.Pow(2, 3) // produces the value 8
JS If-Else Statement:
The if-else statement is used to execute the code whether condition is true or false. There are three forms of if statement in JavaScript.
- If Statement
- If else statement
- If else if statement
- If Statement:
The “if” statement in JavaScript is used to execute a block of code if a certain condition is true.
Syntax:
if(expression)
{
//code to be executed if the condition is true
}
}
2. If else statement:
The “if” statement in JavaScript is used to execute a block of code if a certain condition is true. The “else” clause is used to execute a block of code if the condition is false.
Syntax:
if (condition)
{
// code will be executed if the condition is true
}
else
{
// code will be executed if the condition is false
}
Example:
let number = 5;
if (number % 2 == 0)
{
console.log("The number is even.");
} else {
console.log("The number is odd.");
}
3. If else if statement: (If else ladder):
if(expression1)
{
//content will be evaluated if expression1 is true
}
else if(expression2)
{
//content will be evaluated if expression2 is true
}
else if(expression3)
{
//content will be evaluated if expression3 is true
}
else
{
//content will be evaluated if no expression is true
}
Example:
<html>
<body>
<script>
var a=30;
if(a==20)
{
document.write("a is equal to 20");
}
else if(a==25)
{
document.write("a is equal to 25");
}
else if(a==20)
{
document.write("a is equal to 30");
}
else
{
document.write("a is not equal to 20, 25 or 30");
}
</script>
</body>
</html>
Output:
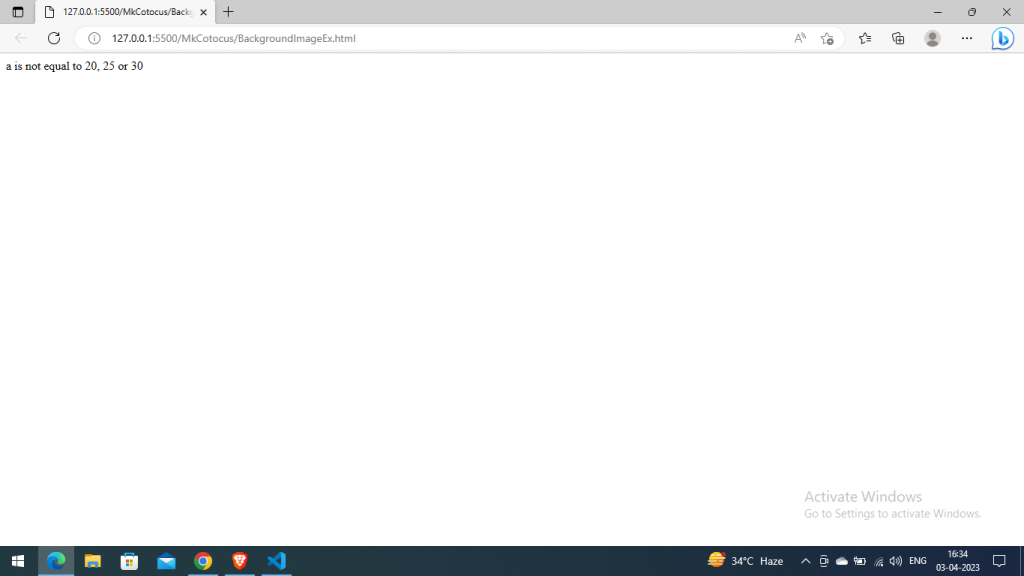
Switch case:
The “switch” statement in JavaScript is another control structure, It is used to execute one code from multiple expressions. It is just like “if-else ladder” statement when you have multiple conditions to check against a single value.
Syntax:
switch (expression)
{
case value1:
// code will be executed if expression == value1
break;
case value2:
// code will be executed if expression == value2
break;
…
default:
// code will be executed if expression does not match any of the values
}
JavaScript Loops:
In JavaScript, Loop statements to execute a block of code repeatedly. There are several type of loop statements:-
- for loop: The for loop repeats a block of code a specified number of times. It has three parts: initialization, condition, and increment/decrement.
Syntax:
for (initialization; condition; increment/decrement)
{
// code will be executed
}
Example:
<!DOCTYPE html>
<html>
<body>
<script>
for (i=1; i<=10; i++)
{
document.write(i + "<br/>")
}
</script>
</body>
</html>
Output:
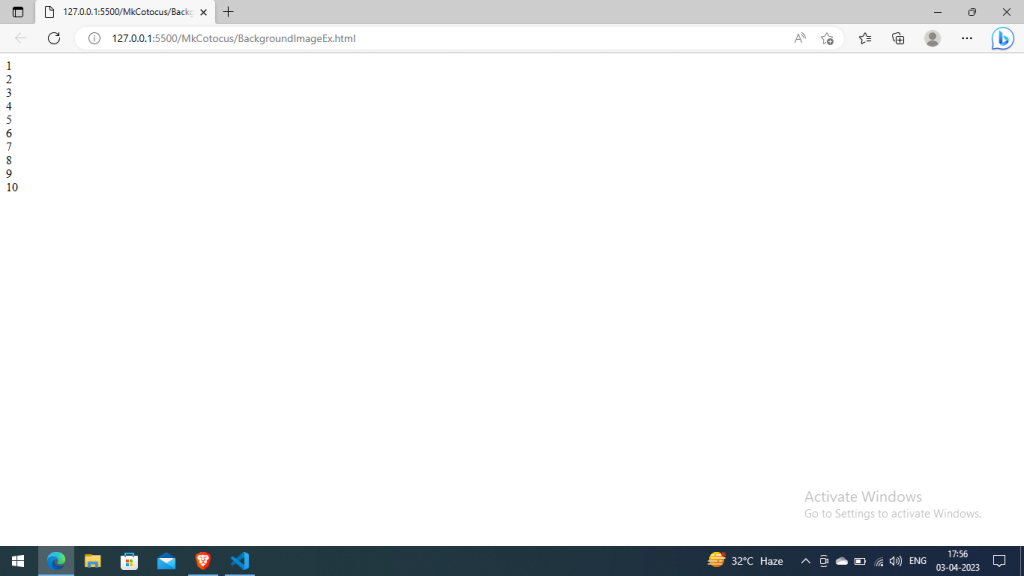
- while loop: The while loop repeats a block of code as long as the specified condition is true.
Syntax:
while (condition)
{
// code to be executed
}
3. do…while loop: The do…while loop is similar to the while loop, except that it always executes the code block at least once before checking the condition.
Syntax:
do {
// code to be executed
} while (condition);
- forEach loop: The forEach loop is used to iterate over an array or other iterable object and execute a function for each element.
Syntax:
array.forEach(function(item, index)
{
// code to be executed
});
- For-in loop: The for-in loop is used to iterate over the properties of an object.
Syntax:
for (variable in object)
{
// code to be executed
}
6. For-of loop: The for-of loop is used to iterate over the values of an iterable object, such as an array or a string.
Syntax:
for (variable of object)
{
// code to be executed
}
JavaScript Functions
JavaScript functions are blocks of code that can be defined and executed whenever needed. A function in JavaScript is a reusable block of code that performs a specific task. You can call a function multiple times, and it will execute the same set of instructions each time. Functions can also take arguments and return a value.
Example of a function that takes two arguments (num1 and num2) and returns their sum:
function addNumbers(num1, num2)
{
return num1 + num2;
}
To call this function and pass in two numbers, you would do something like this:
let result = addNumbers(5, 10);
console.log(result); // Output: 15