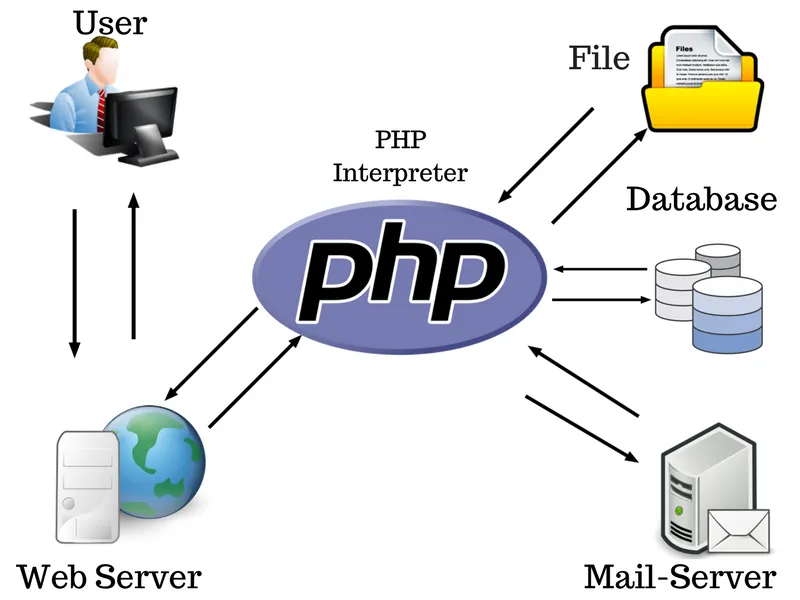
What is PHP ?
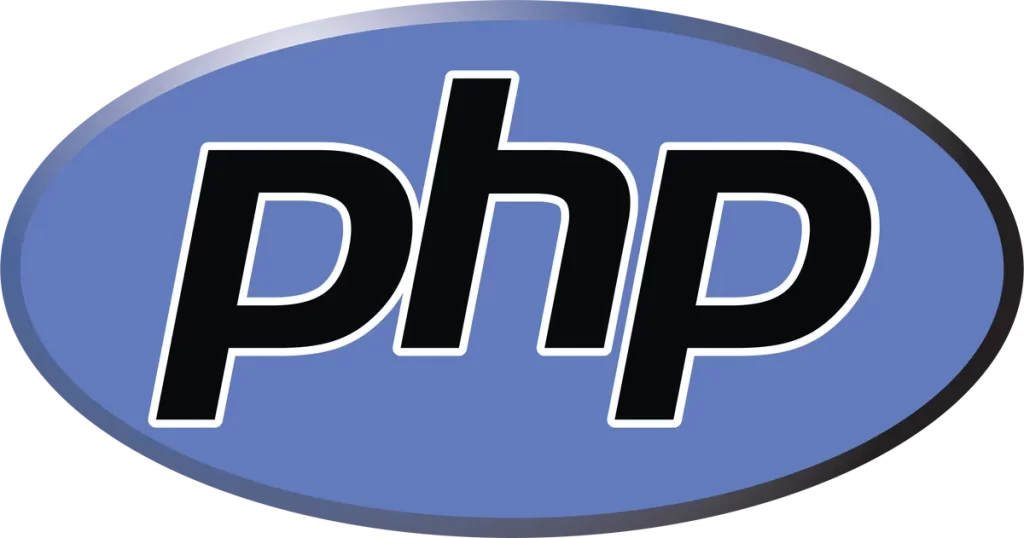
PHP stands for Hypertext Preprocessor. PHP is a server-side scripting language that is primarily used for web development. It is an open-source language that can be embedded into HTML and is executed on the server side. Therefore, it is used to develop web applications.
PHP was created by Rasmus Lerdorf in 1994 but appeared in the market in 1995. PHP 7.4.0 is the latest version of PHP, which was released on 28 November.
Some Important points are:-
- PHP stands for Hypertext Preprocessor.
- PHP was created by Rasmus Lerdorf in 1994.
- The latest version of PHP is 8.2, It was released on November 24, 2022.
- PHP is an interpreted language, i.e., there is no need for compilation.
- PHP is faster than other scripting languages, for example, ASP and JSP.
- PHP is a server-side scripting language, which is used to manage the dynamic content of the website.
- PHP can be embedded into HTML.
- PHP is an object-oriented language.
- PHP is an open-source scripting language.
- PHP is simple and easy to learn language.
Basic Syntax PHP
A PHP script can be written anywhere inside the HTML document. A PHP script starts with <?php tag and ends with ?>. We can write our logic inside this tag and it will be executed accordingly.
<?php
// PHP code goes here
?>
Displaying output in php
<?php
echo "hello";
?>
Output: hello
Basic Example:
<!DOCTYPE html>
<html>
<body>
<h1>My first PHP page</h1>
<?php
echo "Hello World!";
?>
</body>
</html>
Output: Hello World
Uses of PHP:
PHP is a server-side scripting language, which is used to design the dynamic web applications with MySQL database. There are also other uses like
- Handling Forms: PHP can handle form operations. It can gather data, save data to a file and send data through emails.
- Database Operations: PHP can also create, read, update and delete elements in your database.
- Encryption: It can perform advanced encryption and encrypt data for you.
- Dynamic Page Content: It can generate dynamic page content.
Features of PHP:
- Open Source: An open-source language, which means it’s free to use, distribute, and modify.
- Easy to Learn: It has a simple syntax that is similar to C and Perl.
- Platform Independent: Which means it can run on different operating systems, such as Windows, Linux, and macOS.
- Integration with Other Technologies: PHP can be easily integrated with other technologies, such as HTML, CSS, JavaScript, and databases like MySQL, PostgreSQL, and Oracle.
- Object-Oriented Programming (OOP): PHP supports object-oriented programming (OOP) concepts such as encapsulation, inheritance, and polymorphism.
- Scalability: PHP is highly scalable, which means it can handle large applications with ease.
- Security: PHP has built-in security features to prevent attacks like SQL injection and cross-site scripting (XSS).
- Large Community Support: PHP has a large community of developers who contribute to its development, documentation, and support.
- Fast Execution: PHP is optimized for web development, and it’s designed to execute quickly and efficiently on web servers.
PHP Comments:
A comment is a part of the coding file that are not executed by the compiler and interpreter. There are two types:-
- Single Line Comments: The Single Line comments is used to comment only one line.
<?php
// This is a single-line comment
# This is also a single-line comment
?>
2. Multiple-Line Comments: The Multiple-Line comments is used to comment only one line.
<?php
/*
This is a
multiple line
Comment.
*/
?>
Variables in PHP:
Variables are containers that can store information, a variable is declared using a $ sign followed by the variable name. Here, some important points to know about variables:
- PHP is a loosely typed language, so we do not need to declare the data types of the variables. It automatically analyzes the values and makes conversions to its correct datatype.
- After declaring a variable, it can be reused throughout the code.
- Assignment Operator (=) is used to assign the value to a variable.
Syntax of declaring a variable in PHP is given below:
$variablename=value;
Rules for declaring PHP variable:
- All variables should be denoted with a Dollar Sign ($).
- Variables are assigned with the = operator, with the variable on the left-hand side and the expression to be evaluated on the right.
- Variable names can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ).
- Variables must start with a letter or the underscore “_” character.
- Variables are case sensitive, so $name and $NAME both are different variable.
- Variable names cannot start with a number.
For Example:
<?php
$str = "Hello world!"; # String type
$x = 5; # int
type
$y = 10.5; # Float type
?>
PHP Variable Scope:
The scope of the variable is the area within which the variable has been created.
PHP has three types of variable scopes:
- Local variable
- Global variable
- Static variable
Local Variable:
A local variable is declared within a function and can be only used inside the function. This means that these variables cannot be accessed outside the function, as they have local scope.
Example:
<?php
function local_var()
{
$company = "Cotocus"; //local variable
echo "My Company Name is: " .$local_var;
}
local_var(); //Calling the function
?>
Output:
My Company Name is: Cotocus
Global variable:
A global variable is declared outside of any function or block, and can be accessed from any part of the program.
Example:
<?php
$name = "Maruti"; //Global Variable
function global_var()
{
global $name;
echo "Variable inside the function: ". $name;
echo "</br>";
}
global_var();
echo "Variable outside the function: ". $name;
?>
Output:
Variable inside the function: Maruti
Variable outside the function: Maruti
Static variable:
PHP has a feature that deletes the variable once it has finished execution and frees the memory. When we need a local variable which can store its value even after the execution, we use the static keyword before it and the variable is called static variable.
These variables only exist in a local function and do not get deleted after the execution has been completed.
Example:
<?php
function static_var()
{
static $num1 = 5; //static variable
$num2 = 7; //Non-static variable
//increment in non-static variable which will increment its value to 7
$num1++;
//increment in static variable which will increment its value to 4 after first execution and 5 after second execution
$num2++;
echo "Static: " .$num1 ."</br>";
echo "Non-static: " .$num2 ."</br>";
}
//first function call
static_var();
//second function call
static_var();
?>
Output:
Static: 6
Non-static: 8
Static: 7
Non-static: 8