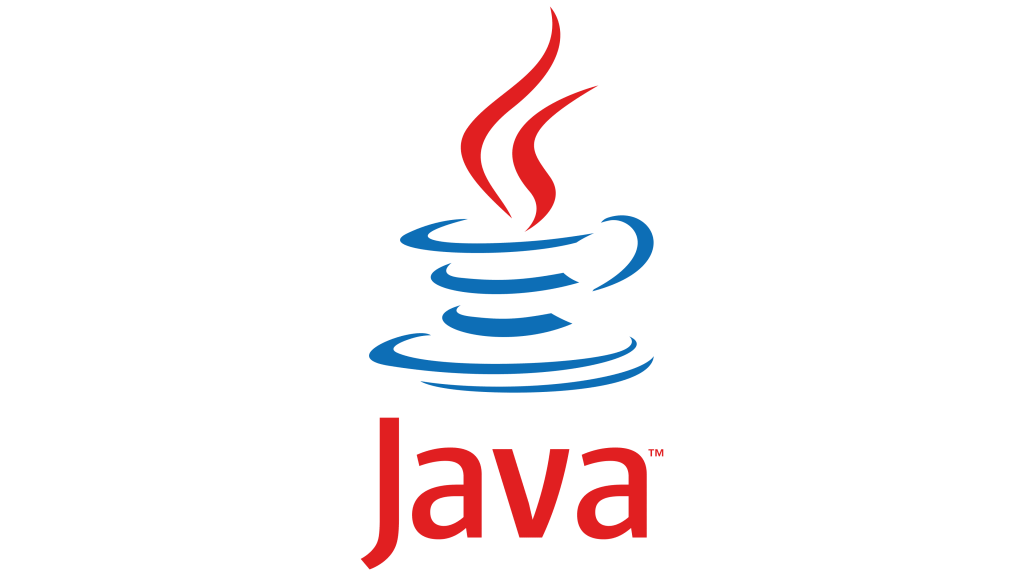
Java is a popular programming language that is used to create software applications. Java is an object-oriented language that is designed to be platform-independent, meaning that it can be run on any operating system without the need for any modifications. It was developed by Sun Microsystems in the year 1995. James Gosling is known as the father of Java. Before Java, its name was Oak. Since Oak was already a registered company, so James Gosling and his team changed the name from Oak to Java.
What are the top use cases of Java?
Here are some of the top use cases of Java:
- Web Applications: Java is commonly used to create web applications that run on servers. Java-based web applications are used by many large companies, including LinkedIn, Amazon, and Netflix.
- Mobile Applications: Java can also be used to create mobile applications for Android devices. Android Studio, which is the official development environment for Android, uses Java as its primary programming language.
- Desktop Applications: Java can also be used to create desktop applications. One example of a popular Java-based desktop application is the Eclipse IDE.
- Enterprise Applications: Java is frequently used to create enterprise-level applications, such as customer relationship management (CRM) systems, enterprise resource planning (ERP) systems, and supply chain management (SCM) systems.
What are the features of Java?
Here are some of the features of Java:
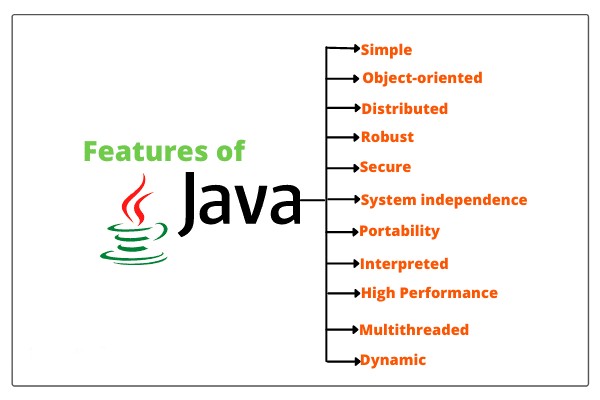
- Object-Oriented: Java is an object-oriented language, which means that it is designed to work with objects and classes. This makes it easier to write complex programs and to reuse code.
- Platform-Independent: Java is designed to be platform-independent, meaning that it can run on any operating system without the need for any modifications.
- Automatic Memory Management: Java has automatic memory management, which means that the programmer does not need to worry about deallocating memory when it is no longer needed.
- Robust: Java is designed to be robust, meaning that it can handle errors and exceptions gracefully without crashing.
- Secure: Java is designed to be secure, with features such as a built-in security manager that controls access to system resources.
What is the workflow of Java?
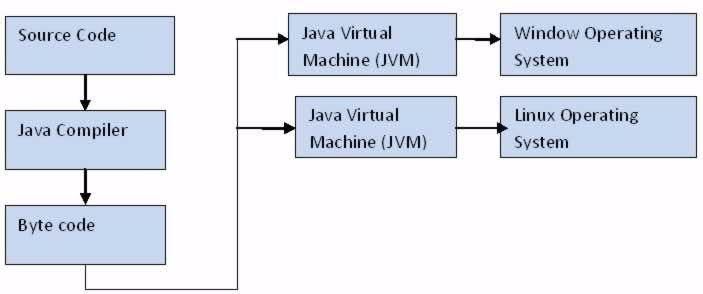
The workflow of Java can be divided into the following steps:
- Write Code: The first step in the Java workflow is to write code using a Java development environment. This can include writing classes, methods, and variables.
- Compile Code: Once the code has been written, it needs to be compiled into byte code that can be interpreted by the Java Virtual Machine (JVM).
- Execute Code: The byte code can then be executed by the JVM, which translates it into machine code that can be run on the operating system.
- Debug Code: If there are any errors in the code, they can be debugged using a debugger tool.
- Deploy Code: Once the code has been tested and debugged, it can be deployed to a server or other environment where it can be used by end-users.
How Java Works & Architecture
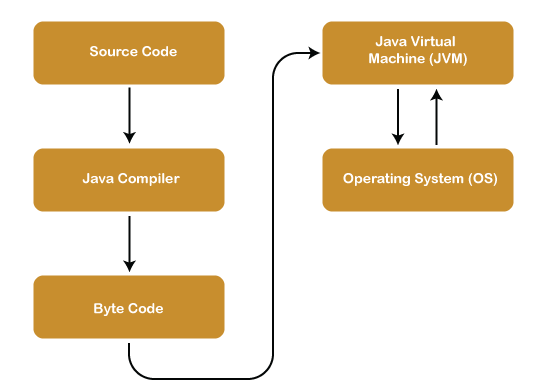
Java works by using a virtual machine that runs on the operating system. The Java Virtual Machine (JVM) is responsible for interpreting the Java byte code and translating it into machine code that can be run on the operating system.
The architecture of Java consists of three main components:
- Java Development Kit (JDK): The JDK is a software development kit that includes everything needed to develop Java applications, including the Java compiler, runtime environment, and libraries.
- Java Runtime Environment (JRE): The JRE is a runtime environment that allows Java applications to run on the operating system. It includes the JVM and other necessary components.
- Java Virtual Machine (JVM): The JVM is responsible for interpreting the Java byte code and translating it into machine code that can be run on the operating system.
How to Install and Configure Java
To install and configure Java, you can follow these steps:
- Download Java: Go to the Java download page and download the appropriate version of Java for your operating system.
- Install Java: Run the installation file and follow the prompts to install Java on your computer.
- Set Java Path: Once Java is installed, you need to set the Java path so that your computer can find the Java executable file. This can usually be done by adding the Java installation directory to your system’s PATH environment variable.
- Verify Java Installation: To verify that Java is installed and configured correctly, open a command prompt and type “java -version”. This should display the version of Java that is installed on your computer.
Fundamental Tutorials of Java ?
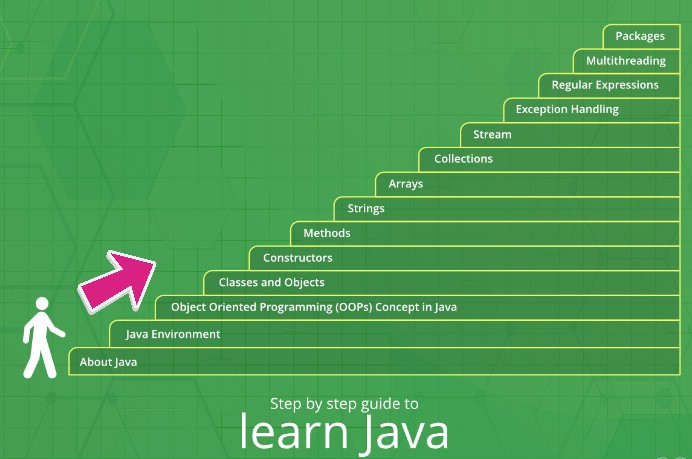
step-by-step fundamental tutorial of Java that will cover the basics of the language:
Step 1: Install Java Development Kit (JDK)
- Go to the Oracle JDK download page or OpenJDK website.
- Download the appropriate JDK version for your operating system.
- Follow the installation instructions for your OS.
Step 2: Set Up Environment Variables (Optional)
- After installing the JDK, you may set up environment variables like
JAVA_HOME
and add the JDK’sbin
directory to thePATH
variable. This step is optional but can make Java development easier.
Step 3: Set Up a Text Editor or Integrated Development Environment (IDE)
- Choose a text editor like Visual Studio Code or an IDE like Eclipse or IntelliJ IDEA for writing Java code.
Step 4: Write Your First Java Program
- Open your text editor or IDE and create a new Java file with the extension
.java
. - Write a simple “Hello, World!” program:
public class HelloWorld
{ public static void main(String[] args)
{ System.out.println("Hello, World!"); } }
Step 5: Save and Compile the Java Program
- Save the Java file with the name
HelloWorld.java
. - Open a terminal or command prompt and navigate to the directory where the file is saved.
- Compile the Java program using the
javac
command:
HelloWorld.java
Step 6: Run the Java Program
- After successful compilation, a
.class
file will be generated. - Execute the Java program using the
java
command:
java HelloWorld
Step 7: Understand the Basic Structure of a Java Program
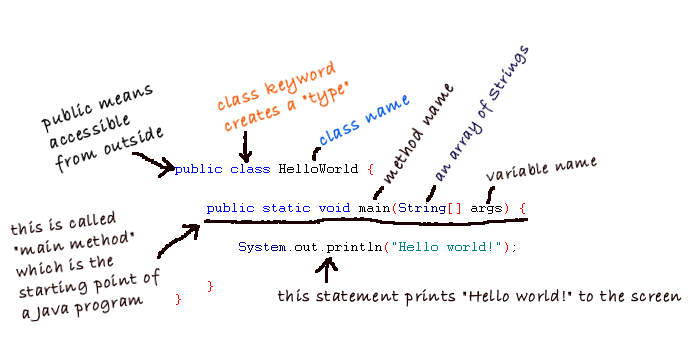
Step 8: Variables and Data Types
- Learn about different data types in Java, such as
int
,double
,char
,boolean
, etc. - Declare variables and assign values to them.
- Perform basic arithmetic operations using variables.
Step 9: Control Flow Statements
- Understand conditional statements like
if
,else if
, andswitch
. - Use loop statements like
for
,while
, anddo-while
to control program flow.
Step 10: Functions (Methods)
- Learn about functions in Java (also called methods).
- Create custom methods to perform specific tasks.
- Understand method parameters and return values.
Step 11: Object-Oriented Programming (OOP) Concepts
- Explore the principles of OOP, such as encapsulation, inheritance, polymorphism, and abstraction.
- Create classes and objects to represent real-world entities.
Step 12: Arrays and Collections
- Learn about arrays and how to work with them in Java.
- Understand Java Collections framework and use collections like
ArrayList
andHashMap
.
Step 13: Exception Handling
- Learn about handling exceptions in Java using
try
,catch
,finally
blocks. - Handle common exceptions to make your code more robust.
Step 14: Input and Output
- Read user input from the console using
Scanner
class. - Write output to files using Java’s Input/Output (I/O) classes.
Step 15: Basic File Handling
- Learn how to read and write files in Java using
File
,FileReader
, andFileWriter
classes.
Step 16: Introduction to Java Libraries
- Explore some of the standard libraries in Java, like
java.lang
,java.util
, etc. - Understand how to use library classes and methods to simplify coding tasks.
Step 17: Practice, Practice, Practice!
- The best way to master Java is through practice. Work on small projects and exercises to reinforce your learning.