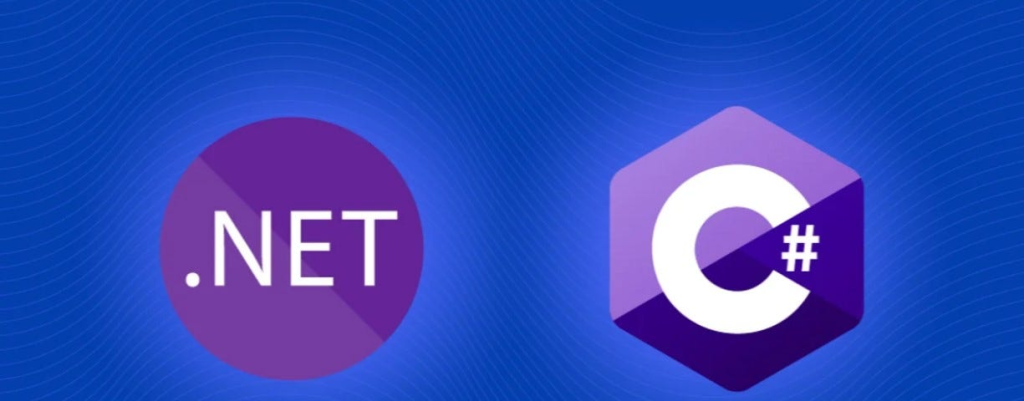
C# is pronounced “C-Sharp”, C# is a modern, object-oriented, general-purpose programming language developed by Microsoft. It was introduced in the early 2000s as part of the .NET framework and has since become one of the most popular programming languages. C# is a powerful language that can be used to create a wide variety of applications, including web applications, desktop applications, and mobile applications and more.
What are feature of C# ?
Some of the key features of C# include:
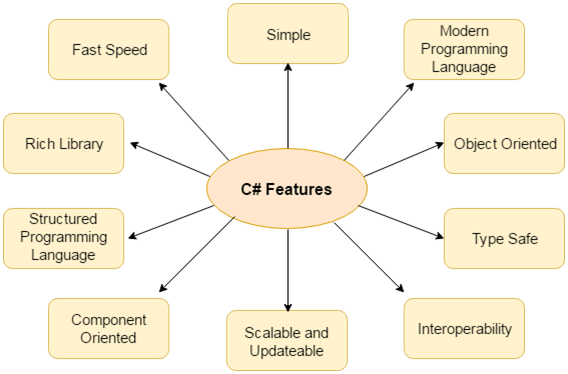
- Object-Oriented: C# is a fully object-oriented language, allowing developers to create and use classes, objects, inheritance, polymorphism, and encapsulation.
- Garbage Collection: C# uses automatic garbage collection to manage memory, making memory management easier for developers.
- Interoperability: C# is interoperable with other programming languages, such as C++, Java, and Visual Basic.
- Type Safe: C# type safe code can only access the memory location that it has permission to execute. Therefore it improves a security of the program.
- Rich Library: C# provides a lot of inbuilt functions that makes the development fast.
- Fast Speed: The compilation and execution time of C# language is fast.
- Type-Safe: C# enforces strong type checking, which helps prevent type-related errors during the development process.
- Asynchronous programming: C# supports asynchronous programming, which allows you to write code that runs in the background while the main thread of execution continues.
- Scalable and Updateable: C# is a programming language that allows for easy updates and scalability. When we want to update our application, we replace the old files with the new ones to ensure the latest version is running. This simplicity makes it convenient to keep our software up-to-date with the latest features and improvements
- Structured Programming Language: C# is a structured programming language in the sense that we can break the program into parts using functions. So, it is easy to understand and modify.
What is the workflow of C# ?
- Writing Code: Start by writing C# code using a text editor or an integrated development environment (IDE) like Visual Studio.
- Compilation: Once the code is written, it needs to be compiled into an intermediate language called Common Intermediate Language (CIL) or Microsoft Intermediate Language (MSIL). This compilation is done by the C# compiler (csc.exe).
- Intermediate Language and JIT Compilation: The compiled code is then executed by the Common Language Runtime (CLR), which converts the CIL/MSIL code into native machine code through Just-In-Time (JIT) compilation.
- Execution: The JIT-compiled native code is executed on the target machine.
How C# Works & Architecture?
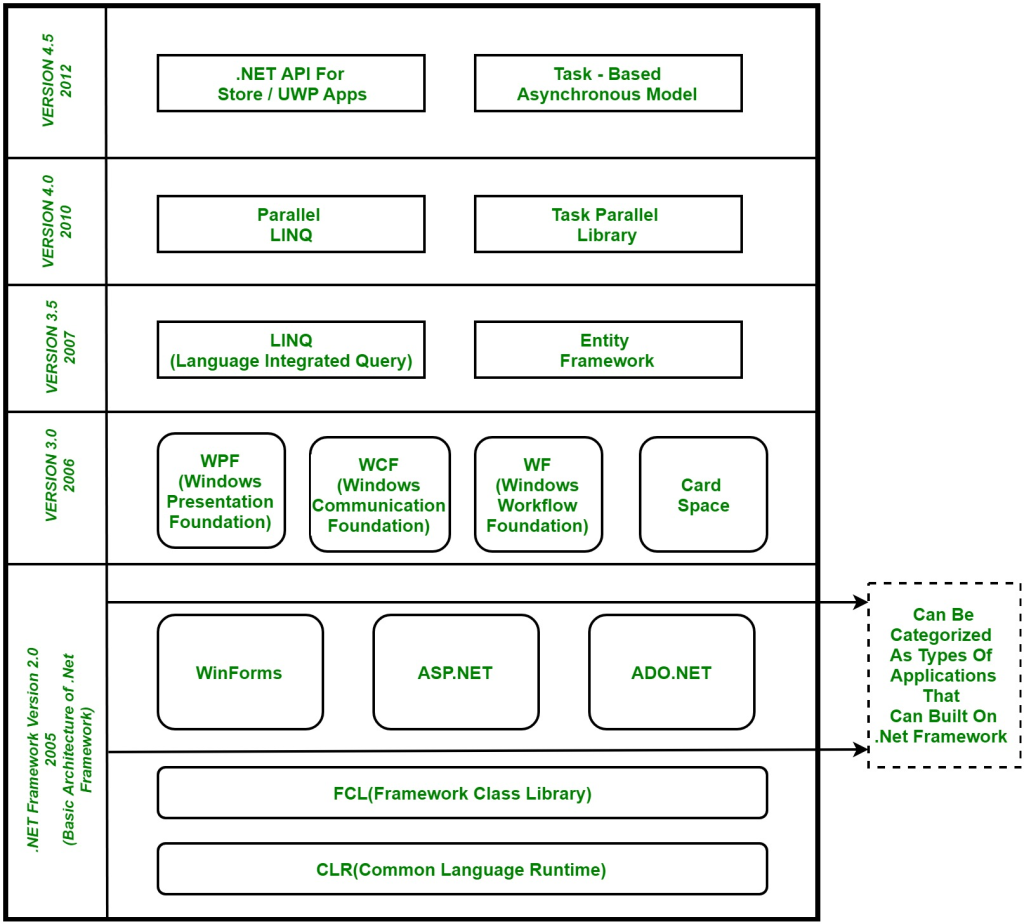
- Source Code (.cs files): C# code is written in text files with a .cs extension.
- C# Compiler: The C# compiler (csc.exe) compiles the C# source code into intermediate language code (CIL/MSIL).
- Common Language Infrastructure (CLI): CIL/MSIL code is platform-independent and runs on any system with a CLI implementation.
- Common Language Runtime (CLR): The CLR is responsible for loading and executing the CIL/MSIL code. It provides services like memory management (garbage collection), security, and exception handling.
- JIT Compilation: When the CIL/MSIL code is executed, the CLR uses Just-In-Time (JIT) compilation to convert it into native machine code, specific to the target platform, for better performance.
How to Install and Configure C# ?
To install and configure C#, you will need to:
- Download and install the .NET Framework. This is the foundation on which C# is built, and it is required to run C# applications. You can download the .NET Framework from the Microsoft website: https://dotnet.microsoft.com/en-us/download/dotnet-framework.
- Install a C# IDE. There are many different C# IDEs available, such as Visual Studio, Visual Studio Code, and JetBrains Rider. Each IDE has its own strengths and weaknesses, so you may want to try a few different ones before you decide which one you like best.
- Configure your IDE. Once you have installed an IDE, you will need to configure it for C# development. This typically involves setting the project type to “C#” and selecting the appropriate .NET Framework version.
How to Download and Install Visual Studio for C# in Windows
Step 1) Download Visual Studio
First, visit the following Visual Studio free download link
https://visualstudio.microsoft.com/downloads/
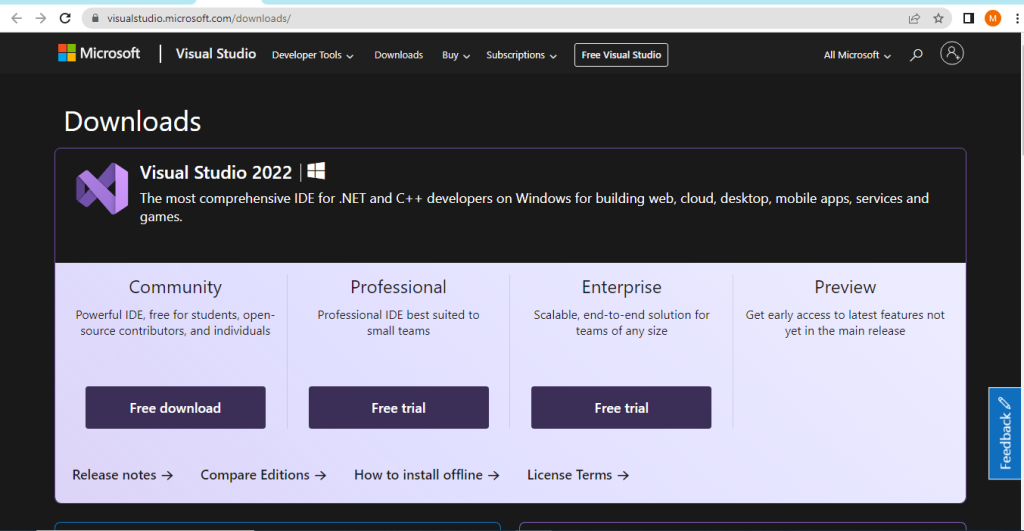
You can select
- Visual Studio 2022 Community Edition
- Visual Studio 2022 Professional Edition (30 Day Free Trial)
Step 2) Open the .exe file
Click on the free downloaded option
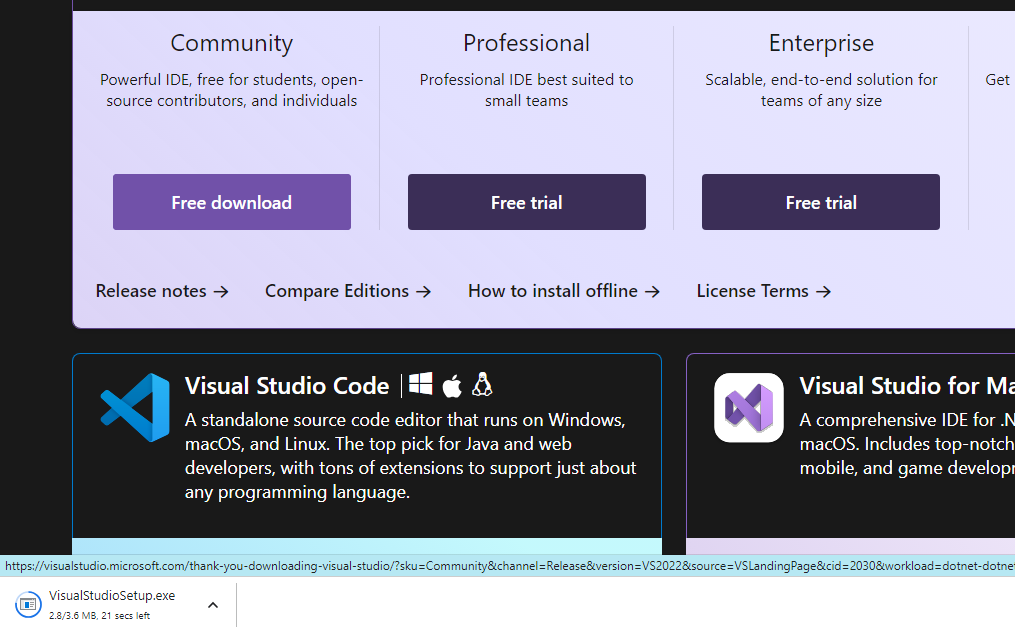
Step 3) Start the installation
In the next screen, click continue to start Visual Studio installation.
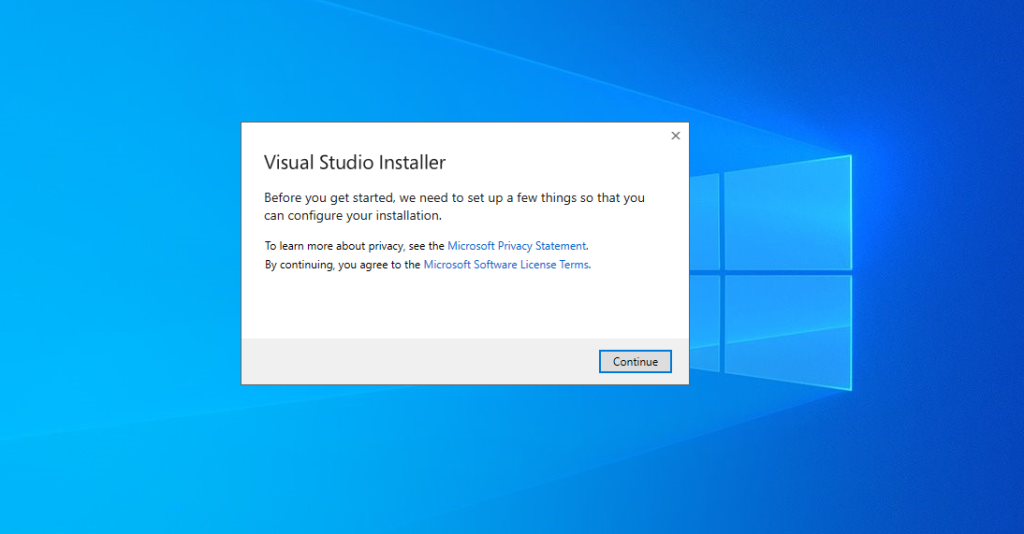
Step 4) Let the installation complete
Visual Studio will start downloading the initial files. Download speed will vary as per your internet connection.
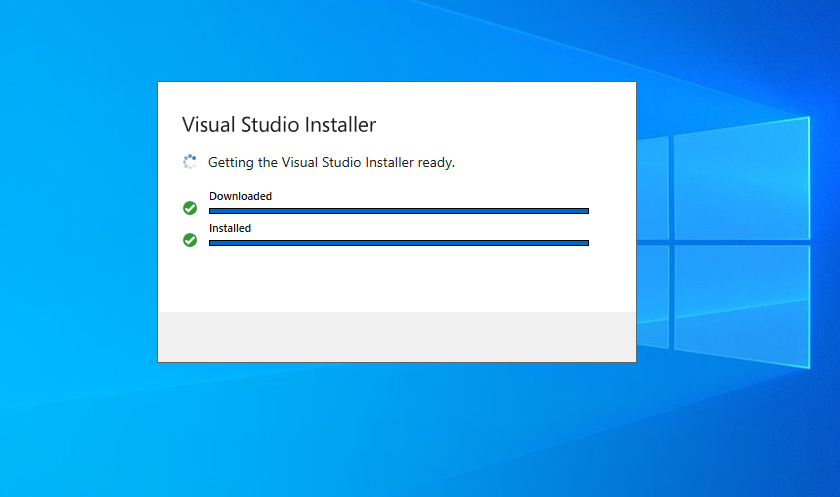
Step 5) Choose the software version
In next screen, click install
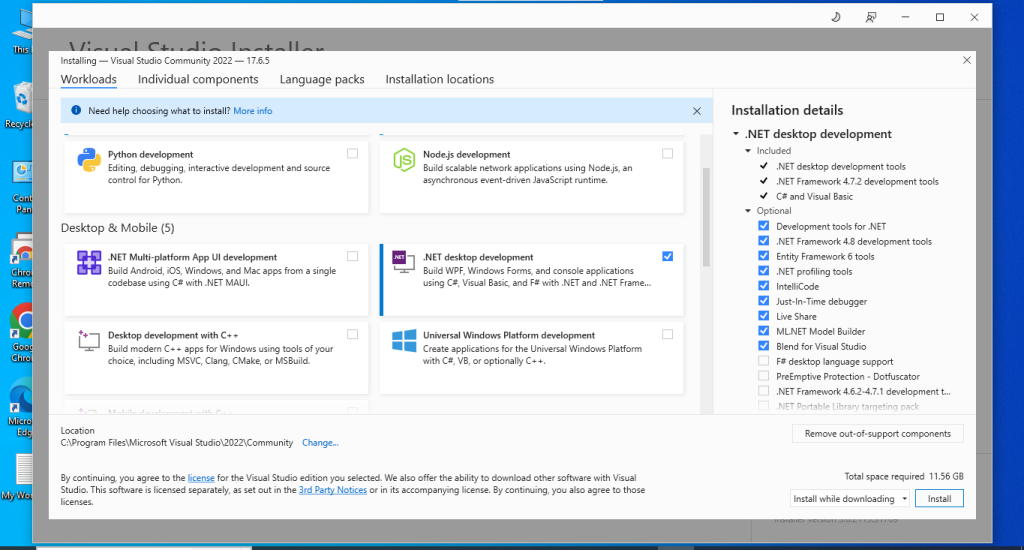
Step 6) Select the desktop version
In next screen,
- Select “.Net desktop development”
- Click install
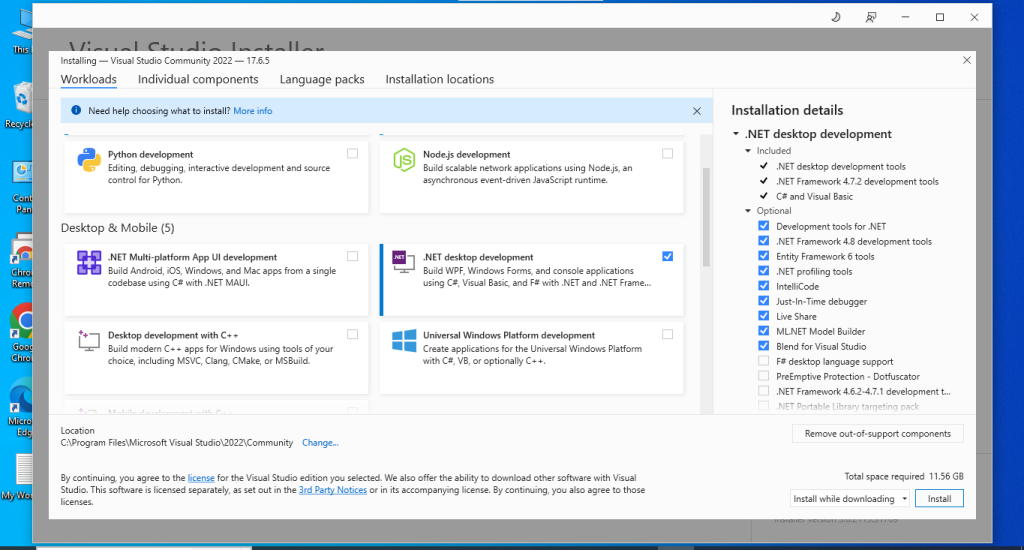
Step 7) Wait for the files to be downloaded
Visual Studio will download the relevant files based on the selection in step 6
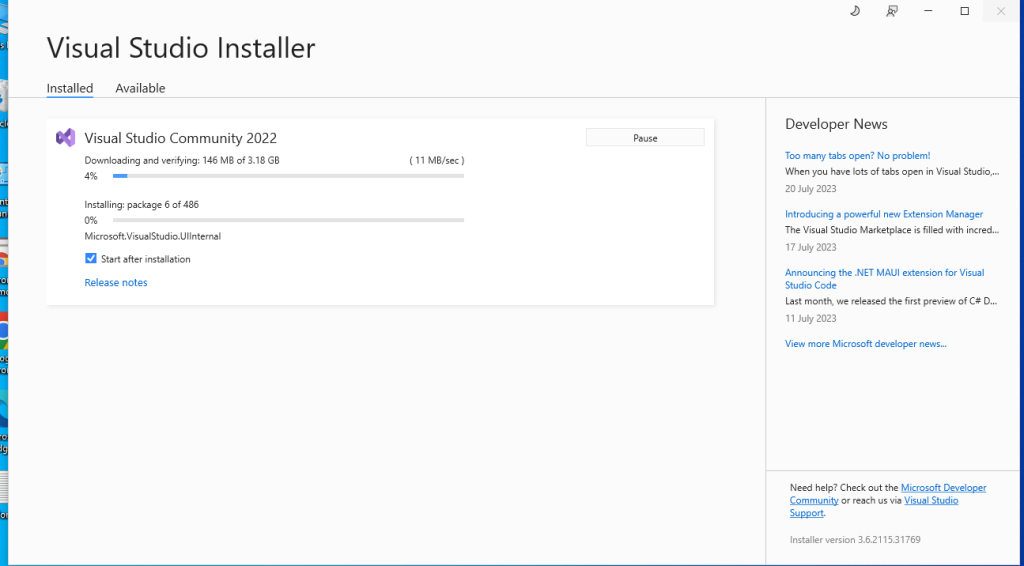
Step 8) Reboot your PC
Once the download is done, you will be asked to reboot the PC to complete Visual Studio setup
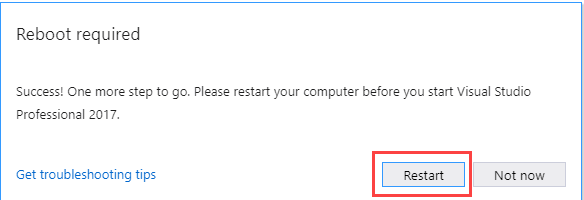
Step 9) Open Visual Studio
Post reboot, open the Visual Studio IDE
- Select a theme of your choice
- Click Start Visual Studio
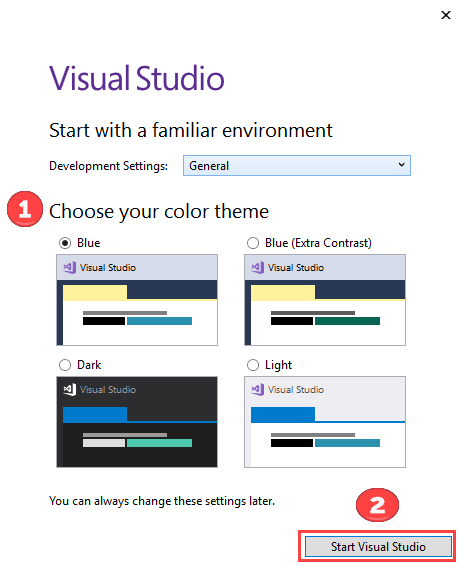
Step 10) Start using Visual Studio
In Visual Studio IDE, you can navigate to File menu to create new C# applications.
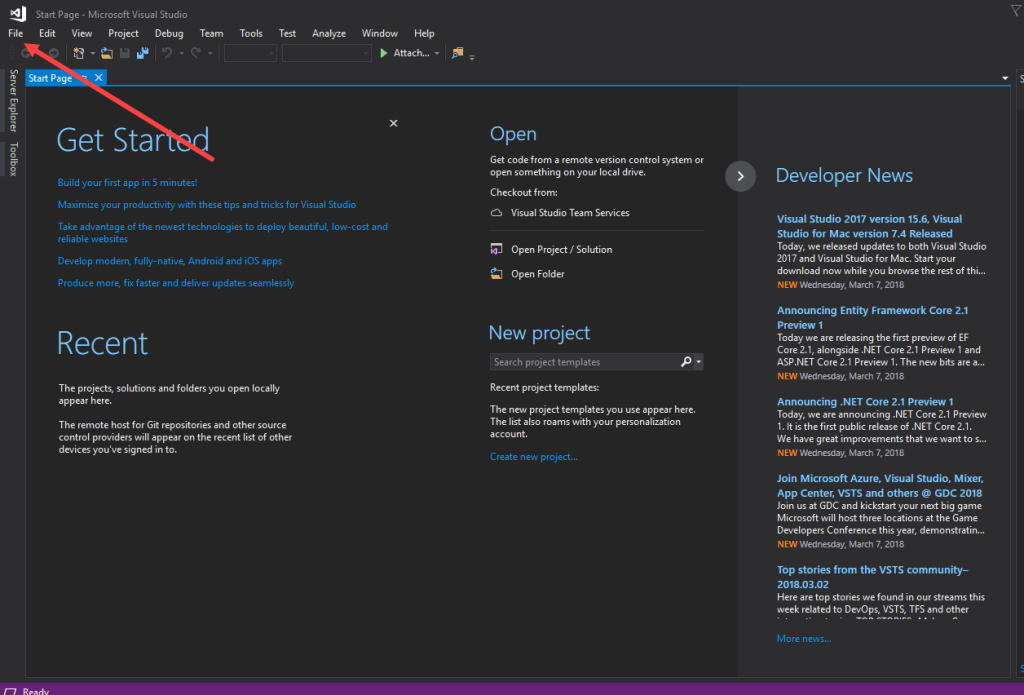
That’s it to Visual Studio for C# installation.
Fundamental Tutorials of C# ?
Here are the step-by-step instructions on how to write a “Hello, World!” program in C#:
- Open a text editor and create a new file.
- Save the file with the .cs extension.
- Write the following code in the file:
C#
// Hello, World! program
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
- Save the file and compile it.
- Run the program.
The program should print the following output to the console:
Hello, World!
Explanations of these code
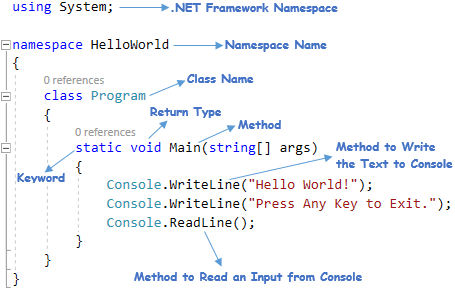