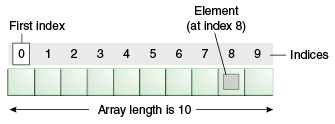
What is arrays ?
An array is a data structure that stores a collection of elements of the same data type. The elements of an array are called its elements or members. Arrays are typically indexed, which means that each element can be accessed by its index. The index of an element is an integer that specifies its position in the array.
For example, the following array stores 5 integers:
int[] myArray = {1, 2, 3, 4, 5};
The first element of the array is 1, the second element is 2, and so on. The index of the first element is 0, the index of the second element is 1, and so on.
Arrays are a very common data structure in computer programming. They are used to store a variety of data, such as lists of numbers, strings, and objects. Arrays are also used to implement other data structures, such as queues, stacks, and linked lists.
What is top use cases of arrays?
Arrays have various use cases in programming. Here are some common scenarios where arrays are used:
- Storing and accessing a list of items: Arrays are often used to store and retrieve a collection of items, such as a list of numbers, names, or any other data type.
- Sorting and searching algorithms: Arrays are essential for implementing sorting and searching algorithms. For example, you can use an array to store a list of numbers and then use sorting algorithms like bubble sort or quicksort to sort the array in ascending or descending order.
- Managing data structures: Arrays are used to implement other data structures like stacks, queues, and hash tables. These data structures rely on arrays to store and manage their elements effectively.
What are feature of arrays ?
Features of Arrays:
- Fixed Size: Arrays have a fixed size defined during their creation, which cannot be easily changed.
- Contiguous Memory: Elements in an array are stored in adjacent memory locations, allowing for efficient access.
- Indexed Access: Elements in an array are accessed using an index, which indicates their position in the array.
- Homogeneous Elements: Arrays store elements of the same data type, ensuring uniformity in the data structure.
- Constant-Time Access: Accessing elements by index in an array is typically a constant-time operation.
- Memory Efficiency: Arrays are memory-efficient due to their contiguous storage, compared to more complex data structures.
- Efficient Iteration: Arrays allow for efficient iteration using simple loop constructs.
What is the workflow of arrays?
To understand how arrays work, let’s take a look at the typical workflow:
- Declare an array: Start by declaring an array variable and specifying the data type and size of the array. For example, you can declare an integer array of size 5.
- Initialize the array: After declaring the array, you can initialize the elements by assigning values to each index of the array. For example, you can assign values like 10, 20, 30, 40, and 50 to the array.
- Access and manipulate elements: You can access and manipulate individual elements of the array using their index. For example, you can retrieve the value at index 2 or change the value at index 3.
- Perform operations: You can perform various operations on arrays, such as sorting, searching, inserting, or deleting elements. These operations help in managing and manipulating the data stored in the array.
How arrays Works & Architecture?
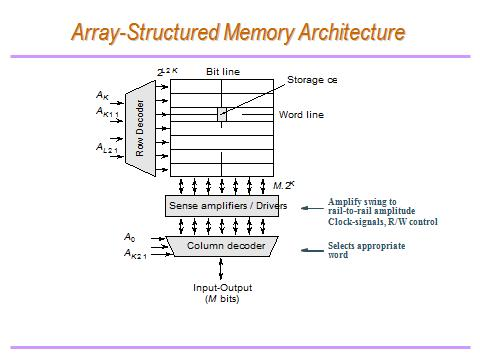
The workflow of arrays generally involves the following steps:
1. Declaration: Start by declaring an array variable and specifying its data type.
2. Initialization: Assign initial values to the array elements. This step can be skipped if you prefer to assign values later on.
3. Accessing elements: Use the array index to access or modify individual elements. Array indices typically start from 0 and go up to the size of the array minus one.
4. Iteration: Employ loops (e.g., for loop, while loop) to iterate through the array elements and perform operations on them collectively.
5. Manipulation: Perform various operations like insertion, deletion, or modification of array elements based on the desired logic.
6. Searching: Implement searching algorithms, such as linear search or binary search, to find specific elements within the array.
7. Sorting: Utilize sorting techniques like bubble sort, insertion sort, or merge sort to arrange the elements of the array in a specific order.
How to Install and Configure arrays ?
To install and configure arrays in your programming language, follow these general steps:
1. Install a programming language: Choose a programming language that supports arrays, such as Python, Java, C++, or JavaScript. Install the necessary development environment and tools for that language.
2. Create an array: Declare an array variable and allocate memory for it. The syntax may differ based on the programming language you are using. For example, in Python, you can create an array using a list: `my_array = []`.
3. Initialize the array: If needed, assign initial values to the array elements. You can do this using loops or directly assigning values to individual array elements, depending on your requirements.
4. Access array elements: Use the appropriate syntax to access and modify elements in the array. In most languages, array indexing starts at 0. For example, in Python, to access the first element of the array: `first_element = my_array[0]`.
5. Perform operations on arrays: Arrays support various operations, such as adding or removing elements, sorting, searching, or iterating over the elements. Refer to the specific programming language’s documentation or tutorials to learn more about array operations.
Step by Step Tutorials for array for hello world program in php
Here’s a step-by-step tutorial for creating a “Hello, World!” program using arrays in PHP:
Step 1: Open a text editor or PHP development environment.
Step 2: Create a new PHP file and save it with a “.php” extension, for example, “hello.php”.
Step 3: Start by declaring an array variable in PHP to hold the words “Hello” and “World”. You can do it using the array() function or a shorthand syntax []:
$words = array("Hello", "World");
// or
$words = ["Hello", "World"];
Step 4: Use the array elements to construct your desired output message. In this case, we’ll concatenate the elements with a space in between:
$message = $words[0] . " " . $words[1] . "!";
Step 5: Display the final message using the “echo” statement:
echo $message;
Step 6: Save the file and navigate to it in your web browser.
Step 7: You should see the output “Hello World!” displayed on the screen.
That’s it! You’ve successfully created a “Hello, World!” program using arrays in PHP. You can modify and expand upon this basic example to explore more functionalities of arrays.