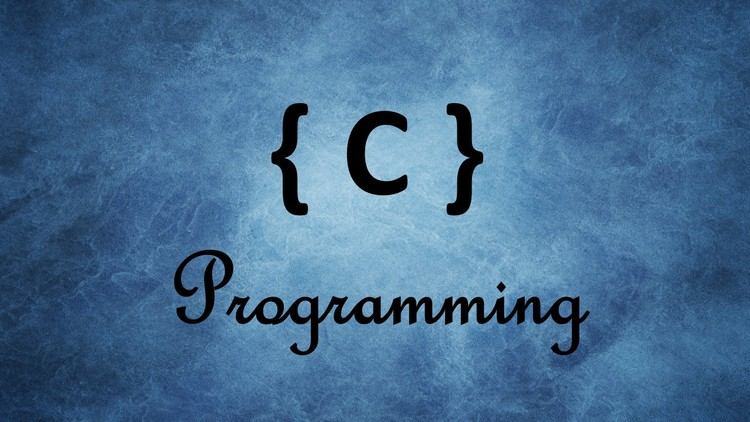
What is C Programming?
C programming is a general-purpose, procedural programming language that was developed in the early 1970s. It was designed by Dennis Ritchie at Bell Labs as an evolution of the B programming language. C is known for its simplicity, flexibility, and low-level capabilities, making it well-suited for system programming, application development, and embedded systems.
What is top use cases of C Programming?
Some of the top use cases of C programming include:
1. Operating Systems: C programming is widely used in the development of operating systems like Unix, Linux, and Windows.
2. Embedded Systems: Due to its low-level capabilities, C is commonly used in developing firmware and software for embedded systems like microcontrollers and IoT devices.
3. Game Development: C is often used in game development to optimize performance and control hardware directly.
4. System Software: Many system utilities and software tools are written in C for efficient resource utilization and low-level access.
5. Device Drivers: C is used for writing device drivers that enable communication between hardware devices and the operating system.
6. Networking: C programming is widely used in network programming to develop protocols, servers, and network applications.
What are feature of C Programming?
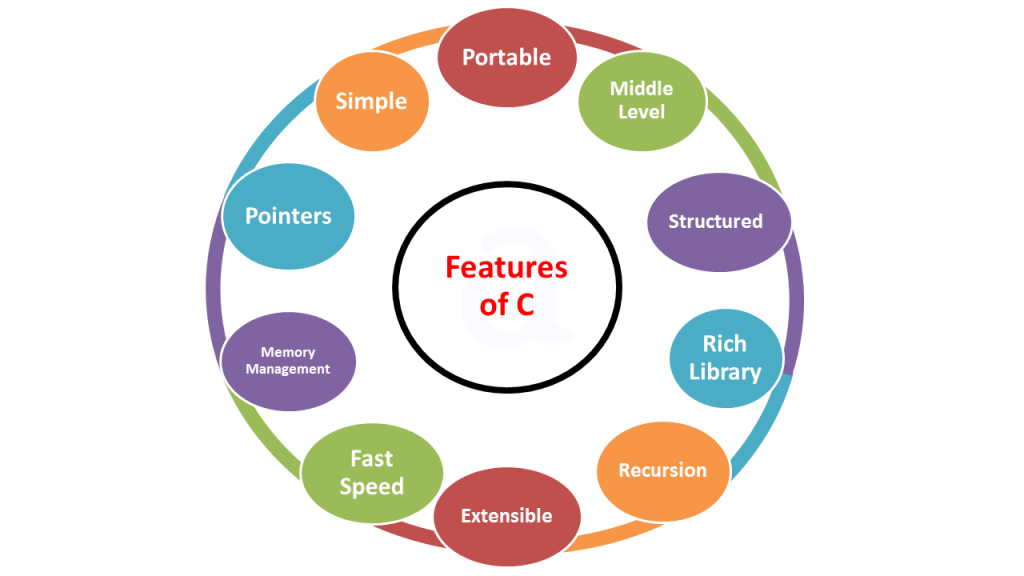
Some features of C programming include:
1. Low-level Access: C allows direct memory manipulation and provides low-level features to interact with hardware.
2. Portability: C programs can be easily ported across different platforms and operating systems.
3. Efficiency: C provides fine-grained control over system resources, making it highly efficient.
4. Modularity: C supports modular programming through functions and libraries, making code organization easier.
5. Extensibility: C allows for easy integration of assembly language code and other languages.
What is the workflow of C Programming?
The workflow of C Programming typically involves the following steps:
- Problem Analysis: Identify the problem and define the requirements of the software application.
- Algorithm Design: Develop an algorithm to solve the problem and break it down into smaller tasks.
- Coding: Write the code in C Programming language based on the algorithm.
- Compilation: Compile the code using a C compiler to convert it into machine-readable instructions.
- Testing: Test the program to ensure it functions as expected and meets the requirements.
- Debugging: Identify and fix any errors or bugs in the code.
- Documentation: Document the code and provide instructions for its usage and maintenance.
How C Programming Works & Architecture?
Here is a brief explanation of how C programming works and its architecture:
1. Code Compilation: C programs are written in a high-level language with human-readable syntax. These programs need to be compiled into machine-readable code before execution. The process involves using a C compiler that translates the C code into machine code, specific to the target hardware and operating system.
2. Program Execution: Once the C code is compiled, the resulting machine code can be executed by the computer’s processor. At runtime, the operating system manages memory, peripherals, and other hardware resources required by the program.
3. Memory Management: In C, memory is managed explicitly, meaning the programmer is responsible for allocating and freeing memory. C provides concepts like variables, pointers, and dynamic memory allocation, allowing fine-grained control over memory usage.
4. Modular Structure: C programs are structured using functions and modules. Functions encapsulate related code blocks, allowing code reuse and logical organization. Modules, also known as header files, contain function prototypes and global variable declarations, ensuring proper separation and reusability of code.
5. Hardware Access: C programming provides direct access to hardware through the use of pointers and low-level constructs. This capability allows efficient interactions with hardware devices, such as input/output operations and device driver development.
6. Standard Libraries: C comes with a rich set of standard libraries that provide pre-built functions for various tasks, such as mathematical calculations, input/output operations, string operations, and more. These libraries simplify programming by providing common functionality, saving developers from reinventing the wheel.
How to Install and Configure C Programming?
To install and configure C programming, follow these steps:
- Choose a C compiler: You can choose from various C compilers such as GCC (GNU Compiler Collection), Clang, Microsoft Visual C++, etc.
- Install the compiler based on your operating system:
- For Windows:
- GCC: Install MinGW-w64 (https://mingw-w64.org/doku.php) or Cygwin (https://www.cygwin.com/).
- Clang: Download the Clang compiler (https://releases.llvm.org/download.html) and set it up.
- Microsoft Visual C++: Download and install Visual Studio (https://visualstudio.microsoft.com/) and select the C++ development workload during installation.
- For macOS:
- GCC: Install Xcode Command Line Tools by running
xcode-select --install
in the terminal. - Clang: Clang is already installed with Xcode.
- GCC: Install Xcode Command Line Tools by running
- For Linux:
- GCC: Install GCC using your package manager. For Ubuntu, run
sudo apt install build-essential
. - Clang: Install Clang using your package manager. For Ubuntu, run
sudo apt install clang
.
- GCC: Install GCC using your package manager. For Ubuntu, run
- Verify the installation: Open a terminal or command prompt and type
gcc --version
orclang --version
to confirm that the compiler is installed correctly. - Configure your code editor or IDE: Choose a code editor or integrated development environment (IDE) for writing C code. Some popular options are Visual Studio Code (VS Code), Code::Blocks, Eclipse, or Xcode.
- Set up build tools: If you’re using a code editor like VS Code, you may need to install additional extensions or configure build tasks to compile and run your C programs seamlessly.
Once you’ve completed these steps, you’re ready to write and compile C programs on your system.
Step by Step Tutorials for C Programming for hello world program
For a step-by-step tutorial on creating a “Hello, World!” program in C, you can refer to this guide:
1. Create a new file with a .c extension, for example, hello.c.
2. Open the file in a text editor or an IDE.
3. Type the following code:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
4. Save the file.
5. Open a command prompt/terminal and navigate to the directory where the file is saved.
6. Compile the program by running the command: `gcc hello.c -o hello`
7. Run the program by executing the command: `./hello` You should see the output “Hello, World!” displayed in the terminal.