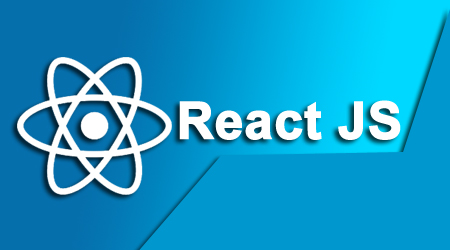
What is ReactJs ?
Reactjs is an open-source, front-end JavaScript library developed by Facebook. It is used to build interactive and reusable UI components for web applications. React uses a virtual DOM (Document Object Model) for efficient rendering and provides a component-based architecture, making it easier to manage and update complex UIs.
What is top use cases of ReactJs ?
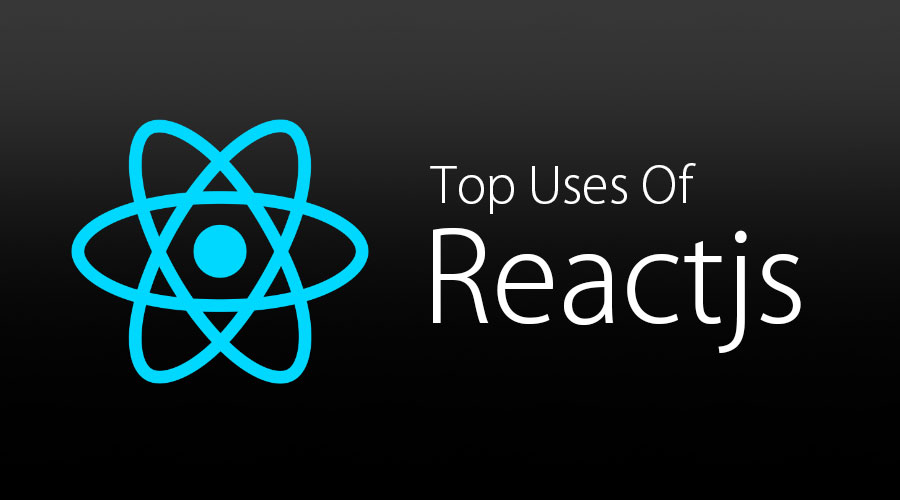
- Single-page Applications (SPAs): ReactJS is commonly used to build SPAs, where all the necessary code is loaded once and the application dynamically updates the content without refreshing the entire page.
- Interactive UIs: ReactJS is great for creating highly interactive user interfaces with rich functionalities. It enables developers to build complex UIs with ease by breaking them down into smaller, reusable components.
- Mobile App Development: React Native, a framework built on top of ReactJS, allows developers to build native mobile apps for iOS and Android using JavaScript. This makes it easier to develop cross-platform applications with a single codebase.
What are feature of ReactJs ?
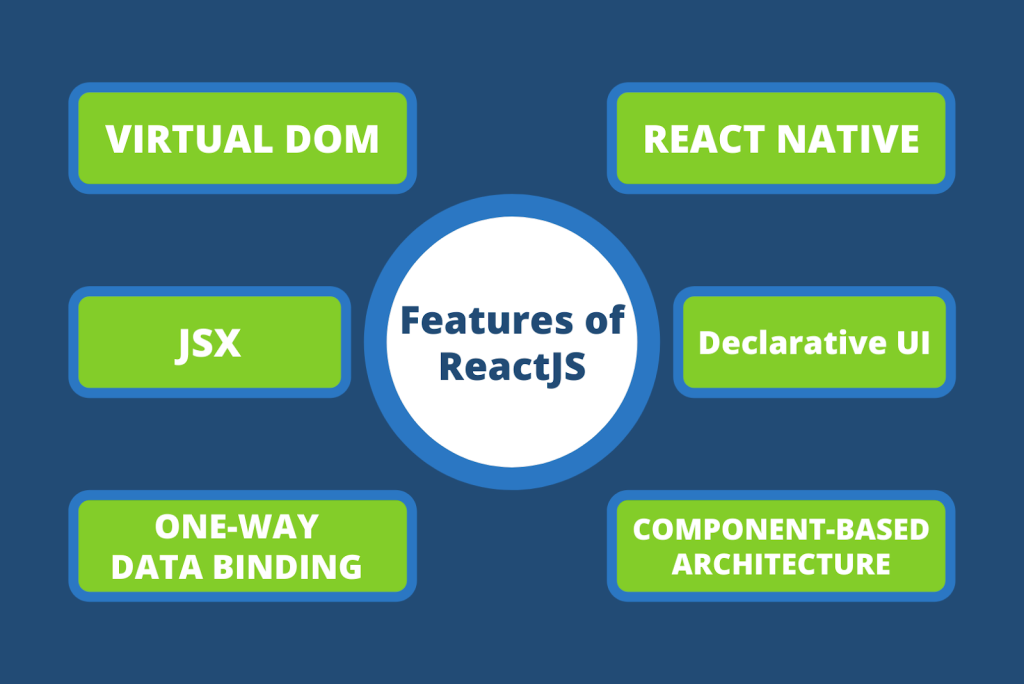
ReactJS is a popular JavaScript library used for building user interfaces in web applications. Some of the key features of ReactJS include:
- Component-based architecture: React divides the UI into reusable components, making it easy to manage and maintain complex user interfaces.
2. Virtual DOM: React uses a virtual representation of the DOM, enabling efficient updates as it only updates the necessary parts of the actual DOM, resulting in improved performance.
3. JSX: React allows developers to write HTML-like syntax within JavaScript, known as JSX. This makes it easier to describe and render components.
4. Unidirectional data flow: React follows a unidirectional data flow. Data flows in a single direction, from parent components down to child components. This helps in maintaining a predictable state and makes debugging easier.
5. Reusable components: React promotes the reusability of components, which increases development efficiency and helps maintain a consistent UI design throughout the application.
6. Fast rendering: React optimizes the rendering process by minimizing direct manipulation of the actual DOM. It efficiently updates only the changed parts in the virtual DOM and reconciles them with the actual DOM.
7. React Native: React can also be used for building cross-platform mobile applications using React Native. It allows developers to write code once and deploy it on both iOS and Android platforms.
What is the workflow of ReactJs ?
- Create a React component. A React component is a reusable piece of code that defines the UI for a specific part of your application.
- Import the component. Once you have created a React component, you need to import it into your application.
- Render the component to the DOM. Once you have imported the component, you can render it to the DOM by calling the
render()
method on the component. - Listen for events and update the component state. React components can listen for events, such as clicks and button presses. When an event occurs, the component can update its state.
- Re-render the component when the state changes. When the state of a component changes, the component is re-rendered. This means that the UI will be updated to reflect the changes in state.
Here is an example of the basic workflow of ReactJS:
// Create a React component
const MyComponent = () => {
const [name, setName] = useState("John Doe");
return (
<div>
<h1>Hello, {name}</h1>
<input type="text" value={name} onChange={setName} />
</div>
);
};
// Import the component
import MyComponent from "./MyComponent";
// Render the component to the DOM
ReactDOM.render(<MyComponent />, document.getElementById("root"));
// Listen for events
const button = document.getElementById("change-name");
button.addEventListener("click", () => {
setName("Jane Doe");
});
// Update the component state
setName("Jane Doe");
This code creates a React component called MyComponent
. The MyComponent
component has a state variable called name
. The name
variable stores the current value of the user’s name.
The MyComponent
component also listens for the click
event on the change-name
button. When the change-name
button is clicked, the setName()
function is called. The setName()
function updates the value of the name
variable.
When the name
variable changes, the MyComponent
component is re-rendered. This means that the UI is updated to reflect the changes in state.
How Reactjs Works & Architecture ?
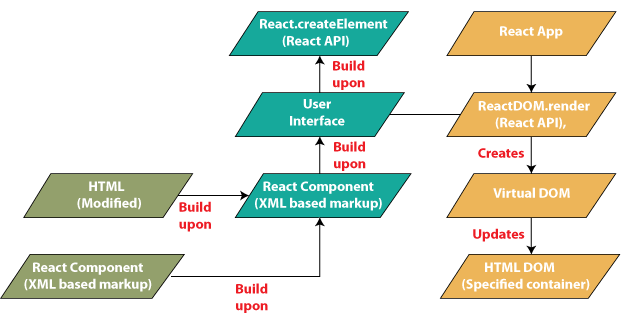
Here is a high-level overview of how React works and its architecture:
1. Component-Based Architecture: React organizes the UI into reusable components. These components are self-contained and can be nested within each other to build complex interfaces.
2. JSX (JavaScript XML): React uses JSX (JavaScript XML), which allows you to write HTML-like code within JavaScript. JSX is then transpiled into regular JavaScript using tools like Babel.
3. Virtual DOM: React creates a lightweight representation of the actual DOM known as the virtual DOM. When a component’s state changes, React compares the virtual DOM with the previous state of the virtual DOM. It identifies the minimal changes required and updates only those parts of the actual DOM.
4. Reconciliation: React uses a process called reconciliation to efficiently update the DOM. It compares the previous and current states of the virtual DOM and determines the minimal set of changes needed to fully update the UI.
5. One-way data flow: React follows a one-way data flow, also known as unidirectional data flow. This means that data flows down from parent components to child components through props (properties). When the data changes, React re-renders the affected components.
6. Virtual DOM diffing: To optimize performance, React uses a diffing algorithm to identify which parts of the virtual DOM need to be updated. This minimizes the number of actual DOM manipulations and improves rendering speed.
7. Component lifecycle methods: React provides various lifecycle methods that allow you to perform actions at different stages of a component’s lifecycle, such as component mount, update, or unmount. These methods enable you to manage state, update UI, or interact with external data sources.
How to Install and Configure ReactJs ?
To install and configure React.js, follow these steps:
- Install Node.js: React.js requires Node.js to be installed on your machine. Go to the official Node.js website (https://nodejs.org) and download the latest LTS version suitable for your operating system. Follow the installation instructions provided.
- Create a New React Project: Open your terminal/command prompt and navigate to the directory where you want to create your new React project. Use the following command to create a new React application using the “create-react-app” command-line tool:
npx create-react-app my-app
Replace my-app
with the desired name of your project.
- Change Directory: Navigate inside the newly created project directory using the following command:
cd my-app
- Start the React Development Server: Run the following command to start the React development server:
npm start
This will compile your React code and run the development server. You can access your React application by opening a web browser and visiting http://localhost:3000.
That’s it! You have successfully installed and configured React.js on your machine. You can now start building React applications by modifying the code inside the src
directory of your project.
Step by Step Tutorials for ReactJs for hello world program
To create a “Hello World” program in ReactJS, you can follow these step-by-step instructions:
Step 1: Set Up Your Development Environment – Ensure that Node.js and npm (Node Package Manager) are installed on your machine. – Open your command line interface (such as Terminal for macOS or Command Prompt for Windows).
Step 2: Create a New React Project
– Run the following command to create a new React project:
npx create-react-app hello-world
– Navigate into the project directory by running:
cd hello-world
Step 3: Modify the React Component
– Open the project directory in your preferred code editor.
– By default, a file named `App.js` will be present in the `src` folder. Open that file.
– Clear the existing code inside `App.js` and replace it with the following:
import React from 'react';
function App() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
export default App;
Step 4: Run the React App
– In the command line, still inside your project directory, run the following command to start the React development server:
npm start
Wait for the server to start, and your default web browser will open with the “Hello, World!” message displayed on `localhost:3000`.
Congratulations! You have created a basic “Hello World” program using ReactJS. You can now customize and expand your React components based on your requirements.