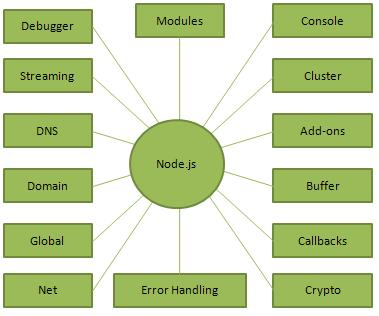
What is node.js ?
Node.js is an open-source, cross-platform, JavaScript runtime environment that runs on the V8 JavaScript engine. It allows developers to run JavaScript code outside of a web browser, enabling server-side scripting and backend development.
What is top use cases of node.js ?
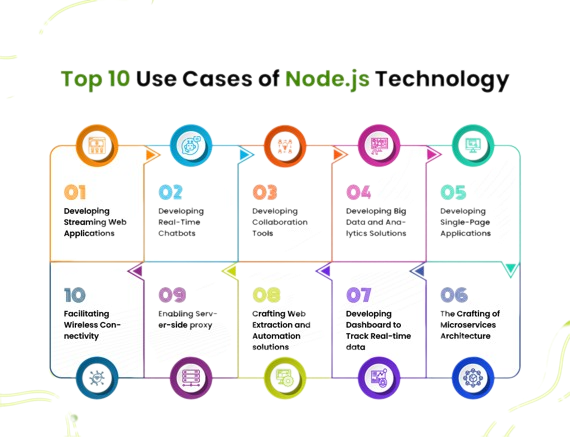
Some of the top use cases of Node.js include:
1. Building server-side web applications and APIs: Node.js provides a fast and efficient way to handle server-side logic and serve web content.
2. Real-time applications: Node.js’s event-driven architecture and non-blocking I/O make it suitable for building real-time applications such as chat applications, collaborative tools, and gaming platforms.
3. Microservices architecture: Node.js’s lightweight and scalable nature makes it ideal for developing microservices-based architectures, where individual services can be developed and deployed independently.
4. Command-line tools: Node.js offers easy and powerful access to the file system, making it a preferred choice for developing command-line tools and utilities.
What are feature of node.js ?
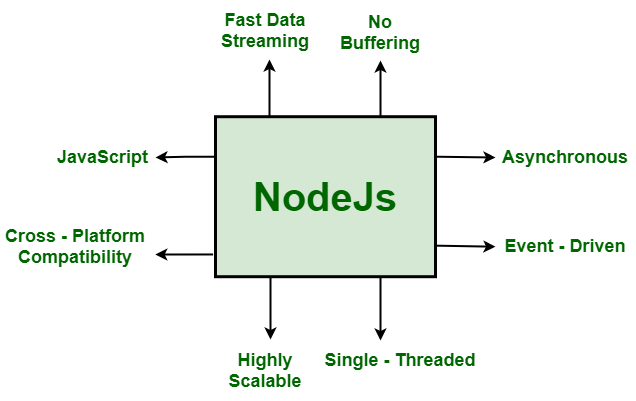
1. Asynchronous and event-driven: Node.js follows a non-blocking, asynchronous programming model, allowing it to handle concurrent requests efficiently.
2. Fast and scalable: Node.js uses Google’s V8 engine, which compiles JavaScript into machine code, resulting in fast execution. It also employs an event-driven, single-threaded architecture that can handle high traffic and thousands of concurrent connections.
3. NPM ecosystem: Node.js comes with npm (Node Package Manager), a vast repository of open-source libraries and modules that can be easily integrated into your projects.
What is the workflow of node.js ?
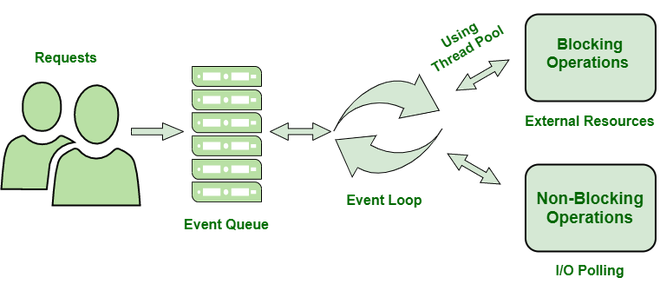
- Request Handling: When a client sends a request to a Node.js server, the server creates an event for that request and adds it to the event loop.
- Event Loop: The event loop is responsible for managing all the events in Node.js. It continuously checks for new events and processes them one by one.
- Non-Blocking I/O: Node.js uses non-blocking I/O operations, which means it can handle multiple I/O operations concurrently without blocking the execution of other code.
- Callbacks: Asynchronous operations in Node.js are handled using callbacks. When an asynchronous operation is completed, a callback function is called.
- Response Handling: Once the server has processed the request and generated a response, it sends the response back to the client.
How node.js Works & Architecture?
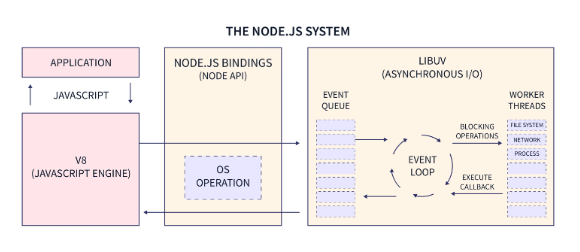
Node.js is an event-driven, non-blocking I/O framework that runs on the V8 JavaScript engine. It is designed to build scalable network applications.
Here is how Node.js works:
- A client makes a request to the Node.js server.
- The Node.js server receives the request and puts it in the event queue.
- The event loop wakes up and checks the event queue for any requests.
- If there is a request in the event queue, the event loop executes the appropriate code to handle the request.
- Once the request has been handled, the event loop goes back to sleep.
The Node.js architecture is based on the following components:
- Event loop: The event loop is responsible for handling all of the requests that come into the Node.js server. It is a single-threaded loop that wakes up when there is a request in the event queue.
- Event queue: The event queue is a queue of requests that are waiting to be handled by the event loop.
- Callbacks: Callbacks are functions that are executed when an event occurs. In Node.js, all of the code that handles requests is executed in the form of callbacks.
- Modules: Modules are JavaScript files that contain code that can be used in Node.js applications. Modules are loaded and executed by the Node.js runtime.
The Node.js architecture is designed to be efficient for handling a large number of concurrent requests. The single-threaded event loop ensures that only one request is processed at a time, but the event loop can switch between requests very quickly. This makes Node.js well-suited for building real-time applications.
The non-blocking I/O in Node.js means that multiple requests can be handled at the same time without blocking. This is done by using callbacks. When a request is made to the Node.js server, the callback for that request is placed in the event queue. The event loop then wakes up and executes the callback for the next request in the queue. This allows Node.js to handle multiple requests at the same time without blocking.
The combination of the single-threaded event loop and non-blocking I/O makes Node.js a very efficient platform for building scalable network applications.
How to Install and Configure node.js ?
To install Node.js, follow these steps:
- Visit the official Node.js website (https://nodejs.org/).
- Download the installer for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the installation wizard’s instructions.
- After installation, verify the installation by opening a terminal (or Command Prompt on Windows) and running
node -v
andnpm -v
. This should display the installed Node.js and npm versions.
Step by Step Tutorials for node.js for hello world program
Step-by-Step Tutorial for a Hello World program in Node.js:
- Create a Project Directory: Create a new folder for your Node.js project.
- Initialize the Project: Open a terminal in the project directory and run the following command to initialize the project and create a
package.json
file:
npm init
- Install Express: For this tutorial, let’s use Express, a popular Node.js web framework. Install it using npm:
npm install express
- Create the Application: Create a file named
app.js
in your project directory and add the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
- Run the Application: Save the file and run the application using the following command:
node app.js
- Test the Application: Open your web browser and go to
http://localhost:3000
. You should see the message “Hello World!” displayed on the page.
Congratulations! You’ve created a basic Hello World program using Node.js and Express.