What is ECMAScript 6 (or ES6)
ECMAScript 2015 (or ES6) is the sixth and major edition of the ECMAScript language specification standard. It defines the standard for the JavaScript implementation.
ES6 brought significant changes to the JavaScript language. It introduces several new features such as, block-scoped variables, new loop for iterating over arrays and objects, template literals, and many other enhancements to make JavaScript programming easier and more fun. In this chapter, we will discuss some of the best ES6 features that you can use in your everyday JavaScript coding.
The let
Keyword
ES6 introduces the new let
keyword for declaring variables. Prior to ES6, the only way to declare a variable in JavaScript was the var
keyword. Let’s see what’s the difference between them is.
There are two critical differences between the var
and let
. Variables declared with the var
keyword are function-scoped and hoisted at the top within its scope, whereas variables declared with let
keyword are block-scoped ({}
) and they are not hoisted.
Block scoping simply means that a new scope is created between a pair of curly brackets i.e. {}
. Therefore, if you declare a variable with the let
keyword inside a loop, it does not exist outside of the loop, as demonstrated in the following example:
As you can see in the above example the variable i
in the first block is not accessible outside the for
loop. This also enables us to reuse the same variable name multiple times as its scope is limited to the block ({}
), which results in less variable declaration and more cleaner code.
The const
Keyword
The new const
keyword makes it possible to define constants. Constants are read-only, you cannot reassign new values to them. They are also block-scoped like let
.
However, you can still change object properties or array elements:
The for...of
Loop Variable
The new for...of
loop allows us to iterate over arrays or other iterable objects very easily. Also, the code inside the loop is executed for each element of the iterable object. Here’s an example:
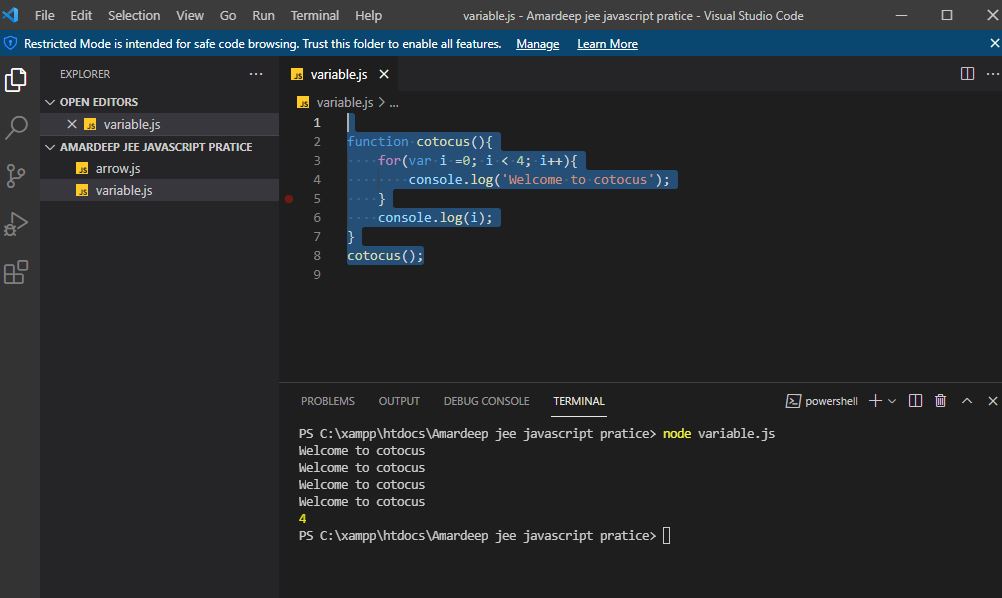
Arrow Functions
Arrow Functions are another interesting feature in ES6. It provides a more concise syntax for writing function expressions by opting out the function
and return
keywords.
Arrow functions are defined using a new syntax, the fat arrow (=>
) notation. Let’s see how it looks:
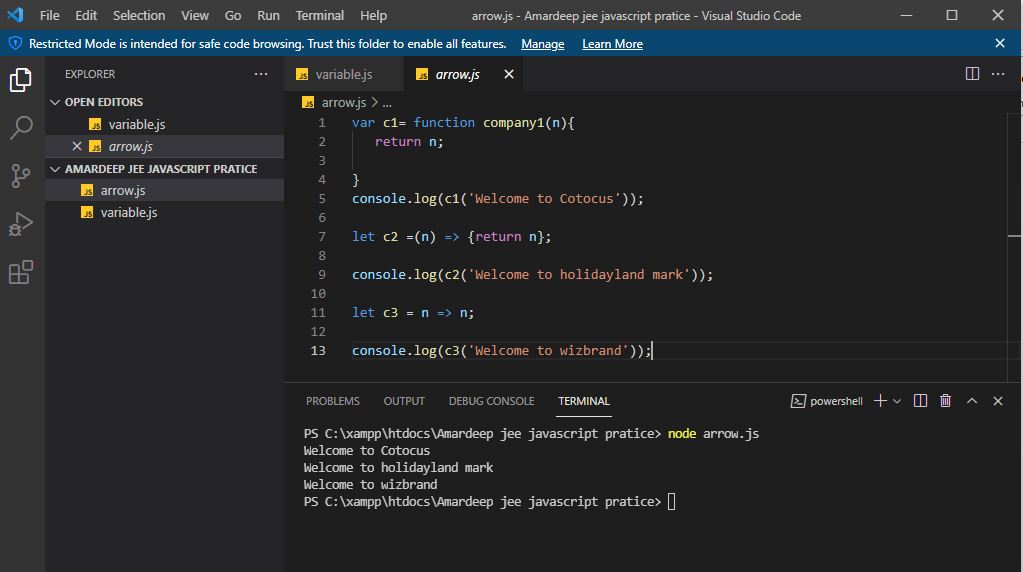