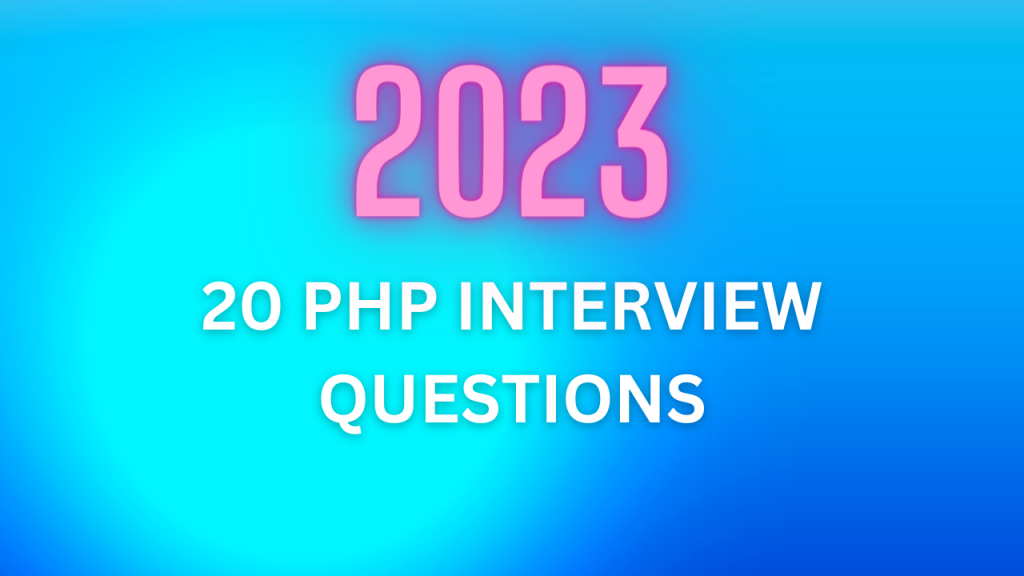
1. How to get the length of string?
To get the length of a string in PHP, you can use the strlen()
function. Here’s an example:
$string = "Hello, World!";
echo strlen($string); // Outputs: 13
This will output the number of characters in the string
2. Explain some of the PHP string functions?
There are several built-in functions in PHP to manipulate strings. Some of them include:
- strlen(): Gets the length of a string.
- strpos(): Finds the position of the first occurrence of a substring in a string.
- substr(): Returns a part of a string.
- str_replace(): Replaces some characters with some other characters in a string.
- strtolower(): Makes a string lowercase.
- strtoupper(): Makes a string uppercase.
- ucfirst(): Makes the first character of a string uppercase.
- ltrim(): Removes whitespace or other predefined characters from the left side of a string.
3. What are the methods to submit form in PHP?
Forms in PHP can be submitted using either the GET or POST method. The method is defined in the form tag’s method attribute. Here’s an example:
<form action="welcome.php" method="post">
Name: <input type="text" name="name"><br>
E-mail: <input type="text" name="email"><br>
<input type="submit">
</form>
In this case, the form data will be sent to the welcome.php page using the POST method. You can then access these values in PHP using the $_POST superglobal array
4. How can you submit a form without a submit button?
To submit a form without a submit button, you can use JavaScript or jQuery to trigger the form submission programmatically. For example, using JavaScript:
document.forms["myForm"].submit();
Here’s an example using jQuery:
$(document).ready(function(){
$('#yourFormId').submit();
});
5. What are the ways to include file in PHP?
There are several ways to include files in PHP:
- include: This statement includes and evaluates the specified file during the execution of the script.
- require: This statement includes and evaluates the specified file. If the file cannot be included, require will cause a fatal error and halt the script.
- include_once: This statement checks if the file has already been included, and if so, it does not include it again.
- require_once: This statement checks if the file has already been included, and if so, it does not include it again. If the file cannot be included, require_once will cause a fatal error and halt the script.
6. Differentiate between require and include?
The main difference between require
and include
is that require
will stop the script and generate a fatal error if the file cannot be found, while include
will only generate a warning and allow the script to continue.
7. Explain setcookie() function in PHP?
The setcookie() function is used to create a cookie. A cookie is a small file stored on the user’s computer by the web browser while browsing a website. Here’s an example:
setcookie("TestCookie", "Hello, World!", time() + (86400 * 30), "/");
This code creates a cookie named TestCookie, with the value Hello, World!. The cookie will expire after 30 days (86400 seconds * 30). The cookie will be accessible on the whole website (/).
8. How can you retrieve a cookie value?
To retrieve a cookie value in PHP, you can use the $_COOKIE
superglobal array. For example:
if (isset($_COOKIE['myCookie'])) {
$value = $_COOKIE['myCookie'];
echo $value;
}
9. What is a session?
Sessions are a server-side mechanism for storing user-specific data across multiple page requests.
Data is stored on the server, typically in files or a database.
10. What is the method to register a variable into a session?
In PHP, you can register a variable into a session using the $_SESSION superglobal array. Here is an example:
<?php
// Starting session
session_start();
// Storing session data
$_SESSION["username"] = "Maruti Kr";
?>
In this example, a session is started with session_start(), and then a session variable named “username” is registered with the value “Maruti Kr”.
11. What is $_SESSION in PHP?
$_SESSION
is a superglobal array in PHP that is used to store session variables. It allows you to access and manipulate session data throughout the application.
12. What is PHP session_start() and session_destroy() function?
session_start() is a function in PHP that starts a new session or resumes the existing one. It must be called before any output is sent to the browser.
session_destroy() is a function in PHP that destroys all of the data associated with the current session. It does not unset any of the global variables associated with the session, or unset the session cookie.
13. What is the difference between session and cookie?
The main difference between a session and a cookie is that sessions are stored on the server, while cookies are stored on the client’s computer. Sessions are more secure for storing sensitive data, while cookies are generally used for lightweight data storage or user preferences.
14. Write syntax to open a file in PHP?
To open a file in PHP, you can use the fopen()
function. Here’s an example:
<?php
$file = fopen("welcome.txt", "r");
?>
In this example, the file “welcome.txt” is opened in read mode (“r”).
15. How to read a file in PHP?
To read a file in PHP, you can use functions like fgets()
or fread()
in combination with a loop. Here’s an example using fgets()
:
$file = fopen("myfile.txt", "r");
while(!feof($file)) {
$line = fgets($file);
echo $line;
}
fclose($file);
16. How to write in a file in PHP?
You can write in a file using the fwrite() function. Here is an example:
<?php
$file = fopen("welcome.txt", "w");
fwrite($file, "Hello, World!");
fclose($file);
?>
17. How to delete file in PHP?
You can delete a file in PHP using the unlink() function. Here is an example:
<?php
unlink("welcome.txt");
?>
In this example, the file “welcome.txt” is deleted.
18. What is the method to execute a PHP script from the command line?
To execute a PHP script from the command line, you can follow these steps:
- Open the command prompt or terminal on your computer.
- Navigate to the directory where your PHP script is located using the
cd
command. - Once you are in the correct directory, type the following command to execute the PHP script:
php script_name.php
(replacescript_name.php
with the actual name of your PHP file). - Press Enter, and the PHP script will be executed, and the output, if any, will be displayed in the command prompt or terminal.
19. How to upload file in PHP?
To upload a file in PHP, you can use the following steps:
- Create an HTML form with the
enctype
attribute set to"multipart/form-data"
to allow file uploads. - Specify an
<input>
element with thetype
attribute set to"file"
. This will allow users to select a file to upload. - In the PHP script that processes the form data, use the
$_FILES
superglobal variable to access the uploaded file. You can retrieve file information like name, size, and temporary storage location from this variable. - Move the uploaded file from its temporary location to a permanent location on your server using the
move_uploaded_file()
function.
20. How to download file in PHP?
To download a file in PHP, you can use the following steps:
- Set the appropriate headers to indicate that you are sending a file download, including the file name, file type, and content length.
- Open the file using functions like
fopen()
,readfile()
, orfile_get_contents()
. - Send the file contents to the client using functions like
echo
orfwrite()
. - Flush the output buffer and exit the script using
flush()
andexit()
ordie()
.