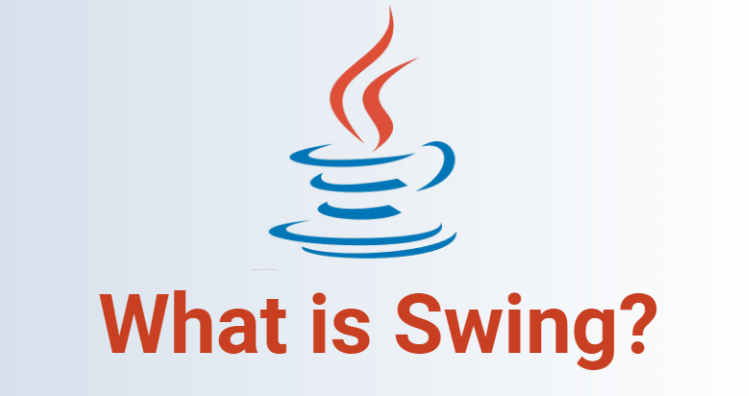
What is Swing?
Swing is a Java Foundation Classes (JFC) library and an extension of the Abstract Window Toolkit (AWT). It offers improved functionality over AWT, including new components, expanded component features, and excellent event handling with drag-and-drop support. Swing is part of the standard Java distribution and is used to build standalone GUI apps, servlets, and applets. It is more portable and flexible than AWT, and its components are platform-independent and lightweight.
What is top use cases of Swing?
Some of the top use cases of Swing are:
- Creating desktop applications: Swing is commonly used to develop desktop applications in various fields, such as finance, healthcare, and education.
- Building UI components: Developers can utilize Swing to design and implement interactive and visually appealing user interface components.
- Game development: Swing can be used to build simple games that require GUI elements and user interaction.
- Data visualization: Swing provides the capability to display and interact with charts, graphs, and other visual representations of data.
What are feature of Swing?
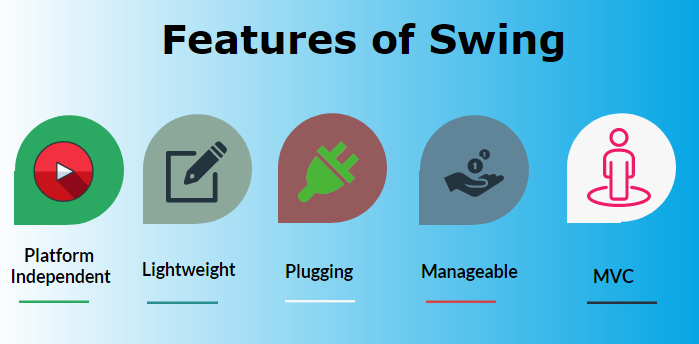
Some features of Swing include:
- Lightweight: Swing is built on top of the Java Foundation Classes (JFC) and is designed to be platform-independent and resource-efficient.
- Customizable: Swing components are highly customizable, allowing developers to change their appearance and behavior to meet specific requirements.
- Layout managers: Swing provides a variety of layout managers that help in arranging components within containers.
- Event-driven programming: Swing is based on an event-driven model, where actions performed by users trigger events that are processed by event listeners.
What is the workflow of Swing?
The workflow of Swing typically involves the following steps:
- Building the GUI: Design and create the user interface of the application by adding Swing components to containers like JFrame, JDialog, or JPanel.
- Event handling: Implement event listeners to handle user actions, such as button clicks or menu selections.
- Data processing: Write the necessary code to process or manipulate data based on user input or interactions.
- Displaying results: Update the UI components with the processed data, displaying the outcomes to the user.
How Swing Works & Architecture?
Swing is a component-based framework in Java, and it’s built on a modified version of the Model-View-Controller (MVC) design pattern. This pattern separates an application into three parts: a model that represents the data, the view that is the visual representation of that data, and a controller that takes user input on the view and translates that to changes in the model.
In Swing, every component has its model, even the basic ones like buttons. There are two kinds of models in Swing: GUI-state models and application-data models. The GUI-state models handle the state of the component, while the application-data models handle data they work with.
Swing uses a single UI object for both the view and the controller, which is sometimes referred to as a separable model architecture. This design allows for efficient handling of data and the use of pluggable look and feel at runtime.
Swing’s architecture is rooted in the MVC design, but it has a unique twist. In Swing, the model and the UI delegate (which is responsible for painting the screen and handling GUI events) are combined into a single object. This object, known as the UI delegate, is responsible for maintaining information about how to draw the component on the screen and reacts to various events that propagate through the component.
One of the key advantages of this design is the ability to tie multiple views to a single model. For example, if you want to display the same data in a pie chart and in a table, you can base the views of two components on a single data model. This way, if the data needs to be changed, you can do so in only one placeāthe views update themselves accordingly.
Pluggable Look-and-Feel Architecture
Swing’s pluggable look-and-feel architecture allows the provision of a single component API without dictating a particular look-and-feel. The Swing toolkit provides a default set of look-and-feels, but the API is open, allowing developers to create new look-and-feel implementations by either extending an existing look-and-feel or creating one from scratch.
In a nutshell, pluggable look-and-feel design means that the portion of a component’s implementation that deals with the presentation (the look) and event-handling (the feel) is delegated to a separate UI object supplied by the currently installed look-and-feel, which can be changed at runtime.
How to Install and Configure Swing?
To install Swing, the Java GUI (Graphical User Interface) widget toolkit, you need to follow these steps:
- Download the latest version of the JDK (Java Development Kit) from the official Oracle website (https://www.oracle.com/java/technologies/javase-jdk11-downloads.html) and install it on your computer.
- Set up the JDK environment variables. Add the JDK’s bin directory path to the system’s “PATH” environment variable. This step allows you to access the Java compiler and other necessary tools from the command line.
- Create a new Java project in your preferred Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or NetBeans.
- In the project configuration settings, ensure that the JDK version matches the one you installed.
- Now, you are ready to start coding Swing applications. Add the necessary Swing libraries to your project by referencing them in your IDE. Usually, the required Swing libraries are part of the JDK installation, so you don’t need to download them separately.
- Write your Swing code following the Swing architecture and components. You can refer to various online tutorials, documentation, or books to learn more about Swing programming.
- Once you have written the code for your Swing application, compile it using your IDE’s build tools or via the command line using the Java compiler (javac).
- Finally, execute your Swing application, and you should see your graphical user interface.
Step by Step Tutorials for Swing for hello world program
Create a new Java file (e.g., HelloWorldSwing.java) and paste the following code:
import javax.swing.*;
public class HelloWorldSwing {
public static void main(String[] args) {
// Create a JFrame (main window)
JFrame frame = new JFrame("Hello, World!");
// Create a JLabel (text label)
JLabel label = new JLabel("Hello, World!");
// Add the label to the frame
frame.add(label);
// Set the frame size and make it visible
frame.pack();
frame.setVisible(true);
}
}
Explain of the Code:
- Import: javax.swing.*; imports all necessary Swing classes.
- public class HelloWorldSwing: Defines the main class.
- main() method: The entry point of the program.
- JFrame frame = new JFrame(“Hello, World!”);: Creates a new frame with the title “Hello, World!”.
- JLabel label = new JLabel(“Hello, World!”);: Creates a label that displays the text “Hello, World!”.
- frame.add(label);: Adds the label to the frame.
- frame.pack();: Packs the frame to fit the preferred size of its components.
- frame.setVisible(true);: Makes the frame visible on the screen.
Compile and Run:
Use your IDE’s “Run” button or compile the code from the command line (javac HelloWorldSwing.java && java HelloWorldSwing).
Output:
A window with the title “Hello, World!” and a label displaying “Hello, World!” should appear on your screen.
Congratulations! You’ve built your first Swing application!