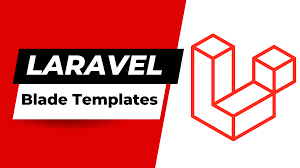
Introduction
Blade Template in Laravel is a powerful templating engine that allows you to write clean and efficient PHP code for creating views in your Laravel application. It provides a way to separate your logic (such as conditional statements and loops) from your presentation, resulting in more maintainable and reusable code.
To create layouts in Blade Template, you can follow these steps:
- Create a layout file: Start by creating a layout file that will serve as the base for your views. This file typically contains the common structure of your web pages, such as headers, footers, navigation bars, etc. Let’s name it
layout.blade.php
.
<!-- layout.blade.php -->
<html>
<head>
<title>@yield('title')</title>
</head>
<body>
<header>
<!-- Header content -->
</header>
<main>
@yield('content')
</main>
<footer>
<!-- Footer content -->
</footer>
</body>
</html>
2. Extending the Layout: Create individual views that extend this layout. Use the @extends
directive to specify the layout to inherit from and define the sections to be replaced.
<!-- home.blade.php -->
@extends('layout')
@section('title', 'Home Page')
@section('content')
<!-- Content for the home page -->
@endsection
3. Including Views: You can include partials or reusable components within your views using the @include
directive.
<!-- home.blade.php -->
@extends('layout')
@section('title', 'Home Page')
@section('content')
<!-- Content for the home page -->
@include('partials.posts') <!-- Including a partial -->
@endsection
4. Using Variables: You can pass data to views using an associative array or an object and then access these variables within the Blade templates.
<!-- home.blade.php -->
@extends('layout')
@section('title', $pageTitle)
@section('content')
<h1>{{ $welcomeMessage }}</h1>
@endsection
5. Conditional Rendering and Loops: Blade provides directives like @if
, @else
, @foreach
, and @while
for handling conditional logic and looping through data.
@if ($condition)
<!-- Display something -->
@else
<!-- Display something else -->
@endif
@foreach ($items as $item)
<p>{{ $item }}</p>
@endforeach
By following these steps, you can create a consistent layout structure for your web application and easily manage content in individual views using Laravel’s Blade templating engine.