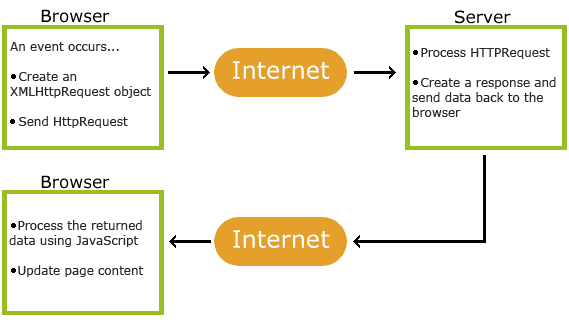
What is Ajax?
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that uses various web technologies on the client-side to create asynchronous web applications. With Ajax, web applications can send and retrieve data from a server asynchronously without interfering with the display and behaviour of the existing page.
What are the top use cases of Ajax?
The top use cases of Ajax include:
1. Dynamic content updates: Ajax allows for the updating of specific parts of a webpage without reloading the entire page. This can be used to dynamically load new data, such as in social media feeds or chat applications.
2. Form submission: Ajax can be used to submit form data to a server without reloading the entire page. This provides a more seamless user experience and avoids the need for a page refresh.
3. Autocomplete and search suggestions: Ajax can be used to provide real-time autocomplete suggestions as users type in a search query, which enhances user experience and speeds up the search process.
4. Real-time data updates: Ajax can be used to retrieve and display real-time data from a server, such as stock quotes or live sports scores.
5. Interactive maps: Ajax can be used to update map data dynamically based on user interactions, such as zooming or panning.
What are the features of Ajax?
Key features of Ajax include:
1. Asynchronous communication: Ajax allows for asynchronous communication between the client and server, meaning that data can be retrieved or submitted without disrupting the user’s interaction with the page.
2. XMLHttpRequest: Ajax uses the XMLHttpRequest object to send and receive data between the client and server.
3. DOM manipulation: Ajax allows for the manipulation of the Document Object Model (DOM) of a webpage, enabling dynamic updates to specific elements on the page.
What is the workflow of Ajax?
- User triggers an event on the web page, such as clicking a button or submitting a form.
- JavaScript code running in the browser sends an XMLHttpRequest to the server.
- The server processes the request and sends back a response.
- JavaScript code in the browser receives the response and updates the web page accordingly, without reloading the entire page.
How Ajax Works & Architecture?
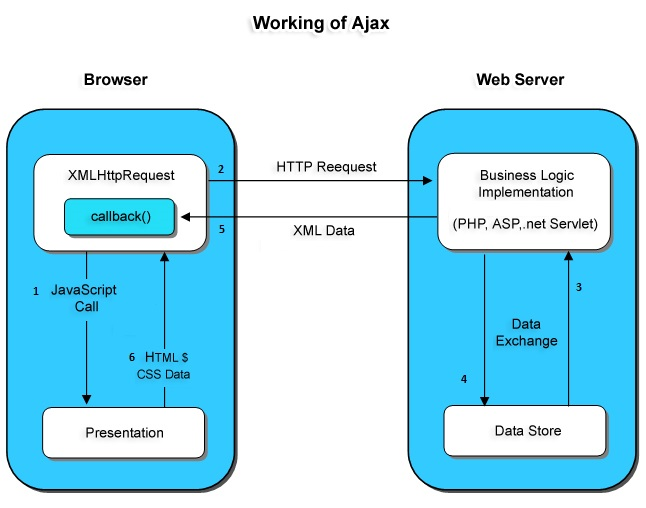
Ajax works by using the following technologies:
- XMLHttpRequest object: The XMLHttpRequest object is a built-in JavaScript object that allows web applications to communicate with the server asynchronously.
- JavaScript: JavaScript is a scripting language that is used to create dynamic web pages.
- HTML DOM: The HTML DOM is a model of a web page that allows JavaScript to interact with the elements of the page.
- XML: XML is a markup language that is used to represent data.
The architecture of Ajax is as follows:
- The client-side: The client-side is the web browser that the user is using. The JavaScript code on the client-side is responsible for sending the requests to the server and updating the content of the web page based on the responses from the server.
- The server-side: The server-side is the computer that hosts the web application. The server-side code is responsible for processing the requests from the client-side and sending the responses back to the client-side.
How to Install and Configure Ajax?
Ajax does not need to be installed separately. It is a built-in feature of most web browsers. However, you may need to configure your web browser to allow JavaScript to run.
To configure your web browser to allow JavaScript to run, follow these steps:
- Open your web browser.
- Click on the “Settings” or “Options” menu.
- Click on the “Security” or “Privacy” tab.
- Find the section that allows JavaScript to run.
- Make sure that the option to allow JavaScript is enabled.
Step by Step Tutorials for Ajax for the Hello world program
Here is a simple “Hello World” program example using Ajax with jQuery:
1. Include jQuery: Import jQuery library in your HTML file by adding the following script tag to your HTML head:
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js">
</script>
2. HTML Markup: Create a button and a container to display the Ajax response. For example:
<button id="helloButton">Click me</button>
<div id="responseContainer"></div>
3. JavaScript/jQuery code: Below the HTML markup, add the following JavaScript/jQuery code to handle the Ajax request on button click and update the response container:
$(document).ready(function() {
$('#helloButton').click(function() {
$.ajax({
url: 'hello-world.php', // Server-side script to handle the request
method: 'POST', // Request method (can be GET, POST, etc.)
success: function(response) {
$('#responseContainer').text(response); // Update the response container with the received data
}
});
});
});
4. Server-side script: Create a server-side script (e.g., PHP) to handle the Ajax request. For example, in a file named “hello-world.php”:
<?php
echo "Hello, World!";
?>
Ensure that your server is properly configured to execute the server-side script.
When the “Click me” button is clicked, an Ajax request will be sent to the server-side script, which will respond with the text “Hello, World!”. The response will then be displayed in the response container on the webpage without refreshing the entire page.
[…] What is Ajax and How Ajax Works & Architecture? […]