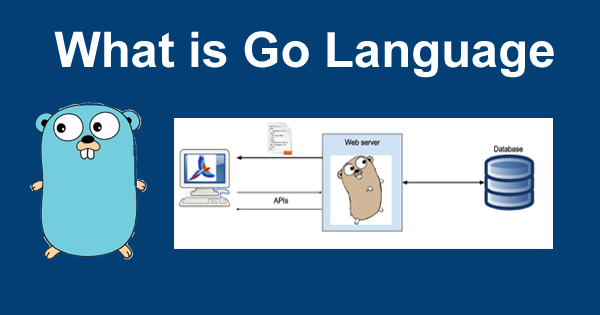
What is Go?
Go, also known as Golang, is an open-source programming language developed by Google in 2007. It was officially released in 2009. Go is known for its simplicity, efficiency, and ease of learning, making it a popular choice for building scalable network services, web applications, and command-line tools
What is top use cases of Go?
Here are some of the top use cases:
- Web Development: Go can be used as a backend server for web applications. It’s particularly effective for building RESTful APIs.
- Network Services: Go’s strong support for concurrency makes it well-suited for building high-performance network services.
- Command-Line Tools: Go’s simplicity and efficiency make it a great choice for creating command-line tools.
- Distributed Systems: Go’s support for concurrency and garbage collection makes it suitable for building large-scale distributed systems.
- DevOps Tools: Go has been used to build tools like Docker, Kubernetes, and the Heroku CLI.
What are feature of Go?
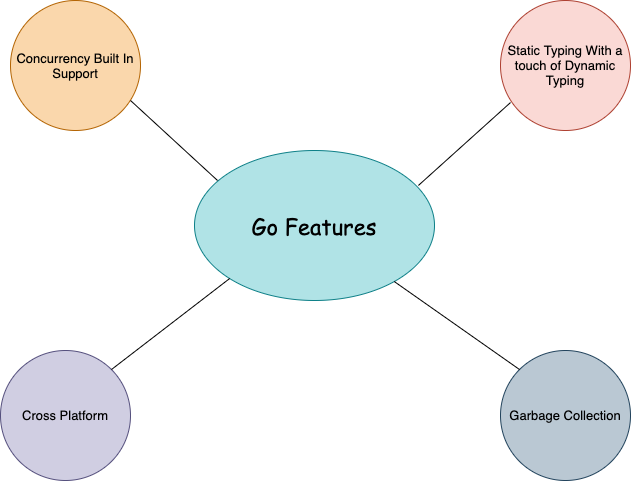
Some key features of Go include:
- Concurrency: Go has built-in support for concurrency through goroutines (lightweight threads) and channels, making it easy to write concurrent programs.
- Garbage collection: Go includes an automatic garbage collector that manages memory allocation and deallocation, reducing manual memory management overhead.
- Static typing: Go is statically typed, which provides better type safety and helps catch errors at compile-time rather than runtime.
- Simplicity: Go’s syntax is designed to be simple and easy to read, reducing cognitive load and improving developer productivity.
What is the workflow of Go?
The workflow of Go typically involves the following steps:
- Write code: Use a text editor or integrated development environment (IDE) to write your Go code.
- Compile: Use the Go compiler (go build) or command line interface (go run) to compile your code into an executable binary.
- Test: Use the testing framework provided by Go to write and execute test cases for your code.
- Build and package: Use the go build command to build your project into a binary or go install to compile and install it into your Go environment.
How Go Works & Architecture?
Go is a statically typed language, meaning that types are checked at compile time. It has built-in garbage collection, which automatically manages memory for you. This eliminates the need for manual memory management, reducing the likelihood of memory leaks and other bugs that can arise from manual memory management.
Go also has a rich standard library, which provides support for a wide range of functionality, including networking, encryption, and file handling.
How to Install and Configure Go?
To install Go (also known as Golang), you can follow these steps:
- Visit the official Golang website at https://golang.org/dl/ and go to the downloads page.
- Choose the appropriate download package for your operating system (Windows, macOS, or Linux). Go supports a wide range of platforms, so make sure to select the correct one.
- Once the download is complete, locate the downloaded package and run the installer.
- Follow the installation wizard instructions, accepting the default configurations unless you have specific customization requirements.
- After the installation is complete, open a terminal or command prompt window on your computer.
- To verify the installation, type the following command:
go version
If Go has been installed correctly, you should see the version number displayed in the terminal window.
7. Set up your Go workspace by creating a directory where you’ll keep your Go projects. This directory is commonly referred to as the “GOPATH.” For example, you can create a directory called “go” in your home directory:
mkdir go
8. Set the GOPATH environment variable to the path of your Go workspace directory. You can do this by adding the following line to your shell’s configuration file (e.g., .bashrc
for Linux or macOS, or .bash_profile
for macOS):
export GOPATH=$HOME/go
Make sure to replace $HOME/go
with the actual path to your Go workspace directory.
9. Restart your terminal or command prompt to apply the changes to the environment variables.
Step by Step Tutorials for Go for hello world program
Once you have Go installed and configured, you can follow these steps to write a “Hello, World!” program:
- Open a text editor and create a new file called “hello.go”.
- In the file, write the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
- Save the file.
- Open a command prompt or terminal and navigate to the directory where you saved the “hello.go” file.
- Run the program using the command:
go run hello.go
You should see the output “Hello, World!” printed to the console.
Note: Make sure you have Go installed and properly configured before attempting to run Go programs.