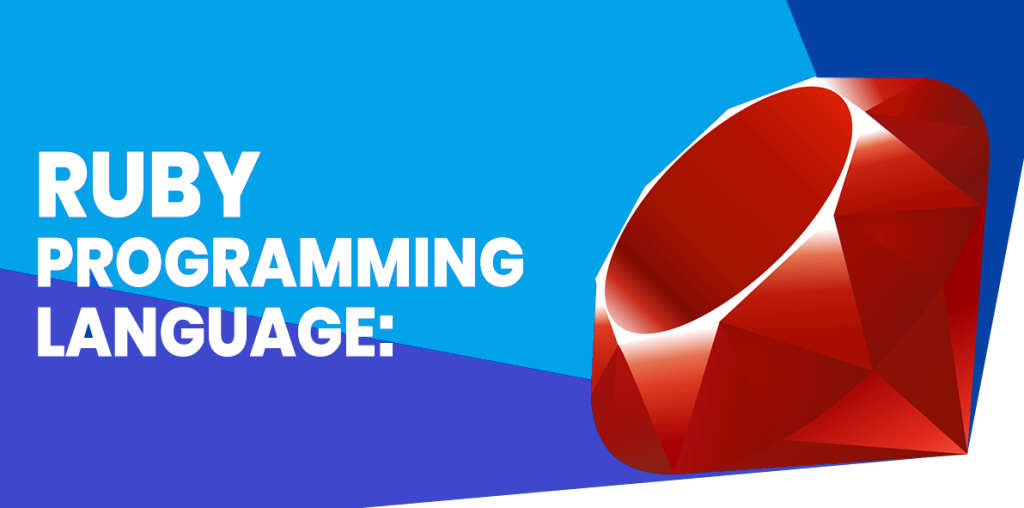
What is Ruby?
Ruby is an interpreted, high-level, general-purpose programming language. It was designed with an emphasis on programming productivity and simplicity. In Ruby, everything is an object, including primitive data types. It was developed in the mid-1990s by Yukihiro “Matz” Matsumoto in Japan.
Top Use Cases of Ruby
Some of the top use cases for Ruby include:
- Web development: Ruby is a popular choice for web development, thanks to its expressive syntax and its large ecosystem of libraries and frameworks. Some popular Ruby web frameworks include Rails, Sinatra, and Hanami.
- Command-line tools: Ruby is a good choice for writing command-line tools, as it is concise and easy to read.
- Data science: Ruby is a popular choice for data science, as it has a number of libraries for data manipulation and analysis.
- Game development: Ruby is a good choice for game development, as it is fast and efficient.
- System administration: Ruby can be used for system administration tasks, such as writing scripts and automating tasks.
Features of Ruby
Ruby offers several features that make it a powerful and flexible programming language. Some of the key features of Ruby include:
- Object-Oriented: Ruby is a fully object-oriented language, which means everything in Ruby is an object. This allows for clean and modular code organization.
- Dynamic Typing: Ruby is dynamically typed, which means you don’t need to specify variable types. This makes Ruby code more flexible and easier to read.
- Garbage Collection: Ruby has automatic memory management through its garbage collection system. This helps developers focus on writing code without worrying about memory allocation and deallocation.
- Elegant syntax: Ruby has an elegant syntax that is easy to read and write.
- Large ecosystem of libraries: Ruby has a large ecosystem of libraries, which makes it a versatile language.
Workflow of Ruby
The typical workflow of Ruby involves the following steps:
- Development: Write Ruby code using a text editor or an integrated development environment (IDE). Ruby code files typically have the “.rb” extension.
- Testing: Use a testing framework like RSpec or MiniTest to write tests for your Ruby code. Testing is an essential part of the development process to ensure the correctness and reliability of the code.
- Debugging: If you encounter any errors or issues in your code, you can use a debugger like Pry or Byebug to step through the code and identify the problem.
- Version Control: Use a version control system like Git to track changes to your code and collaborate with other developers. This helps in managing code changes, branching, and merging.
- Deployment: Deploy your Ruby application to a production environment, such as a web server or a cloud platform. This involves configuring the necessary dependencies, setting up the database, and ensuring the application runs smoothly.
How Ruby Works & Architecture
Ruby is an interpreted language, which means that the Ruby code is converted into machine code at runtime. This makes Ruby slower than compiled languages, but it also makes Ruby more flexible and easier to debug.
The Ruby interpreter is written in C, and it is responsible for interpreting and executing Ruby code. The Ruby interpreter also provides a number of features, such as garbage collection and syntax highlighting.
The Ruby architecture is based on the Model-View-Controller (MVC) pattern. The MVC pattern divides an application into three components:
- The model: The model represents the data of the application.
- The view: The view displays the data to the user.
- The controller: The controller handles the user interaction.
The MVC pattern is a popular design pattern for web applications, as it helps to keep the code organized and maintainable.
Here is a diagram of the Ruby architecture:
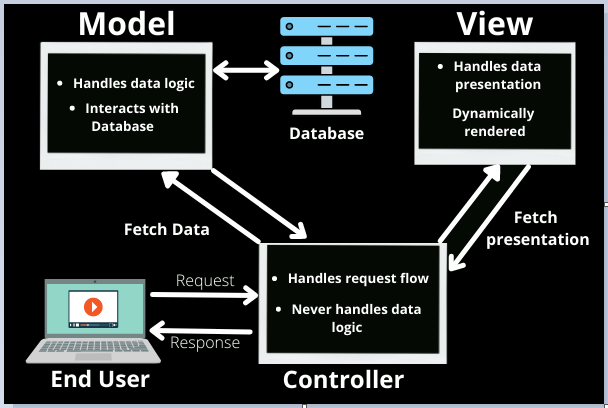
The model is responsible for storing and retrieving data. The view is responsible for displaying the data to the user. The controller is responsible for handling the user interaction.
The Ruby interpreter communicates with the model, view, and controller through a set of APIs. These APIs provide a way for the interpreter to access the data, display the data, and handle the user interaction.
The Ruby architecture is flexible and scalable, which makes it a good choice for a variety of applications. It is also easy to learn and use, which makes it a popular choice for beginners.
How to Install and Configure Ruby
To install Ruby on your machine, follow these steps:
- Visit the official Ruby website (https://www.ruby-lang.org) and download the latest stable version of Ruby for your operating system.
- Run the installer and follow the on-screen instructions to complete the installation process.
- Once Ruby is installed, open a terminal or command prompt and type “ruby -v” to verify the installation. You should see the version number of Ruby displayed.
- Ruby doesn’t require any additional configuration by default. However, you can customize the Ruby environment by setting environment variables or installing additional gems (Ruby libraries).
Step by Step Tutorials for Ruby: Hello World Program
Here is a step-by-step tutorial for writing a “Hello World” program in Ruby:
- Open a text editor and create a new file with the “.rb” extension.
- In the file, type the following code:
puts "Hello, World!"
- Save the file and open a terminal or command prompt.
- Navigate to the directory where you saved the Ruby file.
- Run the Ruby file by typing “ruby filename.rb” (replace “filename” with the actual name of your file).
- The program will output “Hello, World!” to the console.
Congratulations! You have successfully written and executed your first Ruby program.