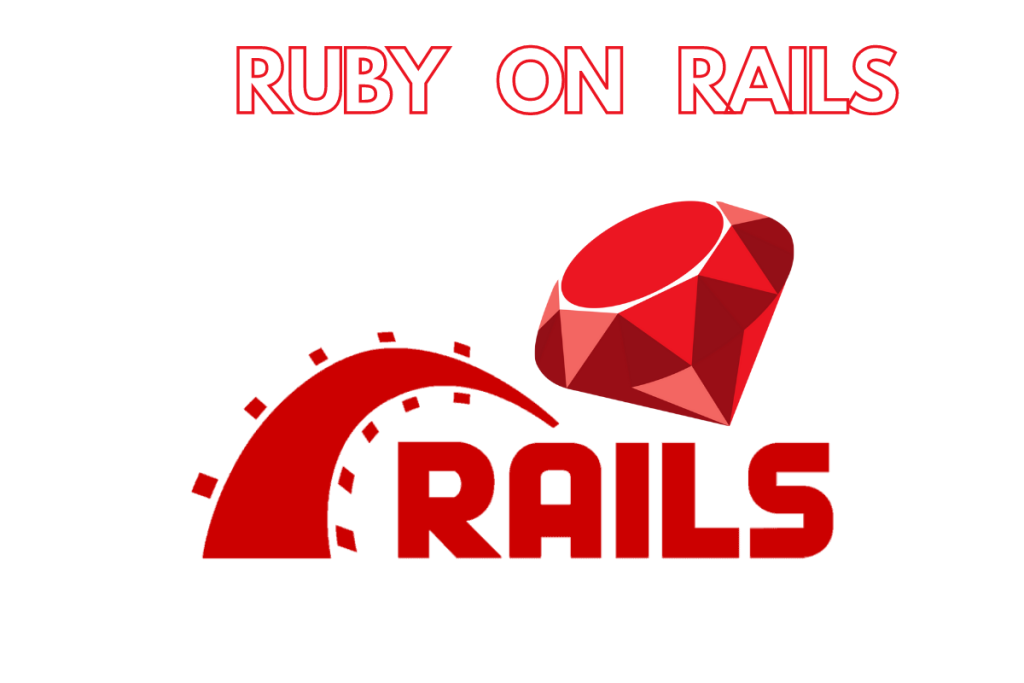
What is ruby-on-rails ?
Ruby on Rails, also known as Rails, is a popular open-source web application framework written in the Ruby programming language. It follows the Model-View-Controller (MVC) architectural pattern, which promotes the separation of concerns and allows for efficient and organized development.
What is top use cases of ruby-on-rails ?
Here are some of the top use cases of Ruby on Rails:
- Ecommerce websites: Ruby on Rails is a great choice for building ecommerce websites because it is fast and scalable. It also has a number of built-in features that make it easy to add products, categories, and shopping carts to your website.
- Content management systems: Ruby on Rails is a good choice for building content management systems (CMS) because it is easy to use and maintain. It also has a number of built-in features that make it easy to add content, pages, and users to your CMS.
- Social networking websites: Ruby on Rails is a good choice for building social networking websites because it is scalable and easy to add new features. It also has a number of built-in features that make it easy to add users, profiles, and friends to your social networking website.
- APIs: Ruby on Rails is a good choice for building APIs because it is fast and efficient. It also has a number of built-in features that make it easy to create and consume APIs.
What are feature of ruby-on-rails ?
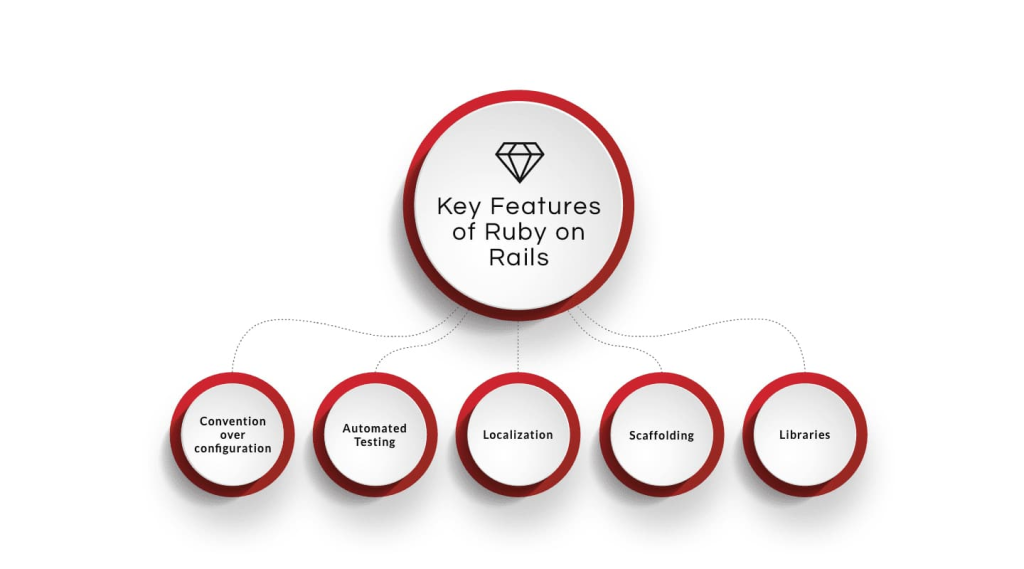
Some features of Ruby on Rails include: –
- Convention over configuration: Rails provides sensible defaults and conventions, reducing the need for explicit configurations.
2. MVC architecture: It separates the application logic into Models, Views, and Controllers, promoting clean code organization and separation of concerns.
3. Database abstraction: Rails supports various databases and provides an ORM (Object-Relational Mapping) layer called ActiveRecord.
4. Extensive library ecosystem: Rails has a rich ecosystem of plugins and gems that facilitate rapid development.
5. Testing framework: Rails has built-in support for unit testing, integration testing, and behavior-driven development.
What is the workflow of ruby-on-rails?
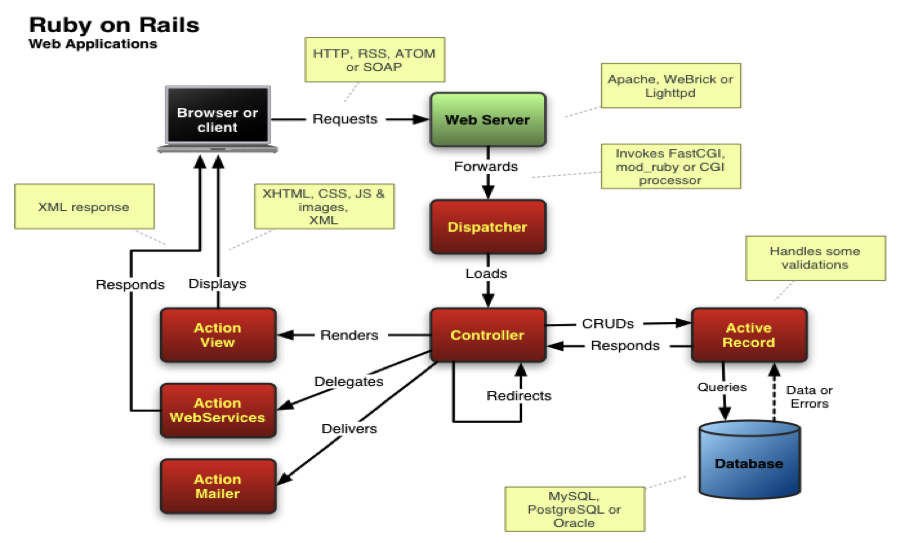
The typical workflow of Ruby on Rails involves the following steps:
- Planning: Define the requirements of the application and create a high-level overview of the desired functionality.
- Modeling: Design the database schema and define the models that represent the data structures.
- Controller and Routing: Create controllers to handle user requests, define routes to map URLs to controller actions.
- Views and Templates: Design the user interface using views and templates. Views display the data to the user, while templates provide the structure and layout.
- Testing: Write tests to ensure the functionality of the application and catch any potential issues.
- Deployment: Deploy the application to a web server or cloud platform to make it accessible to users.
How ruby-on-rails Works & Architecture?
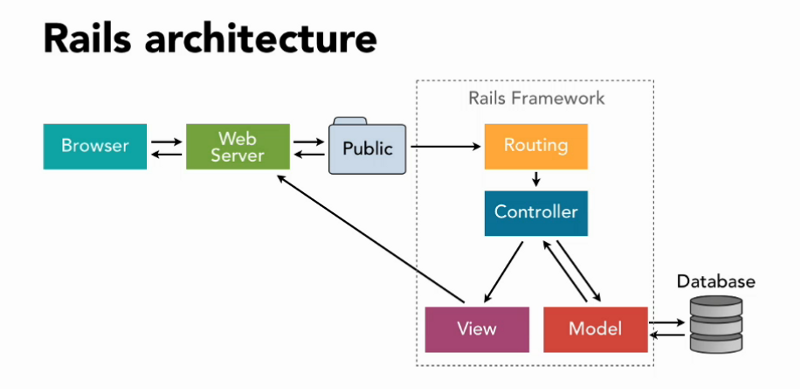
The workflow of Ruby on Rails is as follows:
- Create a new Rails application. This can be done by running the following command:
rails new my_app
This will create a new directory called my_app
with all the necessary files for a Rails application.
- Generate the necessary models, views, and controllers. This can be done by running the following command:
rails generate model User name:string email:string password:string
rails generate controller Users index show
This will generate a model called User
and a controller called Users
. The User
model will have three fields: name
, email
, and password
. The Users
controller will have two actions: index
and show
.
- Write the code for your models, views, and controllers. This is where you will put the logic for your application. For example, you might write code in the
User
model to save users to the database, or you might write code in theUsers
controller to render a list of all users or a single user. - Test your application. It is important to test your application regularly to make sure it is working properly. You can use the RSpec testing framework to write tests for your Rails application.
- Deploy your application to production. Once your application is working properly, you can deploy it to production. This means making it available to the public. You can deploy your Rails application to a hosting service like Heroku or AWS Elastic Beanstalk.
How to Install and Configure ruby-on-rails?
To install and configure Ruby on Rails, you can follow the steps below:
1. Install Ruby:
– Visit the Ruby downloads page (https://www.ruby-lang.org/en/downloads/) and download the latest stable version of Ruby.
– Follow the installation instructions for your operating system (Windows, macOS, or Linux) provided on the download page.
2. Install Rails:
– Open a terminal or command prompt and enter the following command:
gem install rails
– This command will install the latest version of Rails along with its dependencies.
3. Verify Rails Installation:
– Once the installation is complete, you can verify Rails installation by entering the following command:
rails --version
– You should see the Rails version number if the installation was successful.
4. Create a New Rails Application:
– To create a new Rails application, navigate to the directory where you want to create your project using the terminal/command prompt.
– Enter the following command to create a new Rails application:
rails new your_app_name
– Replace “your_app_name” with the desired name of your application. This command will create a new directory with the same name as your application.
5. Configure the Database:
– By default, Rails uses SQLite as its database, which is suitable for development purposes. If you plan to use a different database, you’ll need to configure it. Open the `config/database.yml` file in your Rails application directory and modify the settings under the desired environment (development, test, or production).
6. Initialize the Database:
– In the terminal/command prompt, navigate to your Rails application directory.
– Enter the following command to create the database:
rails db:create
7. Start the Server:
– To start the Rails server, enter the following command:
rails server
– The server will start running on `http://localhost:3000`, and you can access your Rails application on your web browser.
That’s it! You have successfully installed and configured Ruby on Rails.
Step by Step Tutorials for ruby-on-rails for hello world program
Here’s a step-by-step tutorial to create a “Hello, World!” program using Ruby on Rails:
Step 1: Create a New Rails Application Open your terminal and navigate to the directory where you want to create your Rails application. Then, run the following command to create a new Rails app:
rails new HelloWorldApp
This will generate a new Rails application named “HelloWorldApp.”
Step 2: Create a Controller and Action Navigate into the newly created app directory:
cd HelloWorldApp
Now, generate a new controller and action using the following command:
rails generate controller Welcome hello
Step 3: Define the Route Open the file ‘config/routes.rb
‘ in your text editor and add the following line to define a route for the “hello” action:
get 'welcome/hello'
Step 4: Create a View Navigate to the directory ‘app/views/welcome
‘ and create a new file named hello.html.erb
. In this file, add the following content:
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello, Rails!</h1>
</body>
</html>
Step 5: Start the Server Return to your terminal and navigate to the root directory of your Rails app (HelloWorldApp
). Start the Rails server with the following command:
rails server
Your Rails server should now be running locally.
Step 6: Access the “Hello World” Page Open your web browser and visit the following URL:
http://localhost:3000/welcome/hello
You should see the “Hello, Rails!” message displayed on the page.
Congratulations! You’ve successfully created a “Hello World” program using Ruby on Rails.
Ruby on Rails (RoR) is a web framework that uses the Ruby programming language. It is a popular choice for developing web applications because it is relatively easy to learn and use, and it is known for its speed and scalability.
Some of the use cases of Ruby on Rails include:
Overall, Ruby on Rails is a versatile web framework that can be used to develop a wide variety of applications. It is a good choice for developers who want to create fast, scalable, and user-friendly applications.
Here are some of the benefits of using Ruby on Rails:
write my essay
What is ruby-on-rails and How ruby-on-rails Works & Architecture? – Artificial Intelligence