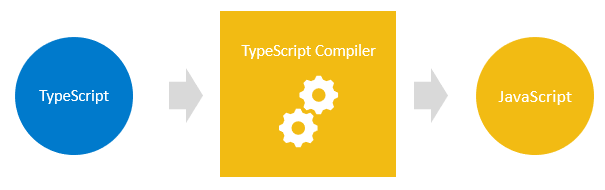
What is Typescript?
Typescript is a programming language developed by Microsoft that is a superset of JavaScript. It adds static typing capabilities to JavaScript, allowing developers to catch errors and improve code quality during development. TypeScript ultimately compiles down to plain JavaScript code.
What are the top use cases of Typescript?
TypeScript is a versatile language that can be used for a variety of purposes. Some of the top use cases of TypeScript include:
- Web development: TypeScript is a popular choice for developing web applications, as it can help to improve the maintainability and performance of code.
- Server-side development: TypeScript can also be used for server-side development, such as Node.js applications.
- Mobile development: TypeScript can be used to develop mobile applications for both Android and iOS.
- Desktop development: TypeScript can also be used to develop desktop applications.
- Game development: TypeScript is a popular choice for game development, as it can help to improve the performance and maintainability of code.
What are the features of Typescript?
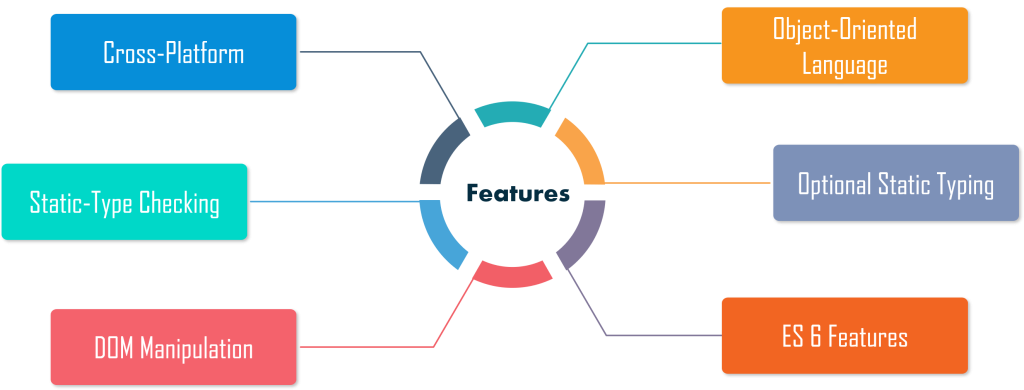
TypeScript offers a number of features that make it a powerful and versatile language. Some of the key features of TypeScript include:
- Static typing: TypeScript is a statically typed language, which means that the types of variables and expressions are checked at compile time. This helps to prevent errors and make your code more reliable.
- Object-oriented programming: TypeScript supports object-oriented programming, which makes it a good choice for developing large and complex applications.
- Cross-platform: Cross-platform in Typescript refers to the ability of the code written in Typescript to run and work on multiple platforms or environments, such as web browsers, mobile devices (iOS, Android), desktop applications (Windows, macOS), and even server-side environments (Node.js).
- Static type checking: Static type checking in TypeScript is a process that ensures the correctness of types at compile-time. It checks the types of variables, function parameters, and return values based on their type annotations. This helps identify potential errors and avoid type-related bugs during development, enhancing code quality and maintainability.
- Functional programming: TypeScript also supports functional programming, which can be useful for developing code that is more concise and easier to read.
- Asynchronous programming: TypeScript supports asynchronous programming, which is essential for developing efficient and responsive applications.
- Modules: TypeScript supports modules, which makes it easy to organize your code and improve its readability.
What is the workflow of Typescript?
The workflow of TypeScript typically involves the following steps:
1. Install and configure TypeScript: TypeScript can be installed via npm using the command `npm install -g typescript`. A `tsconfig.json` file is commonly used to configure compilation options.
2. Write TypeScript code: Create a `.ts` file and write TypeScript code with type annotations, interfaces, and classes.
3. Compile TypeScript: Run the command `tsc` in the command line to compile TypeScript code into JavaScript.
4. Use the compiled JavaScript: The TypeScript compiler generates a `.js` file that can be used in any JavaScript environment or included in HTML files.
How Typescript Works & Architecture?
Here is how TypeScript works:
- The TypeScript compiler reads your TypeScript code.
- The compiler checks your code for errors, including type errors.
- If there are no errors, the compiler converts your TypeScript code to JavaScript.
- The JavaScript code is then executed.
The TypeScript compiler is written in TypeScript itself. This makes it easy to extend the compiler with new features.
The TypeScript architecture is divided into three layers:
- Parser: The parser reads your TypeScript code and converts it into an abstract syntax tree (AST).
- Checker: The checker checks the AST for errors, including type errors.
- Generator: The generator converts the AST back into JavaScript code.
How to Install and Configure Typescript?
To install and configure TypeScript, follow these steps:
Step 1: Install Node.js
– TypeScript requires Node.js to be installed on your computer.
– Visit https://nodejs.org and download the appropriate installer for your operating system.
– Run the installer and follow the instructions to complete the installation.
Step 2: Install TypeScript
– Open a terminal or command prompt.
– Use the following command to install TypeScript globally:
npm install -g typescript
Step 3: Verify the Installation
– To verify that TypeScript is installed, enter the following command in the terminal:
tsc --version
– If it shows the version number, TypeScript is installed correctly.
Step 4: Create a TypeScript Configuration File (optional)
– Create a new folder for your TypeScript project.
– Open a terminal or command prompt and navigate to the project folder.
– Run the following command to initialize a TypeScript project and create a tsconfig.json file:
tsc --init
– This command creates a default tsconfig.json file in the project folder.
Step 5: Configure the tsconfig.json File (optional)
– Open the tsconfig.json file in a text editor.
– Update the compiler options based on your needs. For example, you can change the “target” option to specify the version of ECMAScript you are compiling to, or the “outDir” option to specify the output folder for the compiled JavaScript files.
– For more information, refer to the TypeScript documentation on compiler options: https://www.typescriptlang.org/tsconfig
Step 6: Write TypeScript code
– Create a new .ts file in your project folder.
– Write TypeScript code in the file.
– For example, you can create a file named `app.ts`:
let message: string = "Hello, TypeScript!";
console.log(message);
Step 7: Compile TypeScript to JavaScript
– Open a terminal or command prompt and navigate to the project folder.
– Run the following command to compile the TypeScript code to JavaScript:
tsc app.ts
– This command generates a corresponding JavaScript file (`app.js` in this example) in the same folder.
That’s it! You have successfully installed and configured TypeScript.
Step by Step Tutorials for Typescript for the hello world program
Here is a step-by-step tutorial on how to write a “hello world” program in TypeScript:
- Create a new file called
hello.ts
. - Write the following code in the file:
console.log("hello world");
- Save the file.
- Compile the code by running the following command:
tsc hello.ts
- This will create a file called
hello.js
. - Run the JavaScript file by running the following command:
node hello.js
This will print the message “hello world” to the console.
Here is a breakdown of the code:
- The
console.log()
function is used to print a message to the console. - The string literal “hello world” is the message that will be printed to the console.
The tsc
command is used to compile TypeScript code to JavaScript. The hello.ts
file is the TypeScript file that we want to compile.
The node
command is used to run JavaScript code. The hello.js
file is the JavaScript file that we want to run.