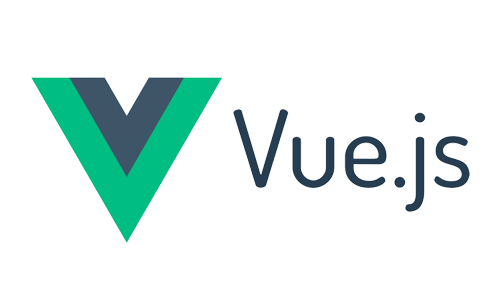
What is vue.js?
Vue.js (pronounced /vjuː/, like “view”) is an open-source, progressive JavaScript framework for building user interfaces and single-page applications. It is lightweight and easy to learn, making it a popular choice for developers of all levels.
What is top use cases of vue.js?
Here are some key features of Vue.js:
- Progressive: Vue.js can be used as a library or a full-fledged framework. You can start with the basics and gradually add more features as your project grows.
- Reactive: Vue.js uses a reactive data model, which means that changes to the data are automatically reflected in the UI without the need for manual DOM manipulation.
- Composable: Vue.js components are reusable and self-contained, making it easy to build large and complex applications.
- Virtual DOM: Vue.js uses a virtual DOM to efficiently update the UI, resulting in high performance.
- Easy to learn: Vue.js has a simple API and a large community of developers. This makes it easy to learn and get started with.
What are feature of vue.js?
Features of Vue.js:
- Vue.js is a progressive JavaScript framework for building user interfaces.
- It offers reactivity, allowing automatic updates to the DOM when the data changes.
- Vue.js uses a virtual DOM, which improves performance by minimizing unnecessary updates.
- It provides easy two-way data binding between the JavaScript data model and the HTML.
- Vue.js has a component-based architecture, enabling composition and reuse of UI elements.
- It supports declarative rendering syntax using HTML-based template syntax.
- Vue.js provides routing capabilities for building single-page applications.
- It has a vibrant ecosystem with a rich collection of official and community-supported libraries and plugins.
What is the workflow of vue.js?
The typical Vue.js workflow involves the following steps:
- Setting up your project: You can set up your project using Vue CLI or other tools.
- Writing your code: You’ll write your code in HTML, CSS, and JavaScript.
- Compiling your code: Vue CLI will compile your code into a format that can be understood by the browser.
- Running your application: You can run your application in a development server or deploy it to a production environment.
How vue.js Works & Architecture?
Vue.js is a JavaScript framework for building user interfaces. It is based on the Model-View-ViewModel (MVVM) pattern, which separates the data (Model), the user interface (View), and the glue that binds them together (ViewModel).
Here’s a breakdown of how Vue.js works and its architecture:
- Model: The Model represents the data of your application.
It can be a simple JavaScript object or a more complex data structure.
Any changes to the Model are automatically reflected in the View. - View: The View is the user interface of your application.
It is typically written in HTML, but can also be written in other languages like JSX.
The View uses data bindings to connect to the Model. - ViewModel: The ViewModel is the glue that binds the Model and the View together.
It is a Vue.js instance that contains the data, logic, and methods for your component.
The ViewModel reacts to changes in the Model and updates the View accordingly.
Model:
- The Model represents the data of your application.
- It can be a simple JavaScript object or a more complex data structure.
- Any changes to the Model are automatically reflected in the View.
View:
- The View is the user interface of your application.
- It is typically written in HTML, but can also be written in other languages like JSX.
- The View uses data bindings to connect to the Model.
ViewModel:
- The ViewModel is the glue that binds the Model and the View together.
- It is a Vue.js instance that contains the data, logic, and methods for your component.
- The ViewModel reacts to changes in the Model and updates the View accordingly.
How to Install and Configure vue.js?
To install Vue.js, you can use either a package manager like npm or yarn, or include Vue.js as a script in your HTML file. Below is an example of installing Vue.js using npm:
npm install vue
Once installed, you can use Vue.js by including it in your project:
<script src="path/to/vue.js"></script>
Step by Step Tutorials for vue.js for hello world program
A basic “Hello World” program in Vue.js involves the following steps:
Step 1: Include Vue.js in your HTML file (as mentioned in the installation step).
Step 2: Create a Vue instance and specify the target element to bind it with:
var app = new Vue({
el: '#app',
});
Step 3: Create a template containing your desired output:
<div id="app">
<p>Hello World!</p>
</div>
Step 4: View the result in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Vue.js Hello World</title>
<script src="path/to/vue.js"></script>
</head>
<body>
<div id="app">
<p>Hello World!</p>
</div>
<script>
var app = new Vue({
el: '#app',
});
</script>
</body>
</html>
You can then preview the HTML file in a browser, and it should display “Hello World!”.