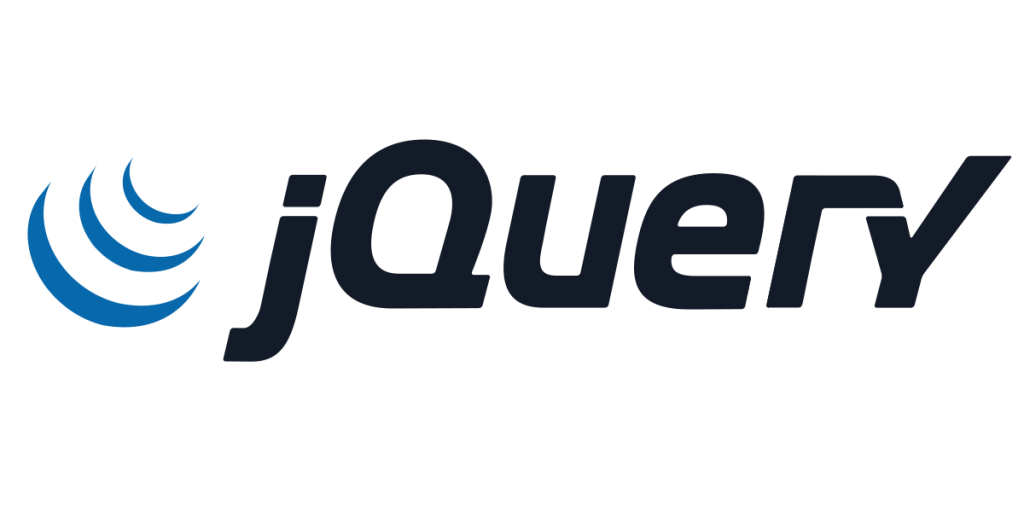
What is jQuery ?
jQuery is a lightweight, fast, small, and feature-rich JavaScript library that helps web developers work with HTML and make their websites more interactive and dynamic. It simplifies tasks like changing content, handling user actions, creating animations, and fetching data from the server without reloading the page. With jQuery, developers can achieve more with less code and ensure their websites work well across different browsers.
What is top use cases of jQuery ?
Some top use cases of jQuery include:
1. DOM manipulation: jQuery provides a number of methods for manipulating the DOM, such as selecting elements, adding and removing elements, and changing the content of elements.
2. Event handling: jQuery provides a number of methods for handling events, such as mouse clicks, keyboard presses, and form submissions.
3. Animation: jQuery provides a number of methods for animating elements, such as fading in and out, sliding, and rotating.
4. AJAX requests: jQuery provides a number of methods for making AJAX requests, which allow you to exchange data with a server without reloading the page.
5. UI components: jQuery offers a wide range of UI components like date pickers, sliders, and tooltips.
What are feature of jQuery ?
Some of the key features of jQuery include:
- Shorthand syntax: jQuery’s syntax is much shorter and more concise than standard JavaScript, making it easier to write and read.
- Efficiency: jQuery is very efficient, making it a good choice for performance-sensitive applications.
- Cross-platform compatibility: jQuery is cross-platform, meaning that it can be used on any platform that supports JavaScript.
- Extensive library: jQuery has a large and extensive library of methods, making it a powerful tool for web development.
What is the workflow of jQuery ?
The workflow of jQuery typically involves:
1. Include the jQuery library in your HTML file using a `<script>` tag.
2. Select HTML elements using jQuery selectors.
3. Perform actions or manipulate the selected elements using jQuery methods and functions.
4. Handle events triggered by the user or browser.
5. Make AJAX requests to retrieve or send data to servers.
How jQuery Works & Architecture?
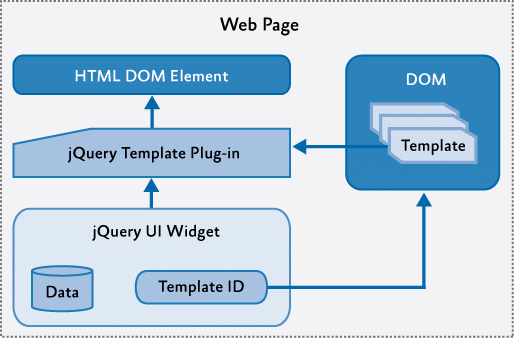
How to Install and Configure jQuery ?
To install and configure jQuery, follow these steps:
- Download the jQuery library:
- Visit the official jQuery website at https://jquery.com/.
- Click on the “Download” button to get the latest version of jQuery.
- Choose either the compressed or uncompressed version based on your preference.
- Include jQuery in your HTML file:
- Copy the downloaded jQuery file into your project directory.
- Add the following line within the
<head>
section of your HTML file:<script src="path/to/jquery.js"></script>
- Replace “path/to/jquery.js” with the actual path to the jQuery file.
- Verify the installation:
- Open your HTML file in a web browser.
- Right-click on the page and select “Inspect” or press
Ctrl + Shift + I
to open the browser’s Developer Tools. - In the Developer Tools, navigate to the “Console” tab.
- Type
jQuery
or$
into the console and press Enter. If jQuery is correctly installed, it should return the jQuery version number.
You have now installed jQuery and can start using its libraries and functions in your web project. Remember to properly link to the jQuery file in any HTML file where you need to use jQuery functionality.
Step by Step Tutorials for jQuery for hello world program
For a step-by-step tutorial and a “Hello, World!” program using jQuery, here’s a basic example:
Step 1: Create an HTML file, for example, “index.html”.
Step 2: Include the jQuery library by adding the following code inside the `<head>` tag:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 3: Add a `<script>` section below the jQuery inclusion to write your JavaScript code. For example:
<script>
$(document).ready(function() {
// This function will be executed once the DOM is fully loaded
$("button").click(function() {
// This function will be executed when a button is clicked
alert("Hello, World!");
});
});
</script>
Step 4: Add a `<button>` element in the body of your HTML file:
<body>
<button>Click me</button>
</body>
Step 5: Save and open the HTML file in a web browser. Whenever the button is clicked, it will trigger an alert saying “Hello, World!”.
In Another Example
Here is a step-by-step tutorial for creating a “Good Morning” program using jQuery:
- Create a new HTML file.
- Add the following code to the HTML file:
HTML
<html>
<head>
<title>Good Morning</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<h1>Good Morning</h1>
</body>
</html>
- Save the HTML file.
- Open the HTML file in a web browser.
You should see the text “Good Morning” displayed on the web page.