What is Array in PHP?
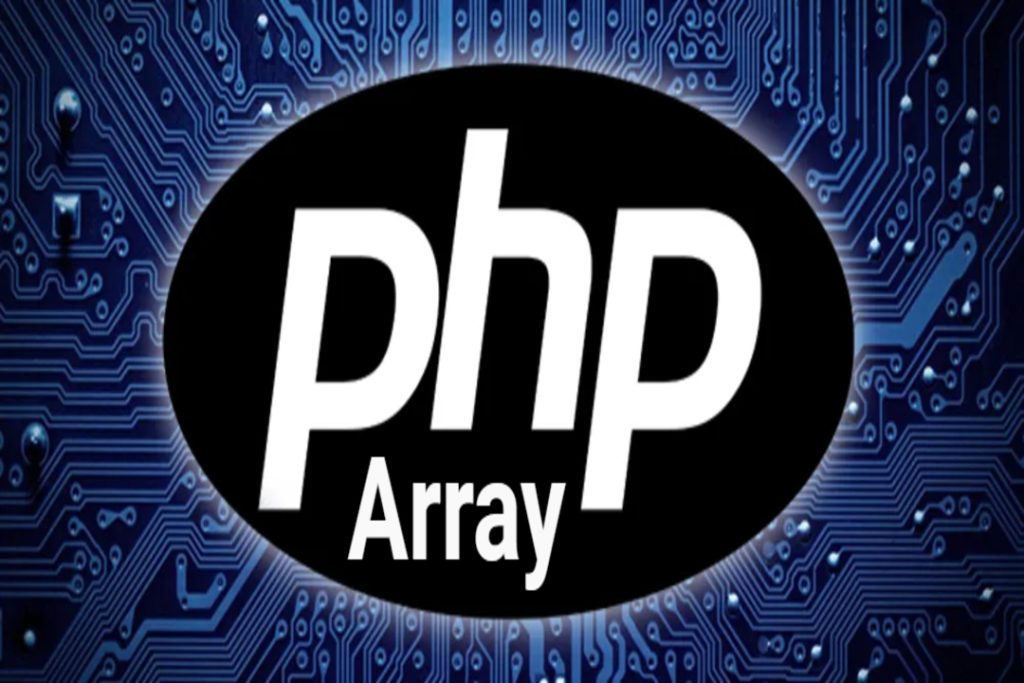
PHP arrays is a type of data structure that can hold multiple values of similar type in a single variable.
The Advantage of PHP Array is
- Less Code: We don’t need to define multiple variables.
- Easy to traverse: By using single loop, we can traverse all the elements of an array.
- Sorting: We can sort the elements of array.
There are 3 types of array in PHP.
- Indexed Array
- Associative Array
- Multidimensional Array
1. Indexed Array:
An indexed array is a type of array where each element is assigned a numeric index, starting from 0 and increasing by 1 for each subsequent element.
There are two ways to define indexed array:
1st way:
$animals= array("dog", "cat", "donkey", "monkey");
Example:
<?php
$animals=array("dog", "cat", "donkey", "monkey");
echo "animals are: $animals[0], $animals[1], $animals[2] and $animals[3]";
?>
Output:
animals are: dog, cat, donkey, monkey
2nd way:
$animals [0]="dog";
$animals [1]="cat";
$animals [2]="donkey";
$animals [3]="monkey";
Example:
<?php
$animals [0]="summer";
$animals [1]="winter";
$animals [2]="spring";
$animals [3]="autumn";
echo "animals are: $animals [0], $animals [1], $animals [2] and $animals [3]";
?>
Output:
animals are: dog, cat, donkey, monkey
2. Associative Array:
An associative array is a type of array where each element is assigned a unique key instead of a numeric index. This means that you can access the elements of an associative array using their keys instead of their positions.
There are two ways to define associative array:
1st way:
$salary=array("Ram"=>"550000","Shyam"=>"250000","Rahul"=>"200000");
Example:
<?php
$salary=array("Ram "=>"550000","Shyam "=>"250000","Rahul"=>"200000");
echo "Ram salary: ".$salary["Ram "]."<br/>";
echo "Shyam salary: ".$salary["Shyam"]."<br/>";
echo "Rahul salary: ".$salary["Rahul"]."<br/>";
?>
Output:
Ram salary: 550000
Shyam salary: 250000
Rahul salary: 200000
2nd way:
$salary["Ram"]="550000";
$salary["Shyam"]="250000";
$salary["Rahul"]="200000";
Example:
<?php
$salary["Ram"]="550000";
$salary["Shyam"]="250000";
$salary["Rahul"]="200000";
echo "Ram salary: ".$salary["Ram"]."<br/>";
echo "Shyam salary: ".$salary["Shyam"]."<br/>";
echo "Rahul salary: ".$salary["Rahul"]."<br/>";
?>
Output:
Ram salary: 550000
Shyam salary: 250000
Rahul salary: 200000
3. Multidimensional Arrays:
A multidimensional array is an array that contains one or more arrays as its elements. Each array within the multidimensional array is known as a dimension, and can contain its own set of elements. This allows you to store complex data structures in a single variable.
Example:
<?php
$cars = array (
array("Volvo",22,18),
array("BMW",15,13),
array("Saab",5,2),
array("Land Rover",17,15)
);
echo $cars[0][0].": In stock: ".$cars[0][1].", sold: ".$cars[0][2].".<br>";
?>