Based on the criteria mentioned above, we have divided databases in mainly two types:
- Relational Database, where the data has a relation in between them. SQL and SQLite are the best examples of such databases.
- Non-relational Database, where data stored is in documents, and data has no relation between them. They are quite unstructured. MongoDB is a bright example of such a database. A non-relational database is also known as a NoSQL database.
Now in the case of Flutter app development, we have two sets of databases, Relational and NoSQL databases for Flutter. To make the choices easier for you, we have mentioned a list of flutter local databases.
We hope that it will prove beneficial to the Flutter Developers!
What Are The Flutter App Databases You Can Use?
Based on the database selection criterion factors for the database, we have picked out some of the top flutter local DBs used in making Android and iOS apps.
The below list has the best databases for your flutter mobile app. Don’t forget to choose one as per your requirements:
- MySQL: it is an open-source Flutter for relational databases that is multi-threaded. You can easily use this for Flutter and many other mobile app development frameworks.
- PostgreSQL: It is also a relational database that is a highly powerful, customizable, open-source, and object-based database.
- MariaDB: This is a popular open-source relational database created by MySQL developers.
- MongoDB: Call it as NoSQL or non-relational schemaless, JSON document database. It is widely popular for its flexibility and scalability.
- RethinkDB: it is an open-source document-oriented database that stores data in JSON format and can simply sync in real-time applications.
- Realm: Being a non-relational object-oriented database, it’s 10-times faster that can be easily customized for iOS and Android applications.
- SQLite: We can call it an embedded database that has local data storage capabilities that get implemented in a Flutter app using SQflite.
- Firebase: It is a cloud-hosted Google-owned database that hosts and syncs data in real-time across multiple devices for multi-user during high availability requirements.
- Hive: It is native to dart and is a fast flutter NoSQL database that requires no device-specific implementations.
sqlite:-
SQLite plugin for Flutter. Supports iOS, Android and MacOS.
- Support transactions and batches
- Automatic version managment during open
- Helpers for insert/query/update/delete queries
- DB operation executed in a background thread on iOS and Android
Other platforms support:
- Linux/Windows/DartVM support using sqflite_common_ffi
- Web is not supported.
Usage example:
- notepad_sqflite: Simple flutter notepad working on iOS/Android/Windows/linux/Mac
Opening a database
A SQLite database is a file in the file system identified by a path. If relative, this path is relative to the path obtained by getDatabasesPath()
, which is the default database directory on Android and the documents directory on iOS.
var db = await openDatabase('my_db.db');
There is a basic migration mechanism to handle schema changes during opening.
Many applications use one database and would never need to close it (it will be closed when the application is terminated). If you want to release resources, you can close the database.
await db.close();
Raw SQL queries
// Get a location using getDatabasesPath
var databasesPath = await getDatabasesPath();
String path = join(databasesPath, 'demo.db');
// Delete the database
await deleteDatabase(path);
// open the database
Database database = await openDatabase(path, version: 1,
onCreate: (Database db, int version) async {
// When creating the db, create the table
await db.execute(
'CREATE TABLE Test (id INTEGER PRIMARY KEY, name TEXT, value INTEGER, num REAL)');
});
// Insert some records in a transaction
await database.transaction((txn) async {
int id1 = await txn.rawInsert(
'INSERT INTO Test(name, value, num) VALUES("some name", 1234, 456.789)');
print('inserted1: $id1');
int id2 = await txn.rawInsert(
'INSERT INTO Test(name, value, num) VALUES(?, ?, ?)',
['another name', 12345678, 3.1416]);
print('inserted2: $id2');
});
// Update some record
int count = await database.rawUpdate(
'UPDATE Test SET name = ?, value = ? WHERE name = ?',
['updated name', '9876', 'some name']);
print('updated: $count');
// Get the records
List<Map> list = await database.rawQuery('SELECT * FROM Test');
List<Map> expectedList = [
{'name': 'updated name', 'id': 1, 'value': 9876, 'num': 456.789},
{'name': 'another name', 'id': 2, 'value': 12345678, 'num': 3.1416}
];
print(list);
print(expectedList);
assert(const DeepCollectionEquality().equals(list, expectedList));
// Count the records
count = Sqflite
.firstIntValue(await database.rawQuery('SELECT COUNT(*) FROM Test'));
assert(count == 2);
// Delete a record
count = await database
.rawDelete('DELETE FROM Test WHERE name = ?', ['another name']);
assert(count == 1);
// Close the database
await database.close();
INSTALLING
install in pubspec.yml
run in flutter terminal
$ flutter pub add sqflite
This will add a line like this to your package’s pubspec.yaml (and run an implicit dart pub get
):
dependencies:
sqflite: ^2.0.0+3
Alternatively, your editor might support flutter pub get
. Check the docs for your editor to learn more.
Import it
Now in your Dart code, you can use
import 'package:sqflite/sqflite.dart';
Version of SQLite:
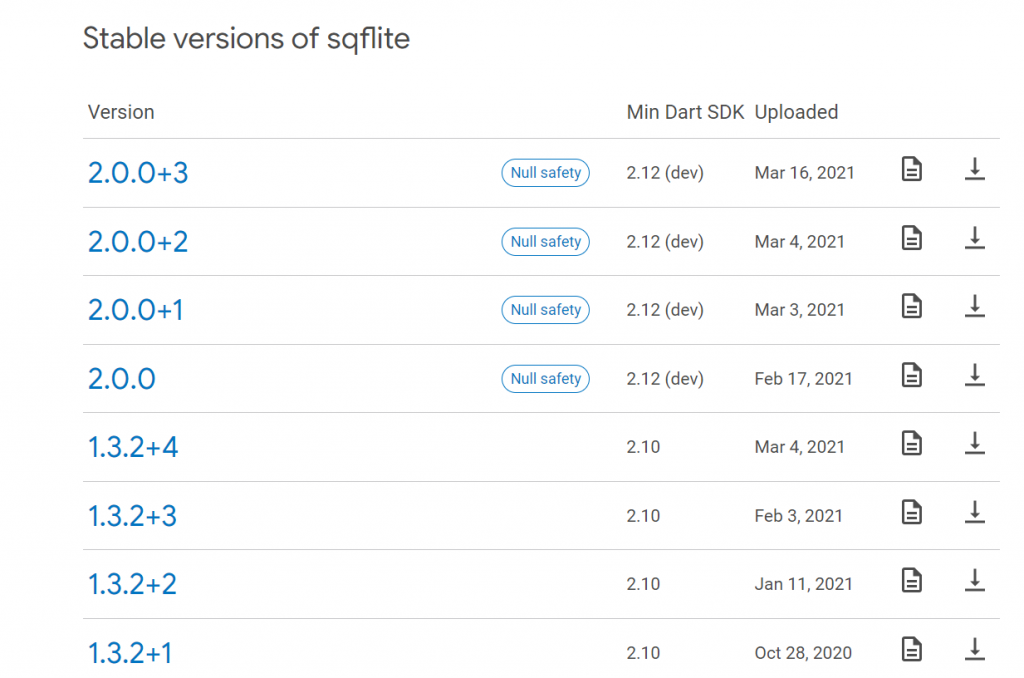