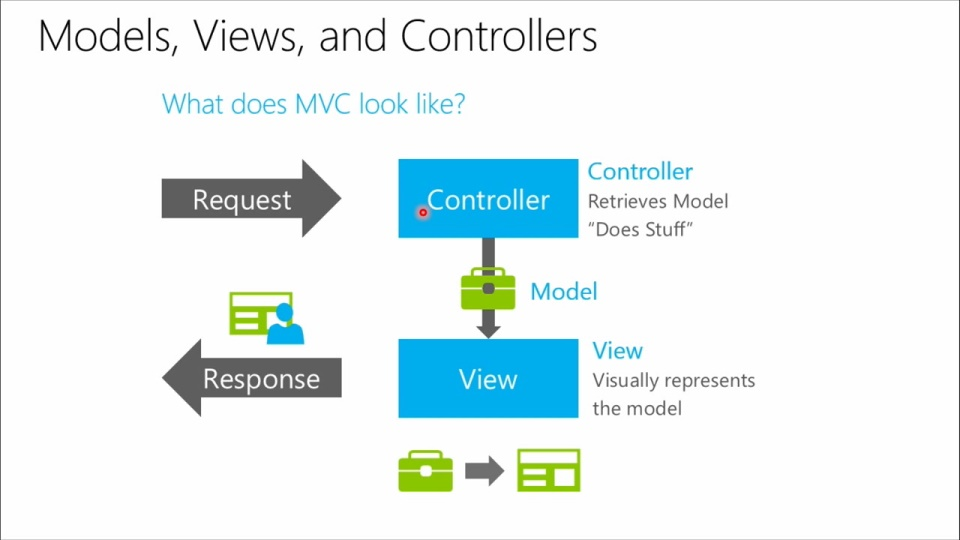
What is asp.net-mvc?
ASP.NET MVC is a web development framework developed by Microsoft that follows the Model-View-Controller architectural pattern. It is a powerful and flexible framework for building dynamic and scalable web applications.
What are the top use cases of asp.net-mvc?
- Building enterprise-level web applications
- Creating content management systems
- Developing e-commerce platforms
- Building social networking sites
- Creating APIs and web services
What are the features of asp.net-mvc?
- Separation of concerns: ASP.NET MVC promotes the separation of concerns between the view, model, and controller. This makes code more reusable, maintainable, and testable.
- Routing: ASP.NET MVC provides a powerful routing system that allows you to map incoming requests to specific controllers and actions.
- Dependency injection: ASP.NET MVC supports dependency injection, which makes it easy to create and manage dependencies between objects.
- Asynchronous programming: ASP.NET MVC supports asynchronous programming, which can improve the performance of your applications.
What is the workflow of asp.net-mvc?
- The user sends a request to the server.
- The request is intercepted by the MVC framework’s routing system.
- The routing system maps the request to the appropriate controller action based on the URL.
- The controller action processes the request and interacts with the model to retrieve or update data.
- The controller action selects the appropriate view to render the response.
- The view is rendered and returned as the response to the user.
How asp. net-mvc Works & Architecture?
Let’s explore the MVC architecture supported in ASP.NET.
MVC is an acronym for Model, View, and Controller, which serves to break down an application into three distinct components: Model, View, and Controller.
Model: The Model component represents the data structure. In C#, a class is employed to define a model, and model objects store data retrieved from the database.
The Model represents data.
View: In MVC, the View acts as the user interface. It presents model data to users and allows them to make modifications. In ASP.NET MVC, the View consists of HTML, CSS, and special syntax (Razor syntax) that facilitates communication with both the model and the controller.
The View constitutes the User Interface.
Controller: The Controller manages user requests. Typically, users interact with the View and trigger an HTTP request, which is then handled by the Controller. The Controller processes the request and returns the appropriate view as a response.
The Controller functions as the request handler.
The diagram below illustrates the interaction among the Model, View, and Controller components.
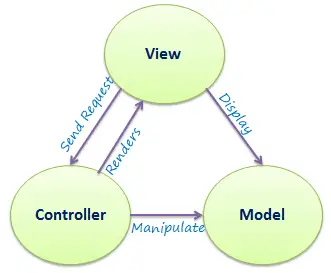
This picture shows how a user’s request works in ASP.NET MVC, including the View and Controller.
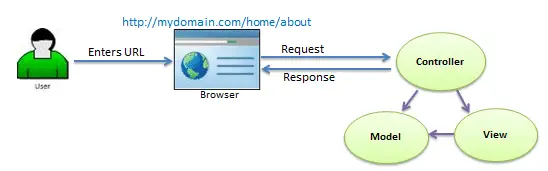
Request Flow in MVC Architecture
In the diagram above, here’s how it works when you type a website address into your browser:
- Your browser sends the request to a webserver.
- The webserver then sends the request to a controller.
- The controller does its job by using the right view and models for that request.
- After that, it creates a response and sends it back to your browser.
How to Install and Configure asp.net-mvc?
To install and configure ASP.NET MVC, you typically need to:
- Install Visual Studio or Visual Studio Code, which come with ASP.NET MVC project templates.
- Create a new ASP.NET MVC project using one of the templates.
- Configure the project settings and dependencies, including the .NET framework version and any required packages.
- Start developing your application by creating controllers, views, and models.
Step by Step Tutorials for asp.net-mvc for Hello World program
To create a Hello World program in ASP.NET MVC, follow these steps:
- Create a new ASP.NET Core project using the following command:
dotnet new mvc
2. Open the Startup.cs
file and add the following code to the ConfigureServices()
method:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
}
- Create a new controller called
HomeController
. - Add the following code to the
HomeController
class:
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
5. Create a new view called Index.cshtml
and add the following code to it:
<h1>Hello World!</h1>
6. Run the application and navigate to http://localhost:5000/
in your web browser. You should see the message “Hello World!” displayed on the page.
Here is a more detailed explanation of each step:
- Create a new ASP.NET Core project. This will create a new ASP.NET Core project with the default configuration.
- Configure the Startup class. The
Startup
class is responsible for configuring the ASP.NET Core application. In this case, we are adding theAddControllersWithViews()
method to theConfigureServices()
method. This will register the controllers and views with the ASP.NET Core application. - Create a new controller. Controllers are responsible for handling user requests and interacting with the model and view. In this case, we are creating a new controller called
HomeController
. - Add code to the controller. The
HomeController
class contains a single action method calledIndex()
. This method is responsible for handling the request for the home page. In this case, we are simply returning theView()
method. This will render the view calledIndex.cshtml
. - Create a new view. Views are responsible for rendering the user interface. In this case, we are creating a new view called
Index.cshtml
. This view contains a single<h1>
element with the text “Hello World!”. - Run the application. Once you have created the controller and view, you can run the application. To do this, simply press
F5
in Visual Studio.
If you followed these steps correctly, you should see the message “Hello World!” displayed on the page when you navigate to http://localhost:5000/
in your web browser.
Congratulations! You have created your first ASP.NET MVC application.