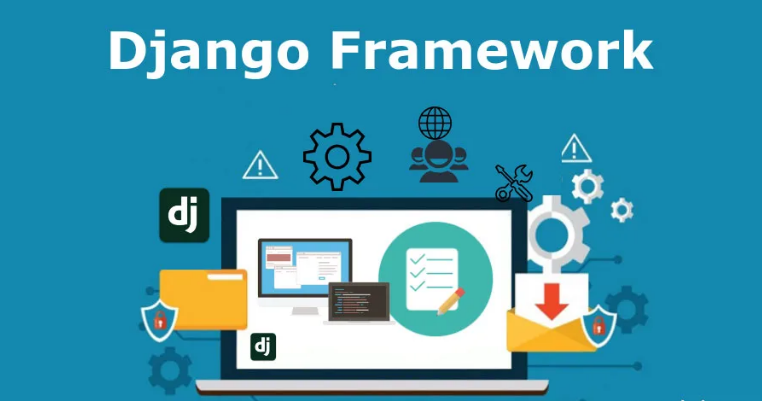
Django is a popular web framework written in Python. It follows the model-view-controller (MVC) architectural pattern and is designed to help developers build web applications quickly and efficiently.
Top Use Cases of Django
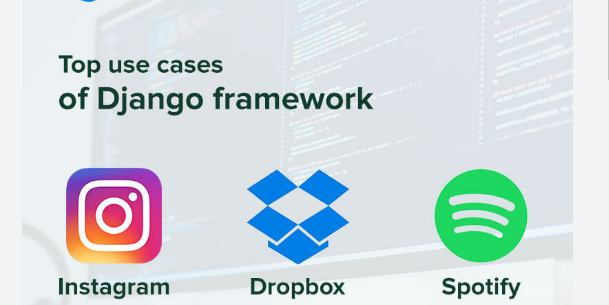
- Content Management Systems (CMS): Django provides a robust framework for building CMS platforms, allowing users to easily manage and publish content on their websites.
- E-commerce Platforms: Many e-commerce websites are built using Django due to its scalability, security features, and support for handling large amounts of data.
- Social Networking Sites: Django’s authentication and user management features make it an ideal choice for building social networking platforms where users can create profiles, connect with others, and share content.
- Data Analysis and Visualization: Django’s integration with data analysis libraries like Pandas and Matplotlib makes it a popular choice for building data-driven applications.
Features of Django
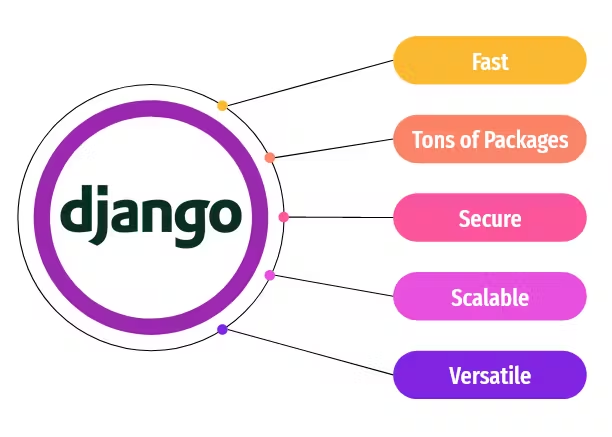
- Object-Relational Mapping (ORM): Django provides a high-level interface for interacting with databases, allowing developers to work with database records using Python objects.
- URL Routing: Django’s URL routing system allows for the mapping of URLs to specific views, enabling clean and organized URL structures.
- Templating Engine: Django’s built-in templating engine makes it easy to create dynamic web pages by separating the design and logic.
- Authentication and Authorization: Django provides robust authentication and authorization mechanisms, ensuring secure access to web applications.
- Robust security: Django takes security seriously and has a number of features built in to protect your applications from attack.
- Easy to learn: Django is designed to be easy to learn, even for beginners. The documentation is clear and concise, and there are many online tutorials and resources available.
- Flexible: Django is a very flexible framework, so you can use it to build a wide variety of applications.
- Highly scalable: Django is designed to be scalable, so you can easily add more features and users to your applications as needed.
Workflow of Django
- URL Routing: Incoming requests are first matched against URL patterns defined in the application’s URLs configuration.
- View Functions: Once a URL is matched, the corresponding view function is called, which processes the request and returns a response.
- Models and Database Operations: Django’s ORM allows developers to define models that represent database tables. Models can be used to perform various database operations like querying, inserting, updating, and deleting records.
- Templates: Views often render HTML templates that contain the structure and presentation of the web pages.
- Forms and Validation: Django provides a powerful form handling mechanism, including automatic form validation and sanitization.
- Rendering and Response: The final step in the workflow involves rendering the response and sending it back to the user’s browser.
How Django Works & Architecture
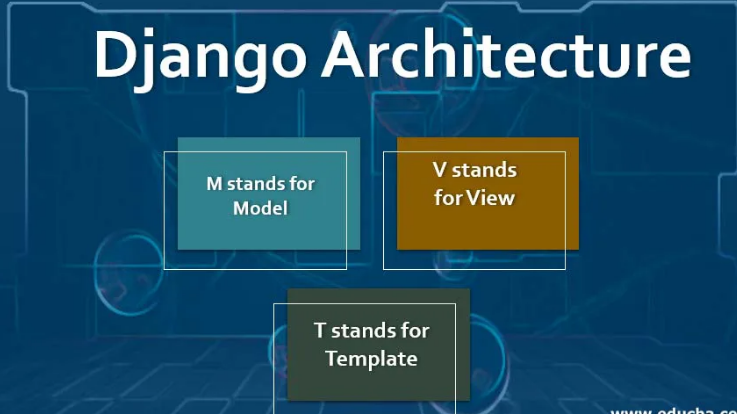
Django is a Python web framework that follows the Model-View-Template (MVT) architectural pattern. The MVT pattern separates the application into three layers:
- Model: The model layer is responsible for storing and retrieving data from the database. It is implemented in Python classes that inherit from the
django.db.models.Model
class. - View: The view layer is responsible for handling user requests and returning the appropriate response. It is implemented in Python functions that take a request object and return a response object.
- Template: The template layer is responsible for rendering the HTML pages that are displayed to the user. It is implemented in templates that use the Django Template Language (DTL).
The following diagram illustrates the MVT architecture of Django:
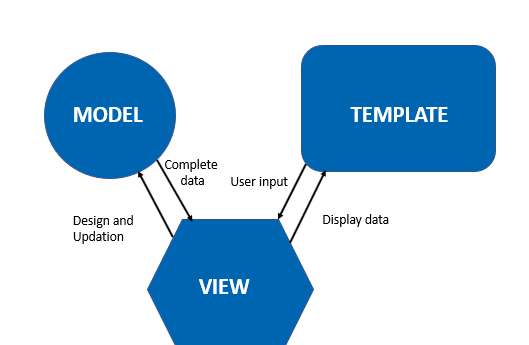
The Django request-response cycle works as follows:
- A user sends a request to the Django server.
- The Django server parses the request and determines the appropriate view function to handle it.
- The view function retrieves the data from the model layer and returns a response object to the Django server.
- The Django server renders the template layer with the data from the response object and sends the rendered HTML page to the user.
How to Install and Configure Django
To install Django, follow these steps:
- Ensure you have Python installed on your system.
- Open the command prompt or terminal.
- Run the following command to install Django:
pip install django
. - Once Django is installed, you can start a new Django project using the command
django-admin startproject project_name
. - Configure the database settings in the project’s settings.py file.
- Run the development server using the command
python manage.py runserver
. - Visit http://localhost:8000 in your browser to see the default Django welcome page.
Remember to keep on learning and exploring Django’s vast capabilities. Happy coding!
Step by Step Tutorials for django for hello world program
Sure! Here are step-by-step instructions to create a “Hello, World!” program using Django:
Step 1: Install Django
– Make sure you have Python installed on your system (version 3.6 or above).
– Open your terminal or command prompt and run the following command to install Django:
pip install django
Step 2: Start a New Django Project
– Create a new directory for your Django project and navigate inside it through the terminal.
– Run the following command to start a new Django project:
django-admin startproject helloworld
Step 3: Create an App
– Change into the project directory:
cd helloworld
– Run the following command to create a new Django app:
python manage.py startapp myapp
Step 4: Configure the Project
– Open the `helloworld/settings.py` file in a text editor.
– Add `’myapp’` to the `INSTALLED_APPS` list. Step
5: Create a View – Open the `myapp/views.py` file and add the following code:
from django.http import HttpResponse
def hello_world(request):
return HttpResponse("Hello, World!")
Step 6: Create URLs
– Open the `helloworld/urls.py` file and add the following code:
from django.urls import path
from myapp.views import hello_world
urlpatterns = [
path('hello/', hello_world)
]
Step 7: Run the Development Server
– Go back to the project’s root directory and run the following command:
python manage.py runserver
Step 8: Test the Application
– Open your browser and navigate to `http://localhost:8000/hello/`.
– You should see the “Hello, World!” message displayed.
Congratulations! You have created a simple “Hello, World!” program using Django.