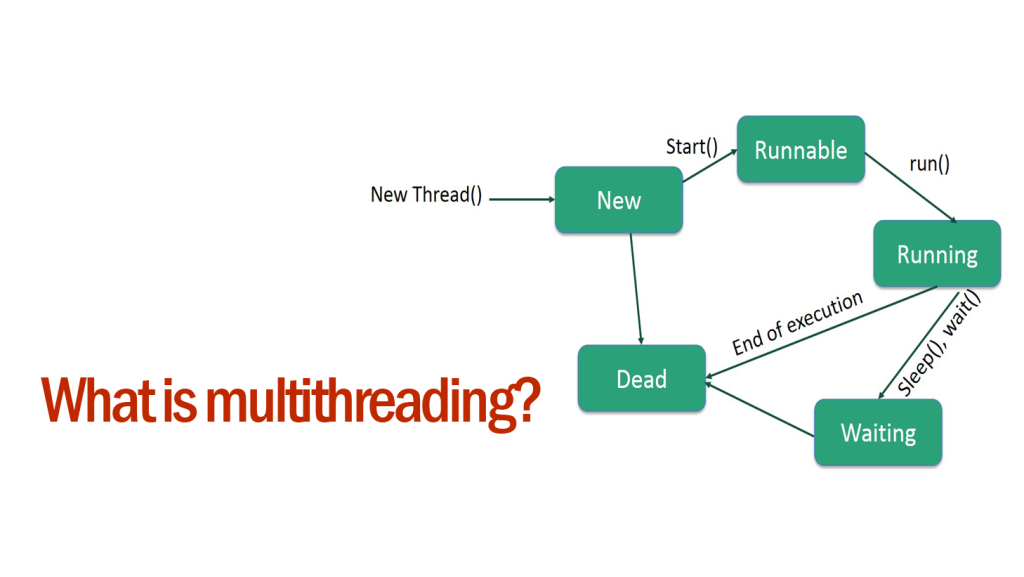
What is multithreading?
Multithreading is a programming technique that allows a single process to execute multiple threads concurrently. A thread is a lightweight subprocess that can be independently scheduled and executed by the operating system. Threads share the resources of their parent process, including memory and open files.
What is top use cases of multithreading?
The top use cases of multithreading include:
- Improving performance: Multithreading can help in utilizing the available CPU resources efficiently by dividing tasks into multiple threads.
- Responsiveness: In user interface applications, multithreading can be used to keep the user interface responsive while performing time-consuming operations in the background.
- Parallel computing: Multithreading can be used to divide a large computational task into smaller tasks that can be executed in parallel, thereby reducing the overall execution time.
- Real-time systems: In applications that require real-time responsiveness, such as video games or real-time simulations, multithreading can help in meeting the tight deadlines.
What are feature of multithreading?
- Thread creation and management: Multithreading allows creating and managing multiple threads within a single process.
- Synchronization: Threads can be synchronized to access shared resources or to ensure proper order of execution.
- Communication: Threads can communicate with each other using shared data or inter-process communication mechanisms.
- Thread pooling: Multithreading allows creating a pool of threads that can be reused to improve performance by avoiding the overhead of thread creation.
What is the workflow of multithreading?
The workflow of multithreading typically involves the following steps:
- Thread creation: Threads are created by the operating system or explicitly by the programmer.
- Thread execution: Each thread executes its own set of instructions in parallel with other threads.
- Thread synchronization: Synchronization mechanisms are used to coordinate access to shared resources and ensure thread safety.
- Thread termination: Threads can be terminated either by completing their tasks or by explicit termination calls.
How multithreading Works & Architecture?
The architecture of a multithreaded application consists of the following components:
- Process: A process is an instance of a program that is running in the operating system. A process has its own address space, which contains its code, data, and stack.
- Thread: A thread is a unit of execution within a process. A thread has its own execution context, which includes its own program counter, stack, and registers.
- Scheduler: The scheduler is a part of the operating system that is responsible for managing the execution of threads. The scheduler decides which thread to run at any given time.
- Synchronization: Synchronization is the process of coordinating the execution of multiple threads so that they do not interfere with each other.
Here is a simplified diagram of the multithreading architecture:
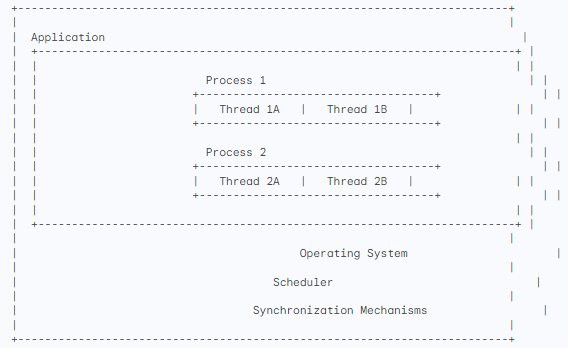
Multithreading is a powerful technique that can be used to improve the performance and responsiveness of applications. However, it is important to use multithreading carefully, as it can also lead to concurrency problems if not used properly.
How to Install and Configure multithreading?
Installing and setting up multithreading is usually included in programming languages and their tools. You don’t install multithreading separately, but you use functions or tools provided by the programming language or its libraries to work with it.
Step by Step Tutorials for multithreading for hello world program
Here’s a step-by-step guide to creating a simple multithreaded “Hello, World!” program in Java:
Step 1: Create a Thread Class
Create a class that extends the Thread class or implements the Runnable interface. For simplicity, let’s use the Runnable interface.
public class HelloWorldThread implements Runnable {
@Override
public void run() {
System.out.println("Hello, World! from Thread: " + Thread.currentThread().getName());
}
}
Step 2: Create Threads and Start Execution
In your main class, create instances of the Runnable class and start threads to execute them.
public class Main {
public static void main(String[] args) {
// Create instances of Runnable
Runnable helloWorldTask1 = new HelloWorldThread();
Runnable helloWorldTask2 = new HelloWorldThread();
// Create threads
Thread thread1 = new Thread(helloWorldTask1);
Thread thread2 = new Thread(helloWorldTask2);
// Start threads
thread1.start();
thread2.start();
}
}
Code Explanation:
- HelloWorldThread implements the Runnable interface, defining the run() method, which contains the code to be executed by each thread.
- In the main() method of the Main class, two instances of HelloWorldThread (implementing Runnable) are created.
- Two Thread objects (thread1 and thread2) are created, passing the respective Runnable instances (helloWorldTask1 and helloWorldTask2) to them.
- start() method initiates the execution of threads, and each thread will execute the run() method defined in the HelloWorldThread class.
Output:
The output will display something similar to the following (the order may vary due to concurrent execution):
Hello, World! from Thread: Thread-0
Hello, World! from Thread: Thread-1
Each thread executes the run() method independently, resulting in multiple “Hello, World!” messages printed to the console.
This simple example demonstrates the basics of multithreading in Java.