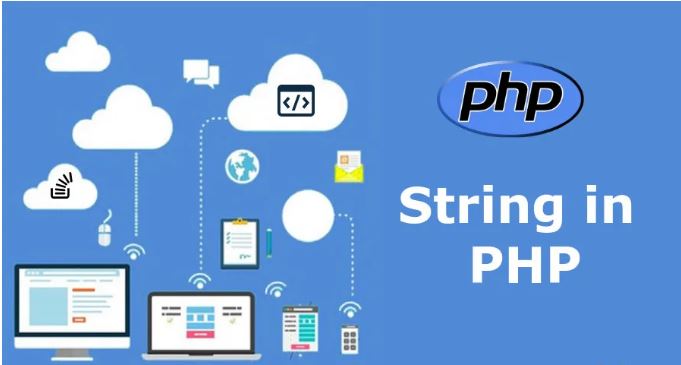
In PHP, A string is a sequence of characters, such as letters, numbers, and symbols, that is used to represent text. Strings in PHP are enclosed in quotes, either single quotes (‘) or double quotes (“).
There are two ways to specify a string literal in PHP.
- single quoted
- double quoted
Single quotes:
In PHP, single quoted strings are one way to represent a string literal. Single quoted strings are denoted by enclosing the string within single quotes (‘).
For Example:
<?php
$str='Hello I'm single quote Example';
echo $str;
?>
Output:
Hello I'm single quote Example
We can store multiple line text, special characters, and escape sequences in a single-quoted PHP string.
Example 2
<?php
$str1='Hello text
multiple line
text within single quoted string';
$str2='Using double "quote" directly inside single quoted string';
$str3='Using escape sequences \n in single quoted string';
echo "$str1 <br/> $str2 <br/> $str3";
?>
Output:
Hello text multiple line text within single quoted string
Using double "quote" directly inside single quoted string
Using escape sequences \n in single quoted string
Example3:
<?php
$num1=10;
$str1='trying variable $num1';
$str2='trying backslash n and backslash t inside single quoted string \n \t';
$str3='Using single quote \'my quote\' and \\backslash';
echo "$str1 <br/> $str2 <br/> $str3";
?>
Output:
trying variable $num1
trying backslash n and backslash t inside single quoted string \n \t
Using single quote 'my quote' and \backslash
Double Quoted:
In PHP, double quoted strings are one way to represent a string literal. Double quoted strings are denoted by enclosing the string within double quotes (“).
For Example:
<?php
$str="Hello I'm double quote Example";
echo $str;
?>
Output:
Hello I'm double quote Example
Now, you can’t use double quote directly inside double quoted string.
Example 2
<?php
$str1="Using double "quote" directly inside double quoted string";
echo $str1;
?>
Output:
Parse error: syntax error, unexpected 'quote' (T_STRING) in C:\wamp\www\string1.php on line 2
We can store multiple line text, special characters and escape sequences in a double quoted PHP string.
Example 3
<?php
$str1="Hello text
multiple line
text within double quoted string";
$str2="Using double \"quote\" with backslash inside double quoted string";
$str3="Using escape sequences \n in double quoted string";
echo "$str1 <br/> $str2 <br/> $str3";
?>
Output:
Hello text multiple line text within double quoted string
Using double "quote" with backslash inside double quoted string
Using escape sequences in double quoted string
In double quoted strings, variable will be interpreted.
Example 4
<?php
$num1=10;
echo "Number is: $num1";
?>
Output:
Number is: 10