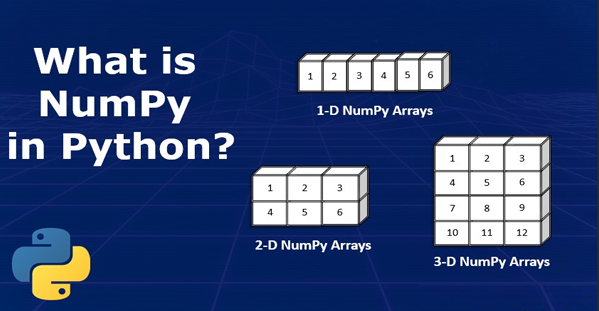
What is NumPy?
NumPy (Numerical Python) is a Python library used for working with arrays and matrices. It is a powerful tool for scientific computing and data analysis. NumPy is widely used in a variety of fields, including physics, engineering, finance, and economics.
What is top use cases of NumPy?
Top use cases of NumPy:
- Data Analysis: NumPy is extensively used for data analysis tasks, such as data cleaning, filtering, and transformation. It allows for efficient manipulation and computation on large datasets.
- Scientific Computing: NumPy is essential for scientific computing tasks, including simulations, modeling, and statistical analysis. It provides efficient algorithms for numerical operations, linear algebra, Fourier transforms, and more.
- Machine Learning: NumPy is a fundamental library in the field of machine learning. It provides the building blocks for implementing various algorithms, such as linear regression, logistic regression, and neural networks. It offers efficient array operations, which are crucial for handling large datasets.
- Image Processing: NumPy is widely used in image processing tasks, including image manipulation, enhancement, and analysis. It provides efficient methods for handling image data as arrays, making it easy to perform operations like cropping, resizing, and filtering.
What are feature of NumPy?
Features of NumPy:
- Fast: NumPy is highly optimized for performing calculations on large arrays and matrices. It uses C code for its core operations, which makes it much faster than pure Python code.
- Flexible: NumPy arrays can be multidimensional, which means they can have more than one dimension. This makes them a powerful tool for representing data with multiple dimensions, such as images and time series data.
- Versatile: NumPy provides a wide range of functions for manipulating and analyzing arrays. These functions cover a wide range of topics, including linear algebra, statistical analysis, and data visualization.
What is the workflow of NumPy?
The typical workflow for using NumPy is as follows:
- Import NumPy: The first step is to import the NumPy library into your Python program. This can be done using the following import statement:
import numpy as np
2. Create a NumPy array: Once NumPy is imported, you can create a NumPy array using the np.array() function. This function takes a list or other iterable object as input and creates a NumPy array with the same elements.
array = np.array([1, 2, 3, 4, 5])
3. Manipulate the array: NumPy provides a wide range of functions for manipulating arrays. These functions can be used to perform calculations, filter data, and sort data.
array_sum = np.sum(array)
print(array_sum)
4. Analyze the array: NumPy also provides a wide range of functions for analyzing arrays. These functions can be used to calculate statistics, perform hypothesis tests, and fit models to data.
array_mean = np.mean(array)
print(array_mean)
How NumPy Works & Architecture?
NumPy arrays are stored in contiguous memory blocks, which makes them very fast for calculations. NumPy also uses a variety of optimization techniques, such as vectorization and caching, to further improve performance.
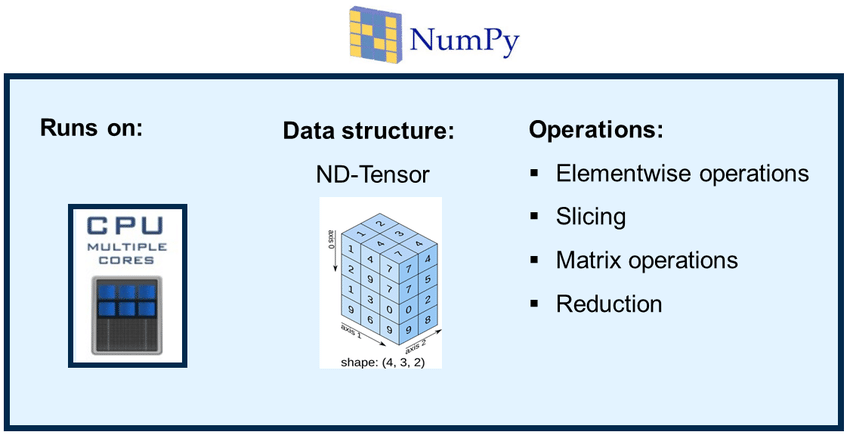
NumPy facilitates an extensive range of mathematical computations on arrays, enhancing Python with robust data structures for efficient calculations involving arrays and matrices. It also provides an extensive library of high-level mathematical functions specifically designed to manipulate these arrays and matrices.
How to Install and Configure NumPy?
Here step-by-step guide for Installing and configuring NumPy:
Step 1: Check Python Installation
Ensure you have Python installed on your system. You can check the Python version using the terminal:
python3 --version
If Python is not installed, download and install the appropriate version for your operating system.
Step 2: Install NumPy
Open a terminal window (Command Prompt or Terminal) and use the pip command to install NumPy:
pip install numpy
This command will download and install the NumPy library for your Python environment.
Step 3: Verify NumPy Installation
To verify that NumPy is installed correctly, start a Python interpreter session using the following command:
python3
Within the Python interpreter, import NumPy and try printing a version information:
import numpy as np
print(np.__version__)
This should print the installed NumPy version, confirming successful installation.
Step 4: Using NumPy
With NumPy installed, you can start using it in your Python scripts. Import NumPy as usual:
import numpy as np
Now you can create and manipulate NumPy arrays, perform calculations, and utilize the vast array of functions and operations provided by NumPy.