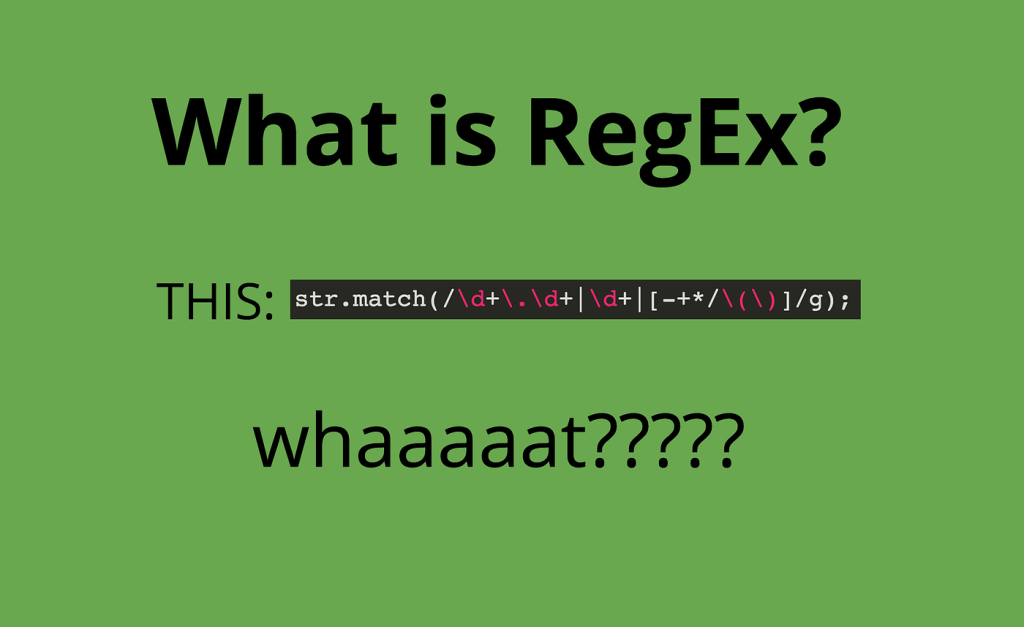
What is Regex?
Regex, short for Regular Expression, is a sequence of characters that forms a search pattern. It is used to find and extract text patterns in strings. Regexes are used in a variety of applications, including text editors, search engines, and programming languages.
Top Use Cases of Regex
The top use cases of regex include:
1. Data validation: regex can be used to verify if data matches a specific format (e.g., checking if an email address is valid).
2. Search and replace: regex can be used to find specific patterns in text and replace them with other patterns.
3. Text parsing: regex can help extract specific information from unstructured text data (e.g., extracting dates or URLs from a document).
4. Text formatting: regex can be used to manipulate text formatting (e.g., adding or removing whitespace).
5. Syntax highlighting: regex is commonly used in code editors to identify and highlight syntax patterns.
Features of Regex
The main features of regex include:
- Patterns: Regexes are made up of patterns that match specific sequences of characters. The patterns can be made up of literal characters, metacharacters, and modifiers.
- Metacharacters: Metacharacters are special characters that have special meaning in regex. For example, the period (.) matches any character, and the asterisk (*) matches zero or more occurrences of the preceding character.
- Modifiers: Modifiers are used to control how the pattern is matched. For example, the
g
modifier matches the pattern globally, which means that it will match the pattern anywhere in the string. - Functions: Regexes can also use functions to perform operations on the patterns. For example, the
sub()
function can be used to replace all occurrences of a pattern with another string.
Workflow of Regex
The workflow of using regex can be summarized in the following steps:
- Define the pattern: Start by defining the pattern you want to match using the regex syntax.
- Compile the regex: Compile the regex pattern into a regex object, which can be used for matching and searching.
- Search for matches: Use the regex object to search for matches within a text document or dataset. You can find all matches or stop after finding the first match.
- Perform operations on matches: Once you have found a match, you can perform various operations on it, such as extracting the matched text, replacing it with a different text, or capturing specific groups within the match.
How Regex Works & Architecture
Regex engines work by parsing the pattern and constructing a finite state machine or a similar data structure. This allows them to efficiently match the pattern against the input text. The exact architecture and implementation details may vary depending on the regex engine or library being used.
When a regex pattern is applied to an input text, the engine starts at the beginning of the text and tries to match the pattern from left to right. It uses various algorithms and optimizations to quickly find matching positions or substrings.
Regex engines may also support additional features, such as backreferences, lookaheads, and lookbehinds, which allow for more advanced pattern matching and manipulation.
How to Install and Configure Regex
Here’s a general guide on how to set up and configure regex in some common programming languages:
1. Python: Python has built-in support for regular expressions through the re
module. You don’t need to install anything extra. Here’s how to use it:
import re
# Your regex code here
2. JavaScript: JavaScript provides regex support as a part of the language. You can use regex literals directly within your code:
var pattern = /your-regex-pattern-here/;
3. Java: Java provides regex support through the java.util.regex
package, which is part of the standard library. You don’t need to install anything extra. Here’s how to use it:
import java.util.regex.*;
// Your regex code here
5. Perl: Perl is known for its strong regex support. You don’t need to install anything extra. You can directly use regex in Perl code:
my $pattern = qr/your-regex-pattern-here/;
6. PHP: PHP provides regex support through functions like preg_match
and preg_replace
. There’s no separate installation for regex; it’s available as part of PHP’s standard library:
$pattern = "/your-regex-pattern-here/";
7. C++ (with Boost Library): If you are using C++, you can use the Boost C++ Libraries to work with regular expressions. You’ll need to install the Boost library if you don’t already have it:
#include <boost/regex.hpp>
// Your regex code here
Step by Step Tutorials for Regex – Hello World Program
To create a “Hello, World!” program using regular expressions in PHP, follow the steps below:
1. Create a new PHP file and open it in a text editor or an integrated development environment (IDE).
2. Insert the following code to define the regular expression pattern and the string to match against:
<?php
$pattern = "/Hello, World!/";
$string = "Hello, World!";
3. Use the preg_match() function to perform a regular expression match on the given string using the provided pattern. This function returns 1 if the pattern matches, or 0 if it does not.
if (preg_match($pattern, $string)) {
echo "Match found!";
} else {
echo "No match found.";
}
?>
4. Save the PHP file with a .php extension, for example, helloworld.php.
5. Open a web browser and run the PHP script by accessing it via a local web server. For example, if you are using XAMPP, place the helloworld.php file inside the htdocs folder and access it at http://localhost/helloworld.php.
6. The output of the script will either display “Match found!” or “No match found.” based on whether the regular expression matches the given string.
By following these steps, you will have created a “Hello, World!” program in PHP that utilizes regular expressions to check if the string matches the defined pattern.