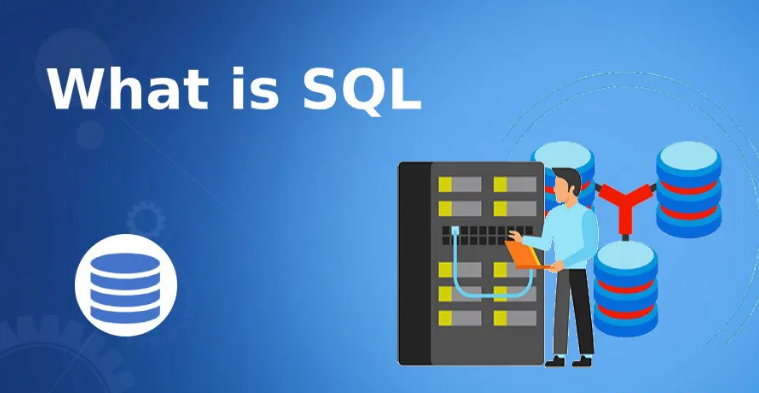
What is SQL?
SQL stands for Structured Query Language, which is used to communicate with the database in the form of queries. SQL is essential for working with relational database management systems (RDBMS) like MySQL, PostgreSQL, Oracle, Microsoft SQL Server, and others.
Database: Collection of data will be store in a database.
What is top use cases of SQL?
The top use cases of SQL include:
- Storing data
- Retrieving data
- Manipulating data
- Creating and managing databases
- Securing data
- Analyzing data
What are feature of SQL?
The features of SQL include:
1. Data Definition Language(DDL):
Data Definition Language (DDL) is a subset of SQL (Structured Query Language) used to define and manage the structure of a database and its objects.
Some of the most common DDL statements include:
- CREATE: This statement is used to create new database objects.
- ALTER: This statement is used to modify existing database objects.
- DROP: This statement is used to delete database objects.
- TRUNCATE: This statement is used to delete all data from a table, but the table structure is retained.
2. Data Manipulation Language(DML): It enables the modification, insertion, update, and deletion of data in databases.
3. Data Querying Language (DQL): DQL is used to retrieve data from tables. There are three command-
- Select : Fetching the column.
- From: From is used to fetching the table name.
- Where: Where is used to provide condition and filtering the data.
4. Data Control Language (DCL): Data Control Language (DCL) in SQL refers to commands like GRANT and REVOKE. It manages user privileges, allowing database administrators to grant or revoke access rights for data and database objects, ensuring security and data integrity by controlling who can perform specific operations within the database.
- GRANT command in SQL allows giving specific permissions to users or roles on database objects for performing certain actions.
- REVOKE command in SQL removes previously granted privileges from users or roles, restricting their access to data and database objects.
5. Transactions Control Language (TCL): TCL is used to control the execution of transactions, which are a series of database operations that are treated as a single unit. There are three command-
- Commit: Save the transaction permanently we can use commit.
- Save Point: Save point is used to save the transaction for temporary.
- Roll back: Roll back is used to delete all the transaction till commit.
What is the workflow of SQL?
The workflow of SQL (Structured Query Language) typically involves the following steps:
1. Gathering requirements: The first step is understanding the specific information you need from a database and gathering the requirements for your query.
2. Designing the database schema: Based on the requirements, you design the structure of the database, including tables, relationships, and constraints.
3. Creating the database: Once the schema is finalized, you create the actual database using a DBMS (Database Management System) such as MySQL, Oracle, or SQL Server.
4. Writing SQL queries: This is where you write SQL statements to retrieve, manipulate, and manage data within the database. There are different types of SQL queries, such as SELECT (retrieve data), INSERT (insert new records), UPDATE (modify existing records), and DELETE (remove records).
5. Executing the queries: You run the SQL queries using a database client or a programming language that supports database connectivity (e.g., Python, Java). The DBMS processes the queries and returns the results.
6. Analyzing and optimizing: After executing queries, you analyze the results and evaluate their performance. You can optimize the queries by indexing, rewriting, or restructuring them to improve efficiency.
7. Testing and debugging: It is crucial to test your SQL queries extensively to ensure they produce the expected results. Debugging is performed to identify and fix any issues or errors encountered during query execution.
8. Deploying and maintaining: Once the queries are thoroughly tested, you can incorporate them into your application, website, or any project that needs to interact with the database. Regular maintenance involves monitoring performance, backing up data, and making necessary adjustments or updates to the queries.
How SQL Works & Architecture?
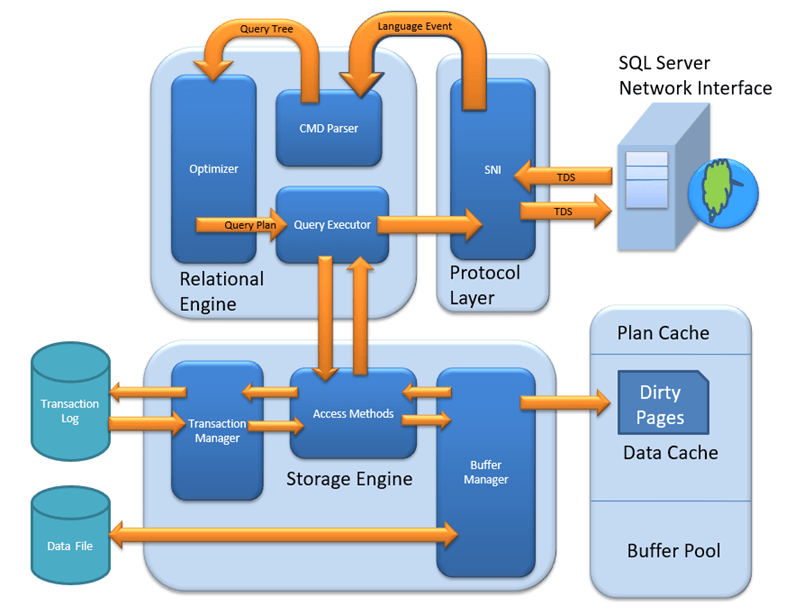
SQL, or Structured Query Language, is a programming language used to manage data in relational database management systems (RDBMS). SQL statements are used to perform tasks such as creating, updating, and querying data in a database.
SQL works by sending commands to the database server. The database server then executes the commands and returns the results to the client application. The client application can then display the results to the user or use them to perform other tasks.
The architecture of a SQL database system is typically divided into three layers:
- The client layer is the layer that interacts with the user. This layer can be a web application, a desktop application, or a command-line interface.
- The middle layer is the database server. This layer is responsible for executing SQL commands and returning the results to the client layer.
- The storage layer is where the data is actually stored. This layer can be a file system, a network storage device, or a cloud storage service.
How to Install and Configure SQL ?
here are the steps on how to install and configure SQL:
Step 1: Download MySQL
- Go to the MySQL website (https://www.mysql.com/).
- Navigate to the Downloads section.
- Choose the appropriate version for your operating system (Windows, macOS, Linux).
Step 2: Install MySQL
Windows:
- Run the MySQL Installer executable you downloaded.
- Choose the “Developer Default” installation type, which includes MySQL Server and various development tools.
- Follow the installation wizard, selecting appropriate options as needed.
- During the installation, you will be asked to set a root password for the MySQL server. Remember this password as you’ll need it later to access MySQL.
macOS:
- Open the DMG package you downloaded.
- Drag the MySQL icon to the Applications folder.
- Open Terminal (found in Applications > Utilities).
- Navigate to the MySQL bin directory using the command
cd /usr/local/mysql/bin/
5. Initialize MySQL with the command:
sudo ./mysql_install_db --user=mysql
6. Start the MySQL server with the command:
sudo ./mysqld_safe --user=mysql &
Linux (Ubuntu as an example):
- Open a terminal.
- Update the package index using:
sudo apt update
3. Install MySQL server using:
sudo apt install mysql-server
4. During the installation, you’ll be prompted to set the root password.
Step 3: Start MySQL Service
On Windows, the MySQL service should start automatically after installation. On macOS and Linux, you may need to start the service manually. For macOS, you can use the command mentioned in Step 2. For Linux, you can start the service using:
sudo service mysql start
Step 4: Access MySQL Command-Line Interface (CLI)
- Open a terminal or command prompt.
- Type the following command and enter the root password you set during installation:
mysql -u root -p
Step 5: Create a New User (Optional)
It’s generally a good practice to create a dedicated user for your applications rather than using the root user for everything. You can create a new user and grant necessary privileges. For example:
CREATE USER 'myuser'@'localhost' IDENTIFIED BY 'mypassword';
GRANT ALL PRIVILEGES ON *.* TO 'myuser'@'localhost' WITH GRANT OPTION;
FLUSH PRIVILEGES;
Step 6: Install GUI Tools (Optional)
While the command-line interface is powerful, you may prefer a graphical user interface (GUI) to interact with the database visually. There are various third-party tools available like MySQL Workbench, phpMyAdmin, etc., depending on your needs.
Fundamental Tutorials of SQL ?
Sure! Here is a step-by-step tutorial for creating your first table in SQL:
- First, make sure you have a database management system (DBMS) installed on your computer. Popular options include MySQL, PostgreSQL, and SQLite.
- Open your preferred DBMS and connect to a database. If you haven’t created a database yet, you can typically use the following command to create one:
CREATE DATABASE database_name;
3. Once you have connected to the desired database, you can create a table using the CREATE TABLE
statement. The basic syntax is as follows:
CREATE TABLE table_name (
column1 datatype1,
column2 datatype2,
column3 datatype3,
...
);
Replace table_name
with the desired name for your table. Inside the parentheses, define the columns of your table along with their data types. For example, if you want to create a table to store information about employees, you could use the following command:
CREATE TABLE employees (
id INT,
name VARCHAR(50),
age INT,
position VARCHAR(50)
);
This creates a table named “employees” with four columns: id
, name
, age
, and position
.
- Once you have defined the table structure, you can insert data into the table using the
INSERT INTO
statement. Here’s an example:
INSERT INTO employees (id, name, age, position)
VALUES (1, 'John Doe', 30, 'Manager');
This inserts a new row into the “employees” table with the specified values for each column.
- You can then perform various operations on the table, such as querying data, updating records, or deleting rows. Here’s an example of selecting all records from the “employees” table:
SELECT * FROM employees;
This retrieves all rows from the table.
That’s it! You’ve created your first table in SQL and performed some basic operations. Feel free to explore more SQL concepts and commands to expand your understanding.
[…] What is SQL and How SQL Works & Architecture? […]